Simplifying Java Header Designs: Icons Without Negative Margins
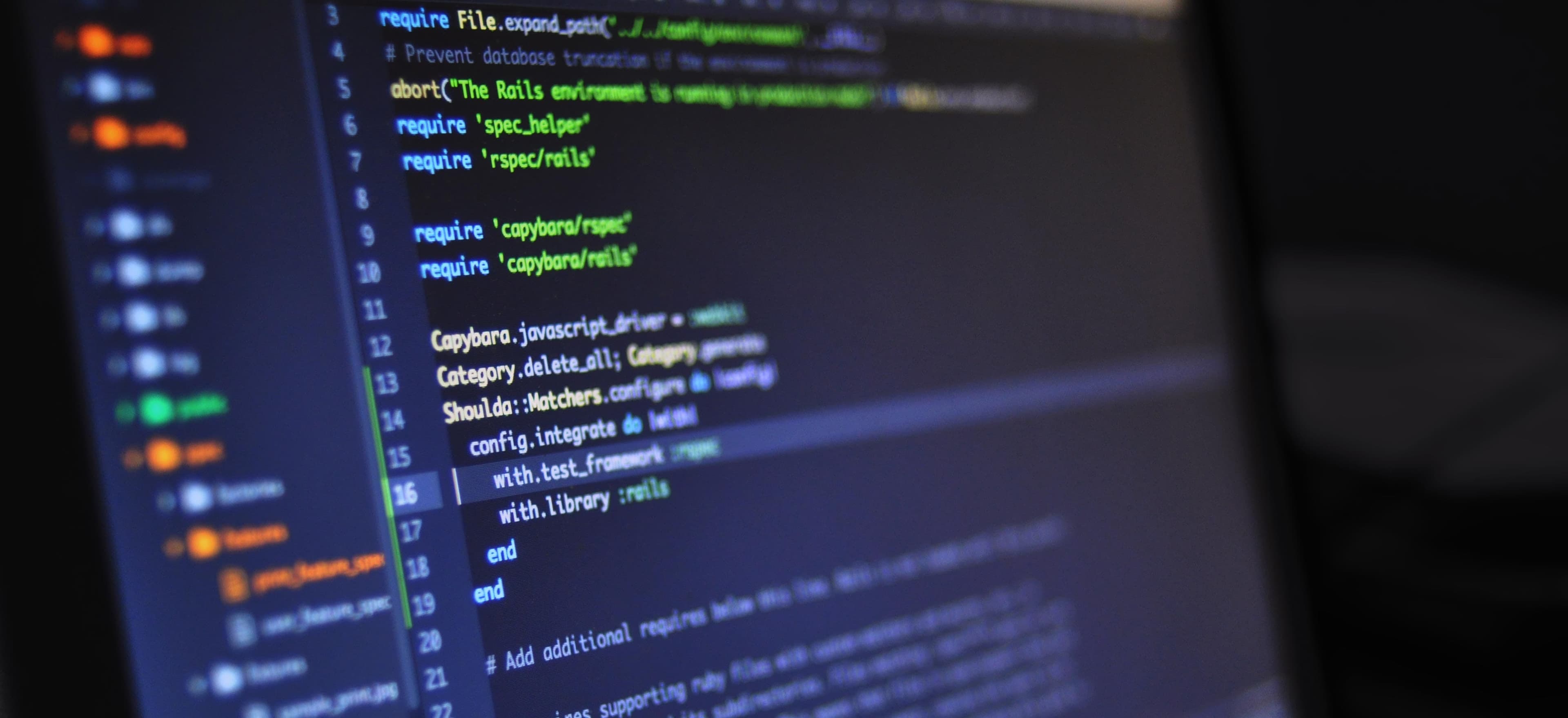
- Published on
Simplifying Java Header Designs: Icons Without Negative Margins
In web development, particularly when designing headers, finding the right alignment for elements can often become a frustrating endeavor. Java developers, especially those leveraging frameworks like JavaFX or Swing, often face the challenge of icon placement within headers. Negative margins have traditionally been the go-to solution for achieving the desired aesthetic. However, this method can lead to unexpected layout issues and complicate responsive designs. This blog post explores how to implement effective icon placement in headers without relying on negative margins, following concepts discussed in Effortless Icon Placement in Headers: No Negative Margins!.
Understanding the Need for Icon Placement
When creating user interfaces, particularly in applications that utilize Java for their graphical user interface (GUI), the placement of icons can significantly affect the overall user experience. Icons serve not just as decorative elements but also as visual cues that enhance usability and accessibility.
Achieving a clean design that works across different devices while avoiding negative margins is crucial. This leads us to explore several layout strategies that can be used in Java GUI frameworks.
JavaFX Layouts for Headers
1. HBox and VBox Layouts
JavaFX provides various layouts, two of the most essential being HBox
and VBox
. These layouts enable developers to stack nodes in horizontal and vertical orientations, respectively. By using these layouts effectively, you can position icons, titles, and menus without the need for negative margins.
