Mastering JUnit: How to Write Effective Test Names
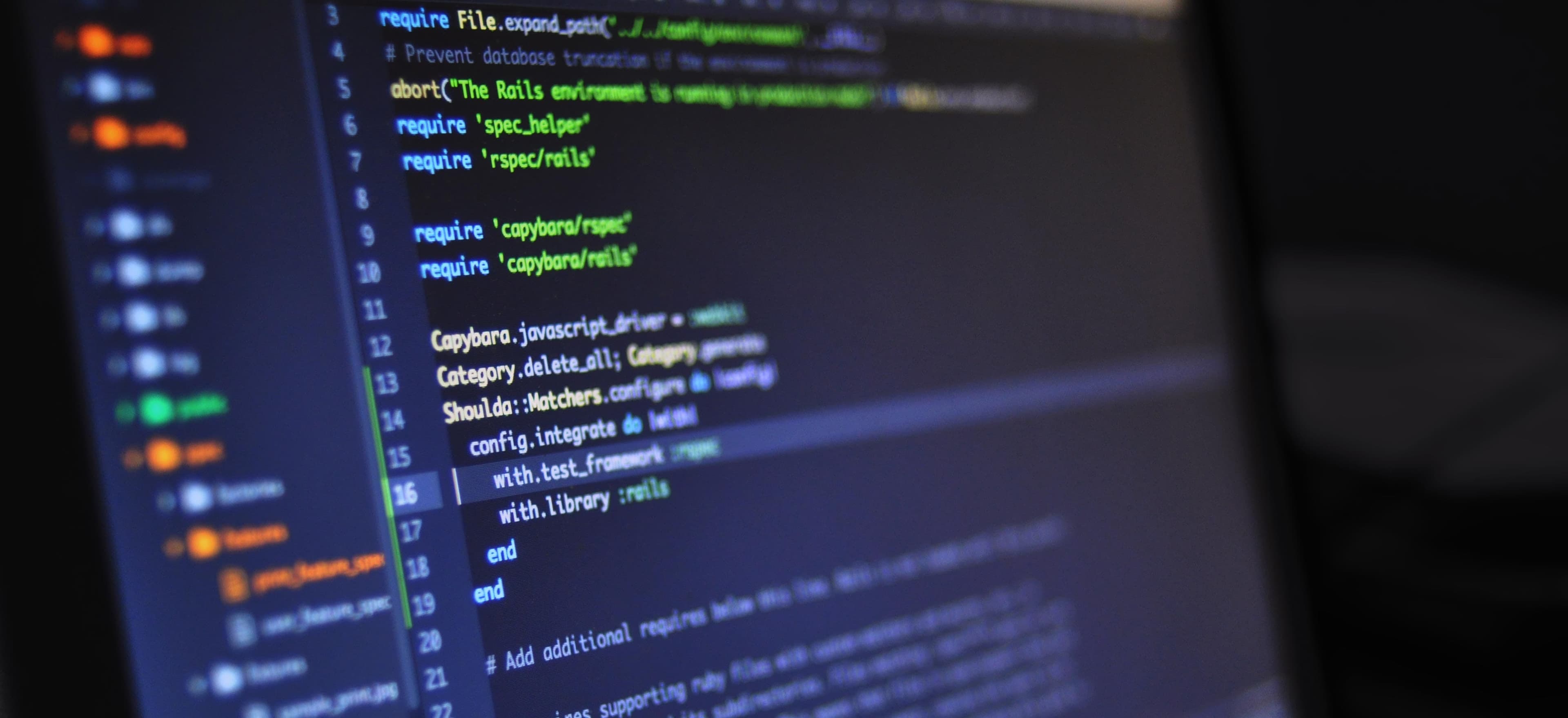
- Published on
Mastering JUnit: How to Write Effective Test Names
Writing effective test names is a crucial aspect of creating reliable and maintainable tests in Java with JUnit. Good test names not only convey the purpose of the test but also help teams understand the codebase better. In this blog post, we’ll explore the best practices for naming your JUnit tests, along with examples and code snippets to illustrate each point.
Why Test Names Matter
When you or someone else revisits a codebase after a while, clear and descriptive test names can significantly reduce the time it takes to understand what each test does. Poorly named tests can lead to confusion, wasted time, and even broken functionality due to misinterpretation.
The Benefits of Good Test Naming
- Clarity: Clearly conveys the intent and behavior being tested.
