Fixing JSON Type Mismatch Errors in JPA with PostgreSQL
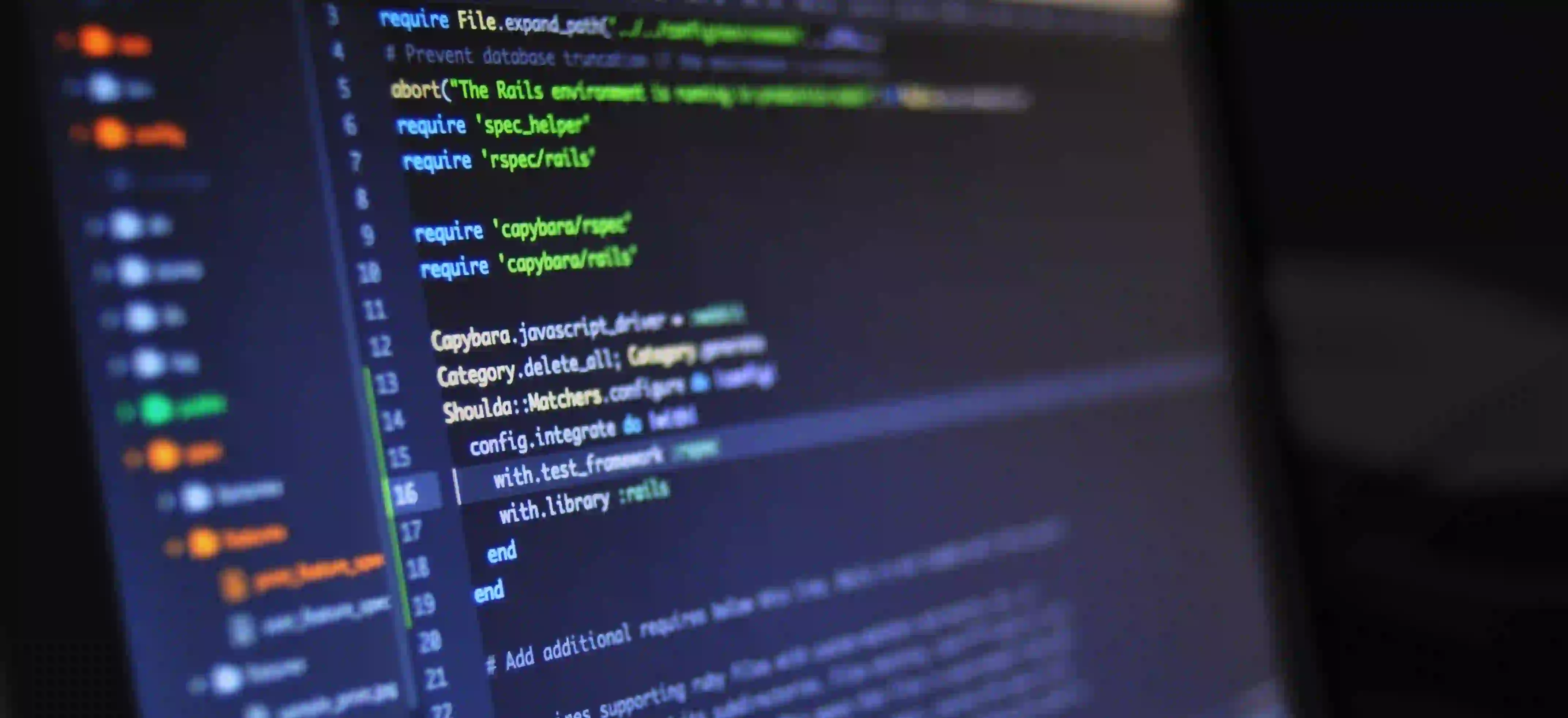
Fixing JSON Type Mismatch Errors in JPA with PostgreSQL
In today’s data-driven world, the ability to handle JSON efficiently in Java applications is paramount, especially when using Java Persistence API (JPA) with PostgreSQL. JPA provides a convenient way to manage relational data, while PostgreSQL's native JSON and JSONB types allow for flexible and efficient data handling. However, developers often encounter JSON type mismatch errors when integrating these two technologies. In this blog post, we will explore the common issues leading to these errors and illustrate effective solutions, along with code examples and explanations to provide clarity.
Understanding JSON Types in PostgreSQL
PostgreSQL supports two JSON data types: JSON
and JSONB
.
