Common Spring Setter Injection Mistakes and How to Fix Them
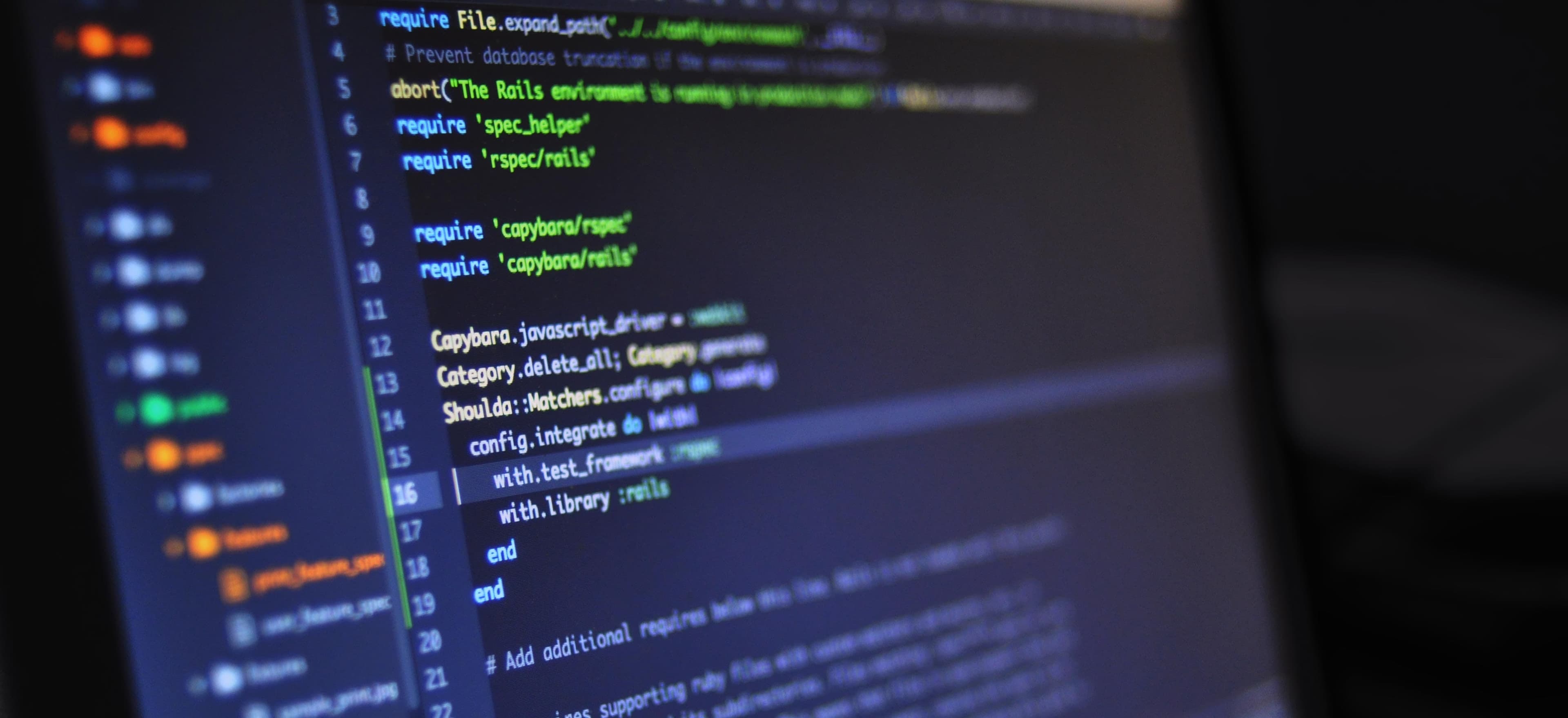
- Published on
Common Spring Setter Injection Mistakes and How to Fix Them
Spring Framework is a powerful tool for developing Java-based applications. One of the core functionalities provided by Spring is Dependency Injection (DI). The setter injection method is one of the most commonly used forms of DI within Spring's context. However, like any pattern or practice, it's not immune to mistakes. In this blog post, we'll explore the common setter injection mistakes in Spring, their implications, and how to effectively mitigate them.
