Mastering Java Layouts: Avoiding Negative Margins in UI Design
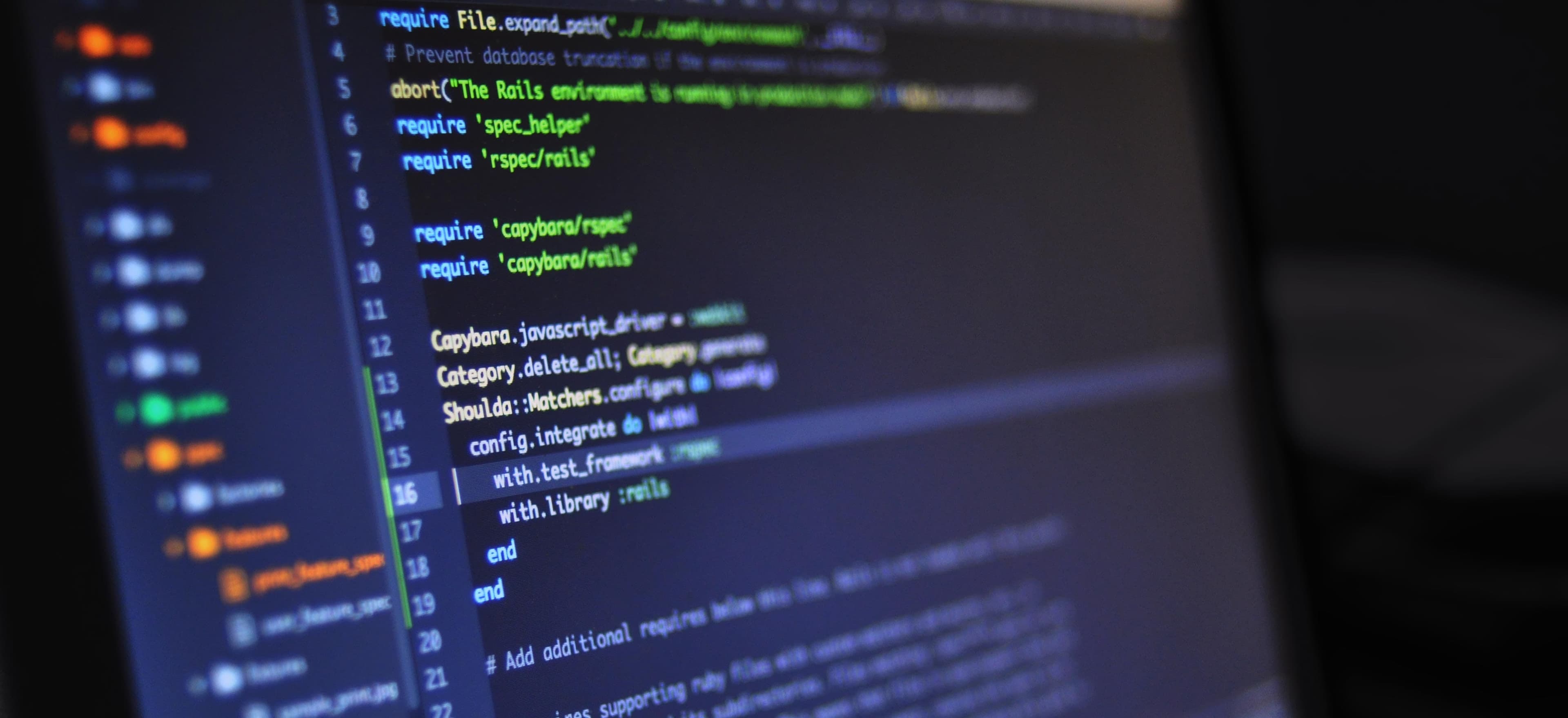
- Published on
Mastering Java Layouts: Avoiding Negative Margins in UI Design
When it comes to UI design in Java, particularly with Swing or JavaFX, one of the common challenges that developers face is managing layouts effectively. Whether you're developing a desktop application or a sophisticated UI, understanding how to use layouts without resorting to negative margins is crucial for creating a clean and responsive design. In this blog post, we'll explore various layout managers in Java and best practices to achieve visually appealing designs without the pitfalls of negative margins.
The Importance of Layout Managers
Java provides several layout managers, each serving a different purpose in organizing components on a container. Layout managers simplify the process of arranging GUI components by automatically handling their size and placement. The most commonly used layout managers include:
- FlowLayout
- BorderLayout
- GridLayout
- BoxLayout
- GridBagLayout
Choosing the correct layout manager can help you avoid negative margins, which can lead to unwanted behavior in your application's user interface. Negative margins are generally discouraged because they can break the layout, making elements overlap or rendering them inaccessible.
FlowLayout: A Simple Start
FlowLayout is one of the simplest layout managers available in Java. It arranges components in a left-to-right flow, wrapping to the next line as needed.
Example Code Snippet
import javax.swing.*;
import java.awt.*;
public class FlowLayoutExample {
public static void main(String[] args) {
JFrame frame = new JFrame("FlowLayout Example");
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
frame.setSize(400, 200);
JPanel panel = new JPanel();
panel.setLayout(new FlowLayout()); // Set FlowLayout
panel.add(new JButton("Button 1"));
panel.add(new JButton("Button 2"));
panel.add(new JButton("Button 3"));
frame.add(panel);
frame.setVisible(true);
}
}
Why Use FlowLayout?
