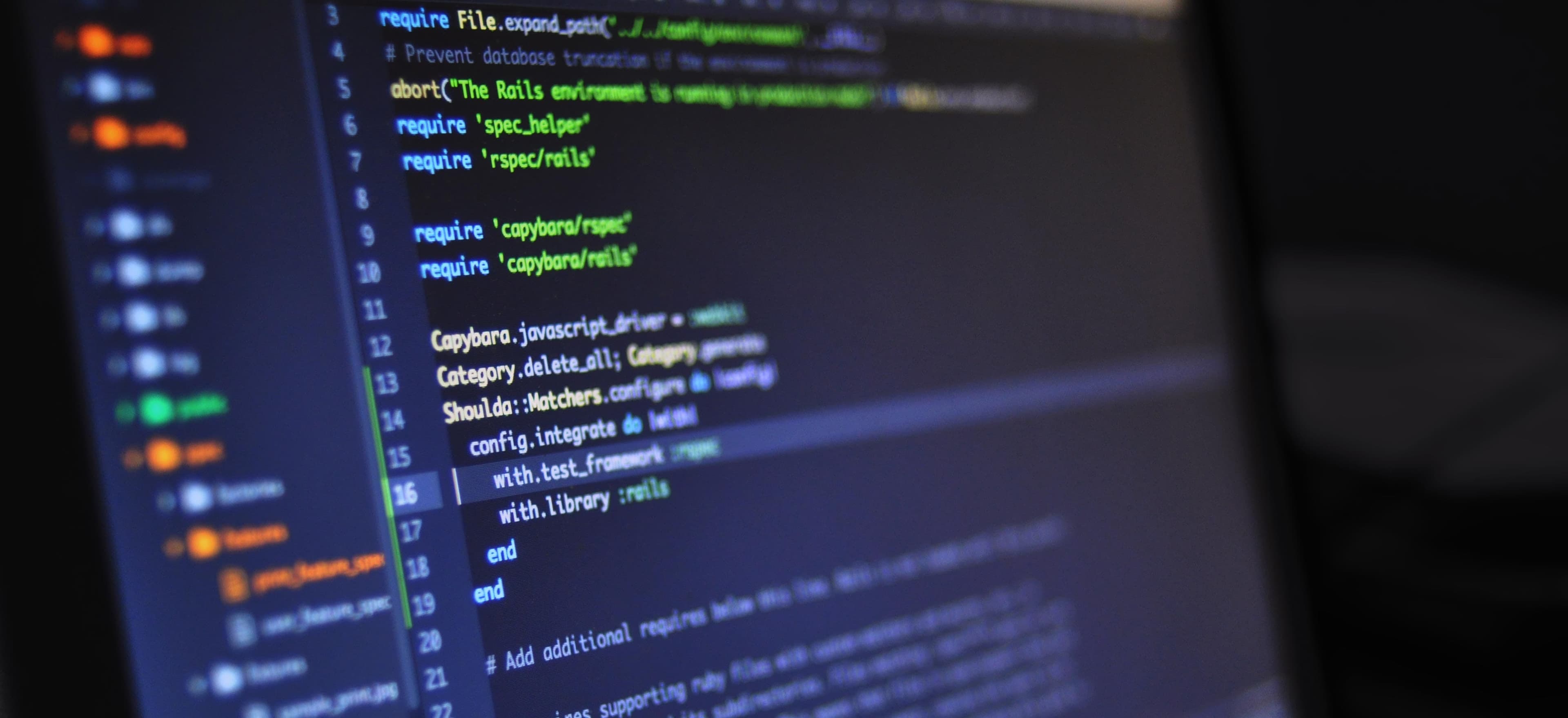
- Published on
Effortless Java Layouts: Centering a Div Made Easy
When designing user interfaces with Java, particularly in web applications, centering elements can often be a challenge. As front-end development practices evolve, the need for responsive and user-friendly designs becomes paramount. Today, we're diving into several techniques for effortlessly centering a div within another div, ensuring that your layout is as polished as it is functional.