What is Setter Injection?
Before diving into the mistakes, let’s briefly recap what setter injection is. Setter Injection refers to the technique where the Spring container injects dependencies into your beans using setter methods. This allows for more flexible configurations compared to constructor injection. Here’s a simple example:
@Component
public class UserService {
private UserRepository userRepository;
@Autowired
public void setUserRepository(UserRepository userRepository) {
this.userRepository = userRepository;
}
}
In this example, UserService
depends on UserRepository
, and the dependency is injected through the setter method.
Common Mistakes in Setter Injection
1. Forgetting to Annotate the Setter Method
One of the first mistakes developers often make is forgetting to annotate the setter method with @Autowired
. Without this annotation, Spring will not recognize the method for dependency injection.
How to Fix:
Always ensure you annotate your setters with @Autowired
.
@Autowired
public void setUserRepository(UserRepository userRepository) {
this.userRepository = userRepository;
}
2. Using Non-Public Setter Methods
Setter methods should be at least protected
or public
. If a setter is marked as private
, the Spring container won’t be able to access it for dependency injection.
How to Fix:
Make sure your setter methods are public
or protected
.
protected void setUserRepository(UserRepository userRepository) {
this.userRepository = userRepository;
}
3. Setter Methods with Parameters of Wrong Type
Another common mistake is defining setter methods with incorrect parameter types. If the type mismatch occurs, Spring will throw an exception.
How to Fix:
Always verify the parameter type of your setters matches that of the corresponding bean being injected.
public void setUserRepository(UserRepository userRepository) { // Correct type
this.userRepository = userRepository;
}
4. Forgetting to Define the Bean in Spring Context
In Spring, if you want to inject a dependency, that dependency must be defined as a bean in the application context. Forgetting to do so will result in a NoSuchBeanDefinitionException
.
How to Fix:
Ensure that the class you are trying to inject is annotated with @Component
, @Service
, or other relevant annotations to create a bean.
@Repository
public class UserRepository {
// Repository logic here
}
5. Circular Dependency Issues
Setter injection can inadvertently cause circular dependencies. For instance, if two beans rely on each other, and both expect their dependencies to be injected via setters, you may run into a BeanCurrentlyInCreationException
.
How to Fix:
To resolve circular dependencies, consider using lazy initialization. Annotate one of the dependencies with @Lazy
to break the cycle:
@Component
public class FirstService {
private SecondService secondService;
@Autowired
public void setSecondService(@Lazy SecondService secondService) {
this.secondService = secondService;
}
}
6. Failing to Handle Optional Dependencies
Sometimes, not all dependencies are required for the class to function correctly. Failing to handle optional dependencies can lead to runtime issues if the injected dependency is null
.
How to Fix:
To make a dependency optional, use the required
attribute of the @Autowired
annotation:
@Autowired(required = false)
public void setUserRepository(UserRepository userRepository) {
this.userRepository = userRepository;
}
7. Setter Injection vs Constructor Injection Confusion
There is a structural and philosophical difference between setter injection and constructor injection. Some developers struggle to recognize when to use one over the other. Setter injection allows for optional and mutable dependencies, while constructor injection is better for mandatory dependencies.
How to Fix:
Evaluate your use case. If a dependency is required for the class to work correctly, prefer constructor injection. For optional dependencies or settings that can change, setter injection might be suitable.
8. Overusing Setter Injection
Setter injection can lead to poor encapsulation if overused. Allowing dependencies to be modified after the object's creation can make your code less predictable and harder to maintain.
How to Fix:
Limit setter injection to optional dependencies or scenarios where mutability is explicitly needed. As a best practice, aim for immutability whenever possible.
Key Takeaways
Setter injection is a flexible and powerful feature in the Spring Framework. However, it comes with its own set of pitfalls. By being aware of the common mistakes, you can avoid them and ensure your application remains robust and maintainable.
For further reading on dependency injection in Spring, you might want to check out the official Spring Documentation or an insightful discussion on Constructor vs. Setter Injection.
By understanding and fixing these common setter injection mistakes, you'll lay the groundwork for a more effective and error-free development process in your Spring applications. Happy coding!
Checkout our other articles