Example Code: HBox Layout
import javafx.application.Application;
import javafx.scene.Scene;
import javafx.scene.control.Button;
import javafx.scene.control.Label;
import javafx.scene.image.Image;
import javafx.scene.image.ImageView;
import javafx.scene.layout.HBox;
import javafx.stage.Stage;
public class HeaderDesignExample extends Application {
@Override
public void start(Stage primaryStage) {
// Create icon and text elements
ImageView icon = new ImageView(new Image("icon.png"));
Label title = new Label("Application Title");
Button menuButton = new Button("Menu");
// Create HBox layout for the header
HBox header = new HBox(10); // Spacing of 10px between nodes
header.getChildren().addAll(icon, title, menuButton);
Scene scene = new Scene(header, 300, 100);
primaryStage.setScene(scene);
primaryStage.setTitle("JavaFX Header Example");
primaryStage.show();
}
public static void main(String[] args) {
launch(args);
}
}
Why Use HBox?
The HBox
layout simplifies the structure of your interface. By using a reasonable amount of spacing (10
pixels in this case), you can effectively separate elements without diving into the complexities of margins. This ensures that your design remains intact across various screen sizes and resolutions.
2. AnchorPane for Precise Control
For situations where you need more control over the positioning of components, AnchorPane
can be beneficial. This layout allows you to anchor nodes to the edges of the pane, giving you the flexibility to manage spacing effectively.
Example Code: AnchorPane Layout
import javafx.application.Application;
import javafx.scene.Scene;
import javafx.scene.control.Button;
import javafx.scene.control.Label;
import javafx.scene.image.Image;
import javafx.scene.image.ImageView;
import javafx.scene.layout.AnchorPane;
import javafx.stage.Stage;
public class AnchorPaneHeaderExample extends Application {
@Override
public void start(Stage primaryStage) {
ImageView icon = new ImageView(new Image("icon.png"));
Label title = new Label("Application Title");
Button menuButton = new Button("Menu");
AnchorPane header = new AnchorPane();
// Set positions for nodes
AnchorPane.setTopAnchor(icon, 10.0);
AnchorPane.setLeftAnchor(icon, 10.0);
AnchorPane.setTopAnchor(title, 10.0);
AnchorPane.setLeftAnchor(title, 50.0);
AnchorPane.setTopAnchor(menuButton, 10.0);
AnchorPane.setRightAnchor(menuButton, 10.0);
// Add nodes to AnchorPane
header.getChildren().addAll(icon, title, menuButton);
Scene scene = new Scene(header, 400, 100);
primaryStage.setScene(scene);
primaryStage.setTitle("JavaFX AnchorPane Example");
primaryStage.show();
}
public static void main(String[] args) {
launch(args);
}
}
Why Use AnchorPane?
AnchorPane
gives you the flexibility to place elements with precision. The ability to define exact pixel distances from its edges ensures that your header layout will not only look good but will also be adaptive as the window resizes. You eliminate the guesswork of negative margins, making your design scalable and maintainable.
Swing Layouts for Headers
For developers using Swing, similar logic applies with the use of layout managers like FlowLayout
, BorderLayout
, or GridBagLayout
.
Example Code: FlowLayout
import javax.swing.*;
import java.awt.*;
public class SwingHeaderExample {
public static void main(String[] args) {
JFrame frame = new JFrame("Swing Header Example");
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
frame.setSize(400, 100);
// Use FlowLayout for header
JPanel header = new JPanel(new FlowLayout(FlowLayout.LEFT, 10, 10));
JLabel title = new JLabel("Application Title");
ImageIcon icon = new ImageIcon("icon.png");
JLabel iconLabel = new JLabel(icon);
JButton menuButton = new JButton("Menu");
// Add components to panel
header.add(iconLabel);
header.add(title);
header.add(menuButton);
frame.add(header);
frame.setVisible(true);
}
}
Why Use FlowLayout?
The FlowLayout
manager will keep all components arranged in a line. Specifying spacing as 10
pixels ensures your header looks organized without messy negative margins. It's simple, intuitive, and works well with automatic resizing.
Best Practices for Icon Placement
- Simple Spacing: Ensure you use consistent spacing between icons and text. This prevents clutter and enhances readability.
- Responsive Design: Always design with different screen sizes in mind. Avoid hard-coded positions; instead, rely on layout managers that adapt.
- Maintain Uniform Sizes: Ensure all icons are of uniform size, which helps in creating a harmonious design.
- Accessibility: Use tooltips and aria-labels to improve accessibility for users dependent on screen readers.
A Final Look
Eliminating the dependency on negative margins while placing icons in headers significantly enhances the maintainability and responsiveness of your Java applications. By utilizing layout managers in JavaFX or Swing, such as HBox
, VBox
, AnchorPane
, and FlowLayout
, you can achieve polished and professional-looking headers efficiently.
If you’d like to dive deeper into managing layouts or redesigning your UI efficiently, consider reading our other articles or check out the Effortless Icon Placement in Headers: No Negative Margins! for further insights.
With these strategies at your disposal, you can simplify and enhance your Java application's header designs, making them not just aesthetically pleasing but user-friendly as well. Happy coding!