Streamlining Java Regex for Effective HTML Tag Conversion
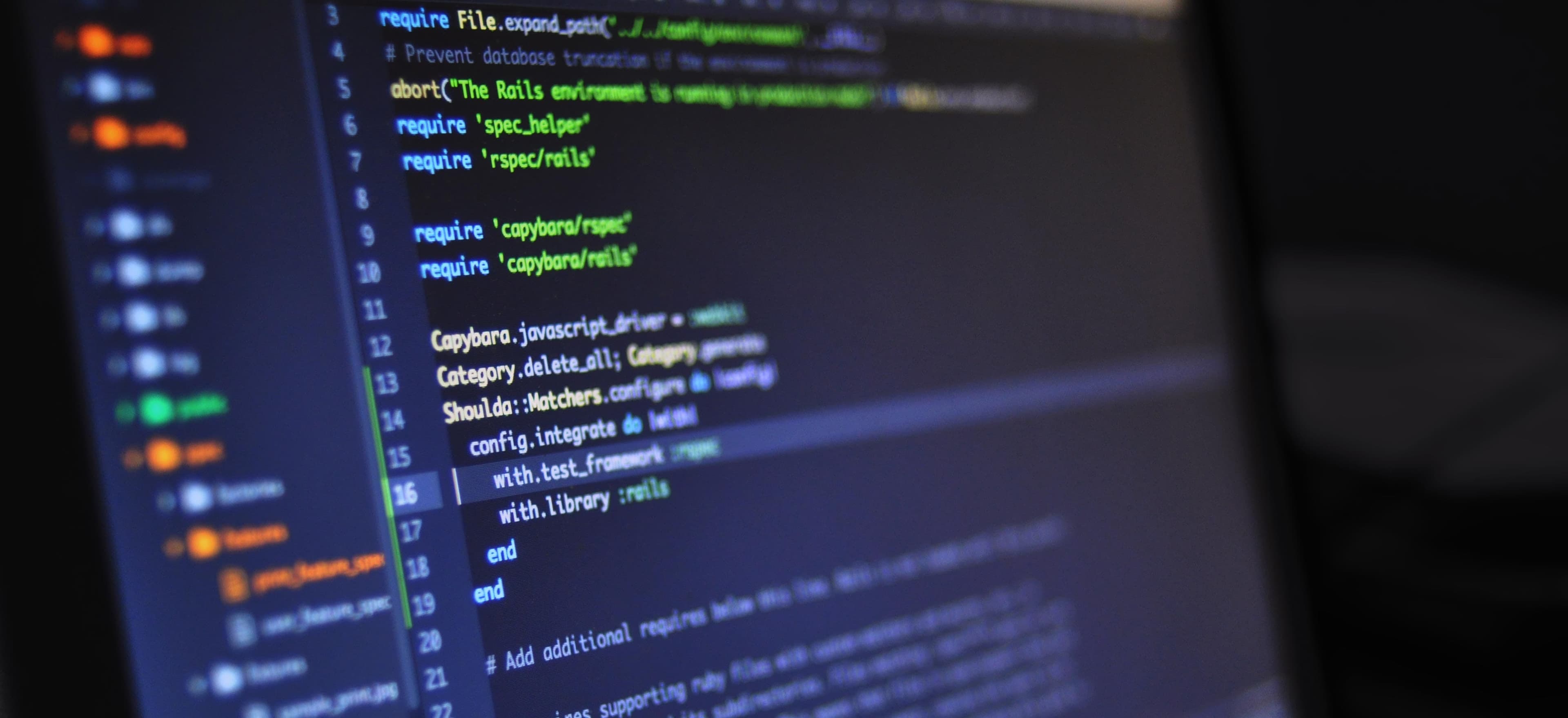
- Published on
Streamlining Java Regex for Effective HTML Tag Conversion
When it comes to processing HTML data, Java developers often find themselves wrestling with regex (regular expressions) to fulfill their needs. Understanding regex is paramount, especially when the task at hand involves converting HTML tags efficiently. In this blog post, we'll dissect the intricacies of Java regex, particularly in light of tag conversion, and explore how we can optimize our approach for better performance. If you’re interested in digging deeper, check out the existing article on "Fixing Regex for Converting HTML Tags Efficiently" at infinitejs.com/posts/fix-regex-convert-html-tags.
Understanding the Basics of Regex in Java
Regex is a powerful tool that enables pattern matching within strings. Java provides a robust API for regex through the java.util.regex
package, which includes classes like Pattern
and Matcher
.
Key Classes:
- Pattern: It represents a compiled representation of a regular expression.
- Matcher: This class performs matching operations on an input string using the pattern.
Before delving into HTML tag conversion, let's look at an example that illustrates the basic regex functionality in Java.
