Overcoming Cache Invalidation Challenges: Strategies for Success
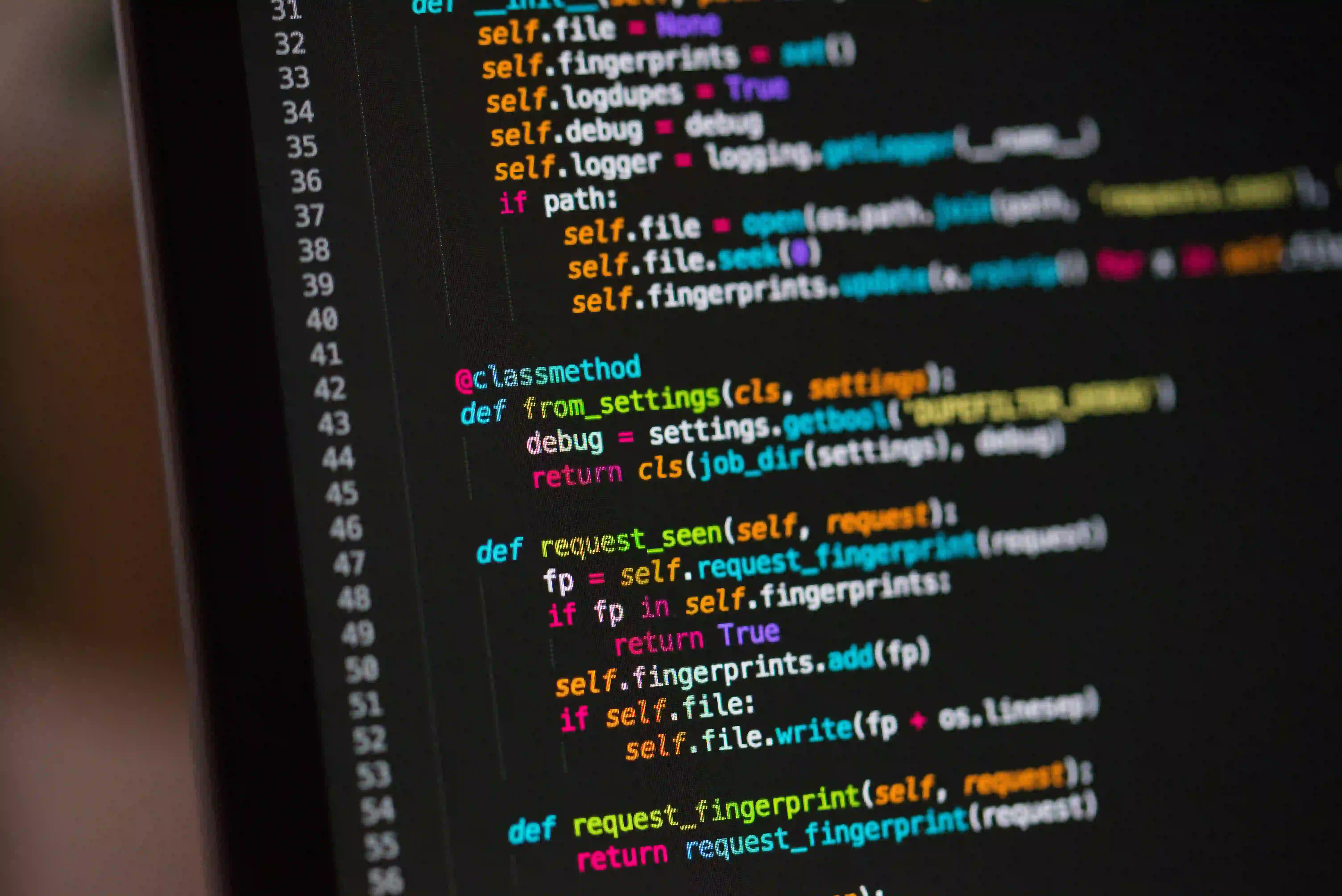
Overcoming Cache Invalidation Challenges: Strategies for Success
Caching is a critical optimization technique in modern software architecture, designed to enhance performance and reduce latency by storing frequently accessed data. However, one of the most persistent challenges developers face is cache invalidation. An effective strategy for managing cache invalidation can make a significant difference in application performance and data accuracy. In this blog post, we will explore the intricacies of cache invalidation and present strategies to overcome these challenges.
