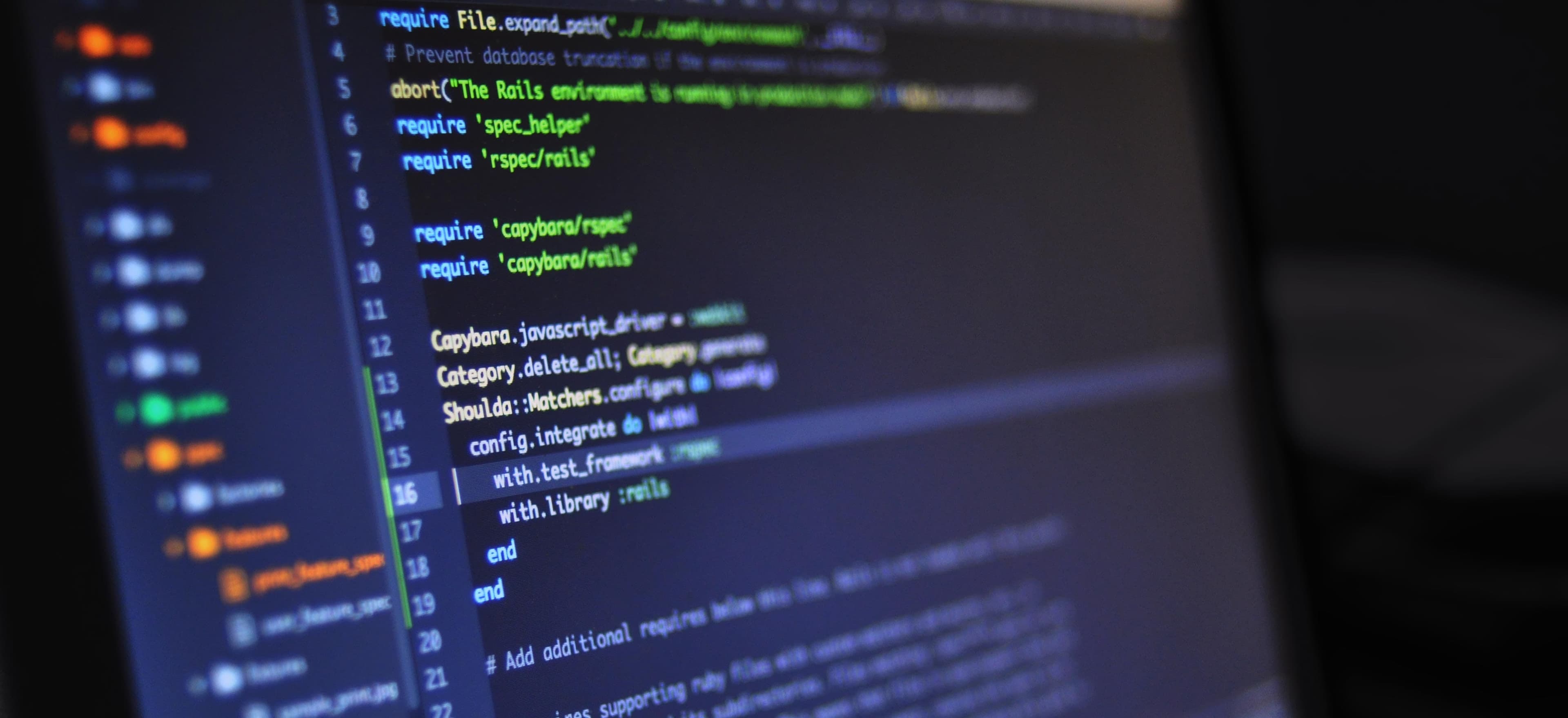
- Published on
Java Tips for Centering Components in Swing Applications
When developing desktop applications using Java's Swing framework, one of the more frequent design challenges is achieving visually appealing layouts. Centering components correctly is crucial, especially for making user interfaces (UIs) intuitive and attractive. Whether you're creating buttons, text fields, or panels, getting everything to fit neatly can be a common source of frustration. In this article, we'll discuss effective strategies for centering components in Swing applications—combining clear explanations with actionable code snippets.
Understanding Swing Layout Managers
Java Swing provides various layout managers to help developers organize components inside containers. Here are some of the most commonly used layout managers:
-
FlowLayout: Arranges components in a left-to-right flow, similar to text.
-
BorderLayout: Divides the container into five regions: North, South, East, West, and Center.
-
GridLayout: Places components in a grid format, defined by rows and columns.
-
BoxLayout: Stacks components either vertically or horizontally.
-
GridBagLayout: A complex and flexible layout manager that allows components to span multiple rows and columns, enabling precise control over component sizes and placement.