- Maintainability: Easier to refactor or update over time.
- Documentation: Acts as a form of living documentation for your codebase.
- Ease of Debugging: Simplifies the identification of failing tests.
Naming Conventions for JUnit Tests
1. Use the Given-When-Then Pattern
One effective way to structure your test names is by using the Given-When-Then pattern. This approach gives context to each test case.
- Given: The initial state or context.
- When: The action performed.
- Then: The expected outcome.
Example:
@Test
void givenValidInput_whenCalculateDiscount_thenReturnsCorrectValue() {
double result = DiscountCalculator.calculateDiscount(100.0, 0.20);
assertEquals(80.0, result);
}
In this example, the test name makes it clear that, with valid input, a specific calculation is expected to yield a certain result. This clarifies the purpose of the test at a glance.
2. Be Descriptive but Concise
A good test name should be descriptive enough to understand the test's purpose, yet concise to avoid making it cumbersome. Aim for brevity while retaining meaningful context.
Example:
@Test
void calculateTax_whenIncomeIsHigh_thenReturnsHigherTaxRate() {
double result = TaxCalculator.calculateTax(100000.00);
assertEquals(30000.00, result);
}
While the test name here is descriptive, it could be streamlined further without losing meaning.
3. Use the Test Subject as a Prefix
Another helpful convention is to prefix your test names with the class or method being tested. This helps in quickly identifying which component each test pertains to.
Example:
@Test
void ItemService_addItem_whenItemIsValid_thenReturnsSuccessMessage() {
String result = itemService.addItem(validItem);
assertEquals("Item added successfully", result);
}
Prefixing the test with the class being tested makes it easier to discover where the test is related, especially in larger projects.
4. Incorporate Edge Cases
In scenarios where edge cases are involved, indicate these conditions in the test name. This can improve understanding for anyone reading the tests.
Example:
@Test
void calculateDiscount_whenNoItemsInCart_thenReturnsZeroDiscount() {
double result = DiscountCalculator.calculateDiscount(0, 0.20);
assertEquals(0.0, result);
}
This approach helps clarify that the test specifically checks a unique case, i.e., an empty cart yielding no discount.
Avoiding Common Pitfalls
1. Don’t Use Generic Names
Using overly generic names like test1
or checkFunctionality
should be avoided. These names offer very little context and can lead to confusion about their purpose.
2. Don’t Include Implementation Details
While the intention is to be descriptive, inclusion of specific implementation details can be counterproductive. Keep the focus on what the test is checking rather than how.
3. Disallow Test Method Overloading
JUnit’s behavior can often lead to confusion if you use overloaded method names. Each test case should have a unique name that reflects its specific behavior.
Code Snippets Overview
Let’s combine all these naming techniques into a fuller JUnit test class example.
import static org.junit.jupiter.api.Assertions.assertEquals;
import org.junit.jupiter.api.Test;
class DiscountCalculatorTest {
@Test
void calculateDiscount_whenValidInputs_thenReturnsCorrectDiscount() {
double result = DiscountCalculator.calculateDiscount(1000.0, 0.15);
assertEquals(850.0, result);
}
@Test
void calculateDiscount_whenNegativeDiscount_thenThrowsException() {
Exception exception = assertThrows(IllegalArgumentException.class, () -> {
DiscountCalculator.calculateDiscount(1000.0, -0.10);
});
assertEquals("Discount cannot be negative", exception.getMessage());
}
@Test
void calculateDiscount_whenNoItems_thenReturnsOriginalPrice() {
double result = DiscountCalculator.calculateDiscount(0.0, 0.15);
assertEquals(0.0, result);
}
}
In the above example, you can see that the test names succinctly convey what each specific test validates, adhering to the conventions we've outlined.
The Bottom Line
Writing effective test names in JUnit is vital to creating a clear and maintainable test suite. By adopting practices like the Given-When-Then naming pattern, maintaining conciseness, prefixing test subjects, and avoiding common pitfalls, you set a solid foundation for your testing strategy.
Remember, a well-named test not only documents the behavior of your application but also contributes to smoother development and maintenance processes for you and your team.
For further reading on JUnit best practices, check out the official JUnit 5 documentation and Martin Fowler's Test Driven Development.
By mastering the art of effective test naming, you enhance the quality and clarity of your codebase—something every Java developer should embrace. Happy testing!
Checkout our other articles