Example: Simple Email Validation
Here is a simple code snippet that uses regex to validate email addresses.
import java.util.regex.Pattern;
import java.util.regex.Matcher;
public class EmailValidator {
public static boolean validateEmail(String email) {
String emailRegex = "^[\\w-\\.]+@([\\w-]+\\.)+[\\w-]{2,4}$";
Pattern pattern = Pattern.compile(emailRegex);
Matcher matcher = pattern.matcher(email);
return matcher.matches();
}
public static void main(String[] args) {
String testEmail = "example@gmail.com";
if(validateEmail(testEmail)) {
System.out.println("Valid email address.");
} else {
System.out.println("Invalid email address.");
}
}
}
Commentary on the Email Validator
In this example, we first compile a regex pattern for validating email addresses. The regex pattern checks for alphanumeric characters, dots, dashes before the "@" symbol and ensures that there is a valid domain name afterwards. The matcher runs the validation on the input email and returns a boolean result.
This snippet serves as a gateway into understanding the potential of regex operations in Java. Now, let's pivot to our primary focus: handling HTML tag conversion.
The Challenge of HTML Tag Conversion
HTML (Hypertext Markup Language) is ubiquitous on the web. When processing HTML, you may need to manipulate tags or convert them to different formats. This often requires regex to search, match, and replace various HTML elements.
However, regex can quickly become complicated and inefficient due to the potential nesting of tags, attributes, or other HTML complexities. The challenge lies in how to streamline our regex patterns for effective HTML tag conversions.
Regex Patterns for HTML Tag Matching
Here’s a foundational regex pattern that can be used to match HTML tags:
String htmlRegex = "<\\s*([a-zA-Z][a-zA-Z0-9]*)\\b[^>]*>(.*?)<\\s*/\\s*\\1\\s*>";
Breakdown of the HTML Regex Pattern
<\\s*
: Matches the opening angle bracket and any whitespace after it.([a-zA-Z][a-zA-Z0-9]*)
: Captures the tag name. This allows only valid HTML tag names.\\b[^>]*
: Matches any attributes within the tag.(.*?)
: Captures everything between the opening and closing tags non-greedily.<\\s*/\\s*\\1\\s*>
: Matches the corresponding closing tag.
Example: Converting HTML Tags to Plain Text
Let’s look at a code snippet that utilizes the above regex for converting HTML tags into plain text by removing them.
public class HtmlTagConverter {
public static String convertHtmlToPlainText(String html) {
String htmlRegex = "<\\s*([a-zA-Z][a-zA-Z0-9]*)\\b[^>]*>(.*?)<\\s*/\\s*\\1\\s*>";
Pattern pattern = Pattern.compile(htmlRegex, Pattern.DOTALL);
Matcher matcher = pattern.matcher(html);
return matcher.replaceAll("$2"); // Replace matched tags with their inner content
}
public static void main(String[] args) {
String htmlString = "<p>This is a <strong>test</strong> paragraph.</p>";
String plainText = convertHtmlToPlainText(htmlString);
System.out.println(plainText); // Output: This is a test paragraph.
}
}
Commentary on HtmlTagConverter
This code snippet defines a method that takes an HTML string and removes any tags, replacing them with only the textual content inside the tags.
Notice how the regex pattern allows for matching pairs of tags. We use Pattern.DOTALL
to allow the dot (.
) to match newline characters, ensuring it can handle multiline HTML correctly.
The line matcher.replaceAll("$2");
captures and replaces matched HTML tags with their inner content. This illustrates clean and effective regex usage.
Streamlining Regex Patterns
While regex is powerful, it can be improved. A few strategies to optimize regex patterns include:
-
Avoid Unnecessary Groups: Use non-capturing groups when you do not need to extract the matched text.
- Change
(<tag>)
to(?:<tag>)
.
- Change
-
Limit Backtracking: Use quantifiers wisely to avoid performance hits from excessive backtracking.
-
Pre-compile Patterns: As shown in previous examples, compile regex patterns before usage to optimize performance.
Additional Tools and Libraries
Apart from using Java's built-in regex tools, consider integrating libraries that simplify HTML parsing. Libraries like Jsoup can help parse HTML and manipulate it without needing to handle string matching manually. This can lead to cleaner, more maintainable code.
Lessons Learned
Regular expressions in Java provide a formidable means to convert HTML tags effectively. However, the complexity of HTML necessitates a prudent approach to regex formulation. Learning to streamline regex patterns - focusing on performance and accuracy - can significantly elevate your development efforts.
If you want a deeper dive into optimizing regex for HTML tag conversion, don’t forget to check out "Fixing Regex for Converting HTML Tags Efficiently" at infinitejs.com/posts/fix-regex-convert-html-tags.
By understanding regex fundamentals, employing best practices, and leveraging libraries like Jsoup, you can tackle HTML parsing tasks more efficiently and maintainably.
Happy coding!
Checkout our other articles