Navigating Database Isolation Levels for Optimal Performance
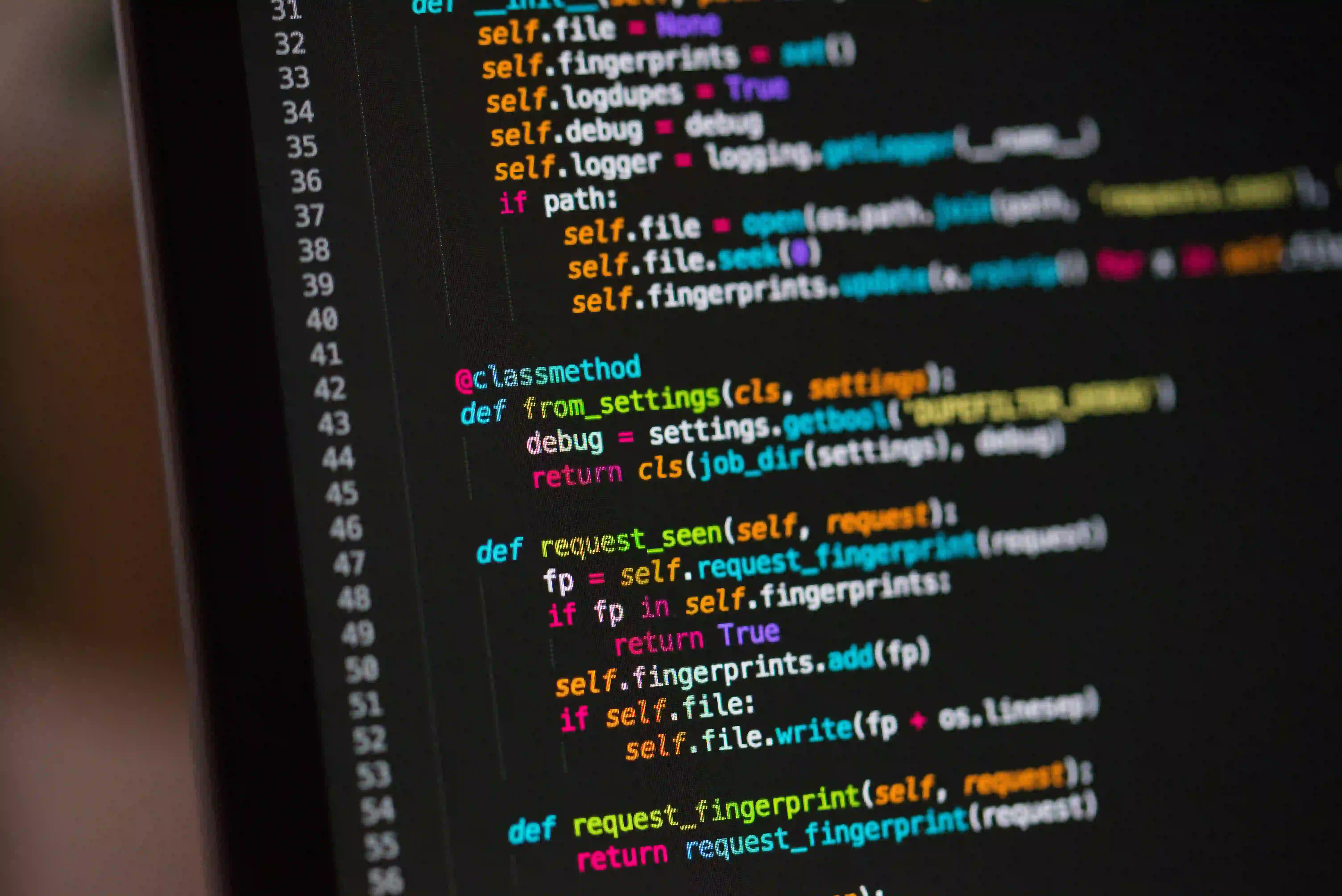
Navigating Database Isolation Levels for Optimal Performance
When working with databases, ensuring data consistency, performance, and reliability is paramount. One of the pivotal aspects of achieving these objectives is understanding and managing database isolation levels. This blog post dives into isolation levels, elucidating their importance, and how you can leverage them for optimal performance in your applications.
What Are Database Isolation Levels?