Understanding Cache Invalidation
Cache invalidation refers to the process of removing or updating data from the cache that becomes stale due to changes in the underlying data source. If not managed correctly, stale data can lead to inaccurate results and can significantly impact user experience.
Why It Matters
The importance of effective cache invalidation cannot be overstated. Here are a few reasons why:
- Data Freshness: Users expect to see the latest data. Stale data can mislead users, leading to poor decision-making.
- Performance: Properly managing cache can help retain high performance, reducing the need to fetch data from the primary store unnecessarily.
- Resource Management: Efficient cache invalidation can help reduce memory usage and prevent resource leaks.
Cache Invalidation Strategies
1. Time-Based Expiration
One of the simplest strategies for cache invalidation is time-based expiration, where caches are set to expire after a predefined interval. This can be beneficial in scenarios where data changes predictably.
Cache<String, String> cache = CacheBuilder.newBuilder()
.expireAfterWrite(10, TimeUnit.MINUTES)
.build();
Why it’s useful: This approach ensures that even if underlying data changes, the cache will automatically invalidate after a certain period, thus maintaining a reasonable balance between performance and data freshness.
2. Write-Through and Write-Behind Caching
In write-through caching, data is written to the cache and the database simultaneously. In contrast, write-behind caching allows writes to be recorded in the cache first, with the data being asynchronously written to the database later.
public void saveData(String key, String value) {
cache.put(key, value); // Write to cache
database.save(key, value); // Write to database
}
Why it’s useful: Write-through ensures that the cache is always updated in line with the database. Write-behind can improve performance by reducing wait times for the user, but it needs careful design to handle potential failures.
3. Cache Aside Pattern
In the cache aside pattern, the application is responsible for loading data into the cache. When data is requested, the cache is checked first. If the data is absent, it is fetched from the database, added to the cache, and then returned.
public String getData(String key) {
String value = cache.getIfPresent(key);
if (value == null) {
value = database.get(key);
cache.put(key, value);
}
return value;
}
Why it’s useful: This pattern minimizes load on the database and ensures that data is only cached when necessary, maintaining freshness.
4. Event-Driven Invalidation
In larger systems, it is often useful to maintain a real-time view of data across different caches. An event-driven architecture using messaging systems such as Apache Kafka or RabbitMQ can help facilitate this.
// Pseudocode for event listener
public void handleEvent(CacheUpdateEvent event) {
cache.invalidate(event.getKey());
}
Why it’s useful: This ensures that when changes occur, they are propagated to all related caches, maintaining consistency across different services.
5. Client-Side Caching
For front-end applications, caching at the client-side can drastically reduce load times and improve user experience. This can be especially relevant in applications with heavy read operations.
// Example of client-side caching using localStorage in JavaScript
localStorage.setItem(key, JSON.stringify(data));
// To retrieve:
const data = JSON.parse(localStorage.getItem(key));
Why it’s useful: Client-side caching offloads the server and minimizes latency for users by reducing the number of requests sent to the backend.
6. Hybrid Approaches
A combination of the above strategies can often yield the best results. For instance, using an event-driven system alongside time-based expiration can help maintain freshness while preventing overwhelming the database.
Building an Invalidation Strategy: Key Considerations
When designing a cache invalidation strategy, it is crucial to consider several factors:
- Nature of the Data: Is the data static or does it change frequently? Understanding the data behavior can help determine the best caching strategy.
- Performance Requirements: What are the performance benchmarks that your application must meet? This can guide your choice of caching techniques.
- System Architecture: The overall architecture may influence how cache invalidation is approached. For microservices, an event-driven approach may be more suitable.
- Scalability: Will your solution scale well as your application grows? Consider how adding more instances of your service will affect cache management.
Lessons Learned
Overcoming cache invalidation challenges is a multi-faceted endeavor that requires a careful balance of strategies tailored to your specific application needs. Implementing these strategies effectively can improve both performance and data accuracy, which are paramount in building modern applications.
While caching can introduce complexities, understanding the benefits and intricacies of various cache invalidation techniques enables developers to make informed decisions.
For more information on caching strategies and their implementations, consider checking resources such as Caching Strategies in Microservices and Effective Caching Techniques.
By following the best practices discussed in this article, you can build a robust caching system that minimizes invalidation challenges and provides a seamless experience for your users. Happy coding!