FlowLayout automatically takes care of the sizing and positioning of components without the need for negative margins. This layout behaves predictably as the window resizes, which greatly enhances user experience.
BorderLayout: Divide and Conquer
BorderLayout allows for the arrangement of components in five areas: North, South, East, West, and Center. This layout simplifies the task of organizing primary elements in a user interface.
Example Code Snippet
import javax.swing.*;
import java.awt.*;
public class BorderLayoutExample {
public static void main(String[] args) {
JFrame frame = new JFrame("BorderLayout Example");
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
frame.setSize(400, 200);
JPanel panel = new JPanel(new BorderLayout());
panel.add(new JButton("North"), BorderLayout.NORTH);
panel.add(new JButton("South"), BorderLayout.SOUTH);
panel.add(new JButton("East"), BorderLayout.EAST);
panel.add(new JButton("West"), BorderLayout.WEST);
panel.add(new JButton("Center"), BorderLayout.CENTER);
frame.add(panel);
frame.setVisible(true);
}
}
Why Use BorderLayout?
This layout manager is efficient for applications that have a clear separation between components, minimizing the risk of overcrowding. Unlike manual placement, BorderLayout automatically handles space allocation, reducing the temptation to use negative margins.
GridLayout: A Structured Approach
GridLayout organizes components into a grid format defined by rows and columns, making it ideal for applications that require uniform spacing.
Example Code Snippet
import javax.swing.*;
import java.awt.*;
public class GridLayoutExample {
public static void main(String[] args) {
JFrame frame = new JFrame("GridLayout Example");
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
frame.setSize(400, 200);
JPanel panel = new JPanel(new GridLayout(2, 3)); // 2 rows, 3 columns
panel.add(new JButton("Button 1"));
panel.add(new JButton("Button 2"));
panel.add(new JButton("Button 3"));
panel.add(new JButton("Button 4"));
panel.add(new JButton("Button 5"));
panel.add(new JButton("Button 6"));
frame.add(panel);
frame.setVisible(true);
}
}
Why Use GridLayout?
GridLayout provides a well-defined structure, ensuring that all components occupy equal space. This uniformity helps prevent overflow or misalignments, factors often leading developers to use negative margins for adjustments.
BoxLayout: The Flexible Option
BoxLayout allows for flexible layouts either vertically or horizontally. This manager is perfect for applications requiring dynamic layouts that adapt to the size of components.
Example Code Snippet
import javax.swing.*;
import java.awt.*;
public class BoxLayoutExample {
public static void main(String[] args) {
JFrame frame = new JFrame("BoxLayout Example");
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
frame.setSize(400, 200);
JPanel panel = new JPanel();
panel.setLayout(new BoxLayout(panel, BoxLayout.Y_AXIS)); // Vertical box layout
panel.add(new JButton("Button 1"));
panel.add(Box.createRigidArea(new Dimension(0, 10))); // Adds space between buttons
panel.add(new JButton("Button 2"));
frame.add(panel);
frame.setVisible(true);
}
}
Why Use BoxLayout?
BoxLayout provides great flexibility and is perfect for applications where spacing is a concern. You can create spaces without resorting to negative margins by using Box.createRigidArea
.
GridBagLayout: The Most Powerful (Yet Complex)
GridBagLayout is one of the most sophisticated layouts available in Java, giving developers the ability to create complex arrangements of components.
Example Code Snippet
import javax.swing.*;
import java.awt.*;
public class GridBagLayoutExample {
public static void main(String[] args) {
JFrame frame = new JFrame("GridBagLayout Example");
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
frame.setSize(400, 200);
JPanel panel = new JPanel(new GridBagLayout());
GridBagConstraints gbc = new GridBagConstraints();
gbc.fill = GridBagConstraints.HORIZONTAL;
gbc.gridx = 0; gbc.gridy = 0; // First column, first row
panel.add(new JButton("Button 1"), gbc);
gbc.gridx = 1; gbc.gridy = 0; // Second column, first row
panel.add(new JButton("Button 2"), gbc);
gbc.gridx = 0; gbc.gridy = 1; // First column, second row
gbc.gridwidth = 2; // Spanning both columns
panel.add(new JButton("Button 3"), gbc);
frame.add(panel);
frame.setVisible(true);
}
}
Why Use GridBagLayout?
Though complex, GridBagLayout provides unparalleled control over the positioning and alignment of components. With careful configuration, this layout can eliminate the need for negative margins altogether.
Final Considerations
Developing effective Java UIs requires a solid understanding of layout managers. By utilizing the built-in layout options, you can avoid negative margins commonly associated with poor UI practices. Each layout manager has its advantages and should be selected based on the requirements of your application.
For additional context on UI practices, consider reading Effortless Icon Placement in Headers: No Negative Margins! here.
Remember to test and tweak your layouts as you go, ensuring a polished final product. Mastering Java layouts is not just about choosing a manager; it’s about creating a user-friendly experience that your users will love!
Checkout our other articles