Understanding the Basics of Div Centering
Before delving into specific methods, it's essential to understand what we mean by centering a div. In web design, centering typically refers to positioning a child element (the inner div) in such a way that it is equally distant from the sides of its parent element (the outer div).
Why Centering Matters
Centering elements not only enhances visual appeal but also improves user experience. A well-centered layout guides users naturally, allowing for straightforward navigation and interaction. With Java frameworks, achieving this goal can seem daunting; however, it becomes second nature with the right techniques.
1. CSS Flexbox
One of the most popular methods for centering divs in modern web design is CSS Flexbox. This approach is flexible (pun intended) and adaptive, making it suitable for responsive design. Here’s how we can achieve centering using Flexbox:
Code Snippet
.parent {
display: flex;
justify-content: center; /* Aligns children horizontally */
align-items: center; /* Aligns children vertically */
height: 100vh; /* Full viewport height */
background-color: #f0f0f0; /* Light background for visibility */
}
.child {
width: 50%; /* Arbitrary width */
height: 50%; /* Arbitrary height */
background-color: #007bff; /* Color to distinguish the div */
}
Explanation
display: flex;
: This property makes the parent a Flexbox container, enabling the use of Flexbox properties for its child elements.justify-content: center;
: Centers the child div horizontally within the parent.align-items: center;
: Centers the child div vertically within the parent.height: 100vh;
: Sets the parent's height to the full viewport height, offering a stable point of reference for centering.
Flexbox is widely supported and can solve many layout challenges beyond just centering.
2. CSS Grid
If you require more complex layouts or want to define specific areas within a parent div, CSS Grid is an excellent alternative. Grid allows for both two-dimensional layouts (rows and columns) and easily centered divs.
Code Snippet
.parent {
display: grid;
place-items: center; /* Centers child both horizontally and vertically */
height: 100vh;
background-color: #f0f0f0;
}
.child {
width: 50%;
height: 50%;
background-color: #28a745; /* Different color for clarity */
}
Explanation
display: grid;
: This property turns the parent into a grid container.place-items: center;
: This shorthand property centers the child element both horizontally and vertically within the grid container.
CSS Grid is particularly beneficial for intricate layouts where responsiveness is key.
3. Absolute Positioning with Margins
In certain scenarios, you might find using absolute positioning combined with margins to be effective. This technique can help center a child div within a parent, but keep in mind that it requires you to manage positioning carefully.
Code Snippet
.parent {
position: relative; /* Positioning context for absolute children */
height: 100vh;
background-color: #f0f0f0;
}
.child {
position: absolute; /* Positioning relative to the nearest positioned ancestor */
top: 50%; /* Move the child down by 50% of the parent's height */
left: 50%; /* Move the child right by 50% of the parent's width */
transform: translate(-50%, -50%); /* Shifts the child back by its own dimensions */
width: 50%;
height: 50%;
background-color: #dc3545; /* Distinguishable color */
}
Explanation
position: relative;
: This makes the parent a positioning context for its absolute children.top: 50%; left: 50%;
: Positions the top-left corner of the child at the center of the parent.transform: translate(-50%, -50%);
: Shifts the child div back to its center, effectively aligning it.
This method is less flexible with resizing but can be useful in specific designs.
Closing the Chapter: The Art of Centering
Mastering the art of centering divs involves understanding both the technical aspects and the visual considerations of web design. With tools like Flexbox and CSS Grid at your disposal, you can create clean, effective layouts with minimum fuss.
To deepen your understanding of CSS positioning and centering elements, check out the article titled Mastering the Art of Centering a Div within a Div. This resource provides more in-depth knowledge and essential tips to enhance your web development skills.
Additional Resources
For further exploration, consider reviewing:
By employing these methods, you can create visually appealing and user-friendly designs that stand the test of time. Happy coding!