Database isolation levels determine how transaction integrity is visible to other transactions during concurrent execution. They dictate how transaction changes are handled concerning other transactions, which is crucial in multi-user environments. The main types of database isolation levels are defined by the SQL standard:
- Read Uncommitted
- Read Committed
- Repeatable Read
- Serializable
Let’s explore each of these isolation levels in detail, discussing their characteristics, advantages, and drawbacks.
1. Read Uncommitted
Definition: This is the most permissive isolation level, allowing transactions to read changes made by others even if those changes have not been committed yet.
Advantages:
- High performance due to minimal locking.
- Ideal for situations where data accuracy isn’t critical.
Drawbacks:
- Susceptible to dirty reads, where a transaction may read data that another transaction has not yet committed.
Example:
Consider two transactions, T1 and T2. If T1 modifies a record but does not commit it, T2 can read this uncommitted change.
// Setting isolation level to Read Uncommitted
Connection connection = DriverManager.getConnection(url);
connection.setAutoCommit(false);
connection.createStatement().execute("SET TRANSACTION ISOLATION LEVEL READ UNCOMMITTED");
Why: Using Read Uncommitted allows for high concurrency but at the risk of dirty data being read.
2. Read Committed
Definition: This isolation level ensures that transactions can only read changes that have been committed.
Advantages:
- No dirty reads occur.
- Offers a balance between performance and accuracy.
Drawbacks:
- Potential for non-repeatable reads, where data can change between reads in the same transaction.
Example:
Imagine the following scenario, where T1 commits a change between reads performed by T2.
// Setting isolation level to Read Committed
connection.createStatement().execute("SET TRANSACTION ISOLATION LEVEL READ COMMITTED");
Why: Choosing Read Committed protects against dirty reads, which is important for most applications that require some level of accuracy.
3. Repeatable Read
Definition: At this level, once a row is read, it cannot be changed by other transactions until it is finished. This level protects against both dirty and non-repeatable reads.
Advantages:
- Better consistency.
- Prevents non-repeatable reads.
Drawbacks:
- More locking compared to Read Committed, which may lead to performance bottlenecks.
- Can lead to phantom reads, where new rows can appear in subsequent reads.
Example:
// Setting isolation level to Repeatable Read
connection.createStatement().execute("SET TRANSACTION ISOLATION LEVEL REPEATABLE READ");
Why: This isolation level is useful when you need to ensure consistent data reads throughout a transaction, but it can reduce concurrency.
4. Serializable
Definition: This is the strictest isolation level. It ensures complete isolation from other transactions, making it appear as if transactions are executed serially.
Advantages:
- Full consistency, preventing all phenomena (dirty reads, non-repeatable reads, and phantom reads).
Drawbacks:
- Results in significant performance degradation due to extensive locking. Potential for increased deadlocks.
Example:
// Setting isolation level to Serializable
connection.createStatement().execute("SET TRANSACTION ISOLATION LEVEL SERIALIZABLE");
Why: Serializable is crucial for high-stakes operations necessitating absolute data integrity, such as financial transactions.
Choosing the Right Isolation Level
The choice of isolation level directly impacts your application’s performance and data integrity. Here are some guidelines to help you select the appropriate level:
-
High-concurrency applications: If your application demands high throughput with lower accuracy requirements, read uncommitted or read committed might be appropriate.
-
Financial applications: Use repeatable read or Serializable to prevent any anomalies.
-
Reporting tools: Read committed is often adequate, allowing faster read requests while not compromising too much on accuracy.
Real-world Considerations
When evaluating isolation levels, keep in mind:
-
Application Context: Always consider the context in which your application operates. Some require stricter controls than others.
-
Database Technology: Different databases (MySQL, PostgreSQL, Oracle) may implement these isolation levels with slight variations. Reference the documentation corresponding to your database for accurate practices. For broader coverage, check Database Isolation Levels.
-
Testing: Simulate various transaction loads to measure how your choice impacts performance in real-world scenarios.
-
Performance Monitoring: Constantly monitor your database’s performance metrics. Databases can exhibit varying behaviors based on current loads and configurations.
Lessons Learned
Mastering database isolation levels is fundamental for developers looking to achieve optimal performance and data integrity. By understanding the nuances of each level, you can make informed choices that align with your application's requirements.
For more in-depth information, the following resources can further enhance your knowledge:
- Understanding SQL Transaction Isolation Levels
- Concurrency Control in SQL Server
As you develop and deploy applications, take the time to explore these isolation levels. By carefully selecting an appropriate level for your application, you can enhance both performance and data reliability. Happy coding!