For centering components, the FlowLayout
and BorderLayout
are particularly useful.
Centering with FlowLayout
FlowLayout is one of the simplest layout managers in Swing. By default, it aligns components to the left, but you can easily change it to center components.
Example Code Snippet:
import javax.swing.*;
import java.awt.*;
public class CenterFlowLayout {
public static void main(String[] args) {
JFrame frame = new JFrame("Centering Components Example");
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
JPanel panel = new JPanel(new FlowLayout(FlowLayout.CENTER));
panel.add(new JButton("Button 1"));
panel.add(new JButton("Button 2"));
panel.add(new JButton("Button 3"));
frame.add(panel);
frame.setSize(400, 300);
frame.setVisible(true);
}
}
Explanation:
In this example:
- We create a
JFrame
and set its default close operation. - A
JPanel
is added with aFlowLayout
initialized toFlowLayout.CENTER
, ensuring that any components added to this panel will be centered. - Three buttons are added to showcase the effect of the centering.
This method is straightforward and works well for simple layouts with a few components.
Centering with BorderLayout
BorderLayout allows for a more structured approach by defining regions. You can place components in specific areas, and the center region will expand to fill available space.
Example Code Snippet:
import javax.swing.*;
import java.awt.*;
public class CenterBorderLayout {
public static void main(String[] args) {
JFrame frame = new JFrame("Centering Components Example");
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
JPanel panel = new JPanel(new BorderLayout());
JButton centerButton = new JButton("Center Button");
panel.add(centerButton, BorderLayout.CENTER);
frame.add(panel);
frame.setSize(400, 300);
frame.setVisible(true);
}
}
Explanation:
In this snippet:
- A
BorderLayout
is specified for the panel. - The button is added to the center region, ensuring that it will always stay in the middle of the panel.
Using BorderLayout.CENTER
is ideal for scenarios where you want one central component to occupy most of the space, effectively centering it.
Centering with GridBagLayout
GridBagLayout can be more complicated but offers the most control. Using it to center a component can yield more complex layouts.
Example Code Snippet:
import javax.swing.*;
import java.awt.*;
public class CenterGridBagLayout {
public static void main(String[] args) {
JFrame frame = new JFrame("Centering Components Example");
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
JPanel panel = new JPanel(new GridBagLayout());
GridBagConstraints gbc = new GridBagConstraints();
gbc.gridx = 0;
gbc.gridy = 0;
gbc.anchor = GridBagConstraints.CENTER; // Center aligns
panel.add(new JButton("Centered Button"), gbc);
frame.add(panel);
frame.setSize(400, 300);
frame.setVisible(true);
}
}
Explanation:
Here we use GridBagLayout
:
- The
GridBagConstraints
class is utilized to define how components behave within the grid. - We set
anchor
toGridBagConstraints.CENTER
, which ensures the button is centered within its grid cell.
This flexibility is useful when designing more complex interfaces.
Centering Text with JLabel
Sometimes, you may need to center text within labeled components. Swing's JLabel
can easily be centered using SwingConstants
.
Example Code Snippet:
import javax.swing.*;
public class CenterJLabel {
public static void main(String[] args) {
JFrame frame = new JFrame("Centering JLabel Example");
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
JLabel label = new JLabel("Centered Text", SwingConstants.CENTER);
JPanel panel = new JPanel();
panel.add(label);
frame.add(panel);
frame.setSize(400, 300);
frame.setVisible(true);
}
}
Explanation:
In this example:
- We specify
SwingConstants.CENTER
when creating theJLabel
, which centers the text. - A simple panel is used to hold the label; however, for more extensive applications, you might want to consider the layout of the enclosing panel.
Important Layout Tips
-
Always Consider Resizing: Swing components are highly responsive. Make sure the layout behaves as expected when the window resizes.
-
Combine Layout Managers: Don’t hesitate to nest layout managers. Using a
BorderLayout
with panels that useFlowLayout
inside can create interesting and functional UIs. -
Test on Different Screen Sizes: It's also wise to test your application on different screen sizes to ensure it remains visually appealing across devices.
Additional Resources
For further insights into design challenges specific to CSS layouts, consider reviewing Mastering the Art of Centering a Div within a Div. Although it targets web development, the principles of alignment and layout are universal.
Closing Remarks
Centering components in Swing applications doesn't have to be complicated. By understanding the right layout managers and utilizing them effectively, you can create polished UIs with ease. Whether using FlowLayout
, BorderLayout
, or even GridBagLayout
, each provides different advantages to suit your particular needs.
By following the tips in this article, you'll enhance the usability and aesthetics of your applications, leading to a more pleasant experience for the end-user. Happy coding!
Checkout our other articles