- JSON: Stores text in a JSON format.
- JSONB: Stores data in a decomposed binary format, which allows for faster access and manipulation.
Using JSONB
is generally preferred due to its performance advantage concerning query execution and indexing capabilities.
Common Causes of JSON Type Mismatch Errors
When using JPA to map objects to database tables that include JSON types, developers frequently encounter type mismatch errors. Here are some common causes:
-
Incorrect Entity Mapping: When Java objects are mapped to JSON columns improperly, it can lead to serialization/deserialization issues.
-
Data Type Mismatch: If the Java class data type does not correspond to the PostgreSQL JSON/JSONB types, a type mismatch error will occur.
-
Null Value Handling: When inserting or retrieving JSON fields that may be null, improper handling can lead to errors.
-
Driver Configuration Issues: Using outdated PostgreSQL driver versions can also contribute to compatibility issues with JSON types.
Example Scenario
Let’s consider an example with an entity representing a User
, which includes a JSONB field for user preferences.
Entity Class
Here’s how you can define an entity class in JPA representing a user:
import javax.persistence.*;
import com.fasterxml.jackson.databind.JsonNode;
@Entity
@Table(name = "users")
public class User {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long id;
private String name;
@Column(columnDefinition = "jsonb")
private JsonNode preferences;
// Getters and Setters
}
Why Use JsonNode
?
We use JsonNode
from the Jackson library for the preferences
field. This allows for seamless serialization and deserialization of JSON data.
Common JSON Type Mismatch Error
If you attempt to persist a User
object and experience an error like this:
org.hibernate.HibernateException: "could not extract a result set"
This might indicate that the PostgreSQL JSON field is not correctly mapped to the preferences
attribute in the User
entity.
Properly Mapping JSON Fields
To avoid JSON type mismatch errors, ensure the following best practices:
-
Use Correct Data Types: Use
JsonNode
from Jackson or the correspondingMap<String, Object>
if dealing with dynamic JSON structures. -
PostgreSQL Configurations: Ensure the database is set to recognize the
JSONB
type. A common SQL command for creating the table would be:📄snippet.txtCREATE TABLE users ( id SERIAL PRIMARY KEY, name VARCHAR(100), preferences JSONB );
-
JPA Annotations: Use annotations appropriately to define JSON fields.
Example of Persisting a User
Here’s how to persist a user object properly:
public void createUser(EntityManager entityManager) {
User user = new User();
user.setName("John Doe");
ObjectMapper objectMapper = new ObjectMapper();
ObjectNode preferences = objectMapper.createObjectNode();
preferences.put("theme", "dark");
preferences.put("notifications", true);
user.setPreferences(preferences);
entityManager.persist(user);
}
Explanation of the Code
In the createUser
method:
- We create a
User
object and set the name. - An
ObjectMapper
instance is used to construct thepreferences
asObjectNode
, which can be easily converted to JSON. - Finally, we persist the
User
via theEntityManager
.
Handling Null Values
To handle JSON fields that can be null, ensure you account for possible null before persisting or updating:
public void updateUserPreferences(EntityManager entityManager, Long userId, JsonNode newPreferences) {
User user = entityManager.find(User.class, userId);
if (user != null) {
if (newPreferences != null) {
user.setPreferences(newPreferences);
} else {
user.setPreferences(null); // Explicitly setting to null
}
entityManager.merge(user);
}
}
Summary of the Update Logic
- Retrieve the user by ID using
find()
. - Check if the new preferences are null and set appropriately to avoid issues during database operations.
Using PostgreSQL Driver with JPA
Ensure that you are using a compatible version of the PostgreSQL driver. Check your pom.xml
if you're using Maven:
<dependency>
<groupId>org.postgresql</groupId>
<artifactId>postgresql</artifactId>
<version>42.4.0</version>
</dependency>
The Closing Argument
By understanding how to properly map JSON types in JPA with PostgreSQL, developers can avoid common pitfalls associated with JSON type mismatch errors. Leveraging the power of PostgreSQL's JSONB type combined with the serialization capabilities of Jackson in Java leads to better data handling and improved application performance.
For more options on working with JSON in PostgreSQL, you can check the official PostgreSQL JSON documentation.
If you run into further issues or have questions about JPA and PostgreSQL integration, feel free to share them in the comments! Happy coding!