Java High CPU Usage: Key Fixes You Need to Know
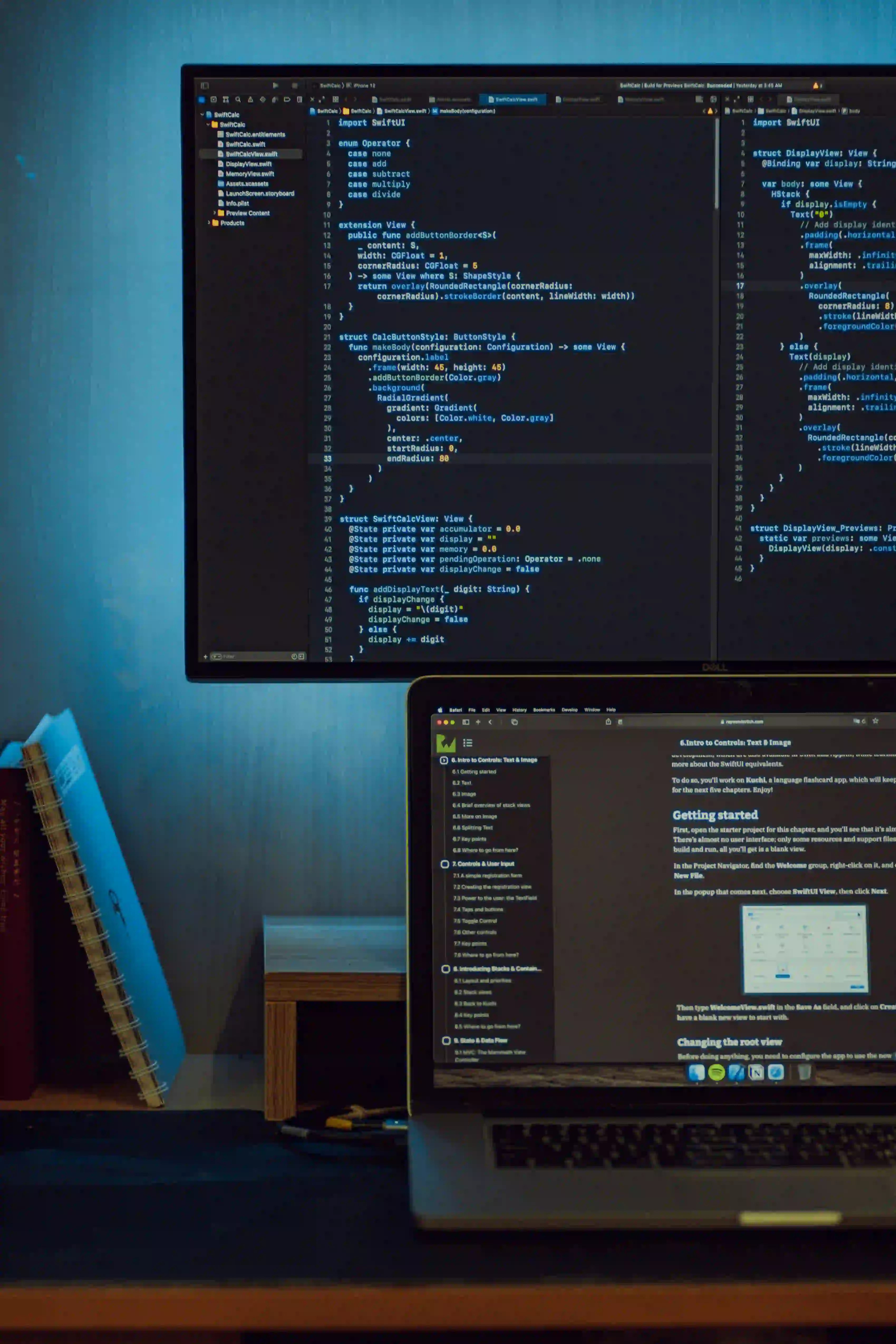
Java High CPU Usage: Key Fixes You Need to Know
Java is a robust language that powers many applications globally, but it is not immune to performance issues. One of the most common problems developers face is high CPU usage. This post will discuss the causes of high CPU usage in Java applications, provide essential fixes, and include practical code snippets to help you troubleshoot effectively.
Understanding High CPU Usage in Java
High CPU usage in a Java application can severely degrade performance and lead to scalability problems. Before diving into solutions, it's crucial to understand possible causes:
- Inefficient Algorithms: Algorithms that consume excessive CPU cycles can lead to inefficiencies.
- Thread Contention: Too many threads can increase CPU usage as the JVM constantly manages them.
- Garbage Collection: Java uses a garbage collector that can spike the CPU when trying to reclaim memory.
- Infinite Loops: Unintentional infinite loops can cause a spike in CPU usage as the application runs code repeatedly without terminating.
Diagnosing High CPU Usage
Before you can fix high CPU usage, you need to diagnose the problem. Here are steps to monitor CPU usage in Java applications.
1. Java Profilers
Profilers like VisualVM or JProfiler can provide insights into CPU usage. They help you identify which methods consume the most CPU time.
2. JConsole
Java Management Extensions (JMX) provides tools like JConsole, which can monitor JVM statistics. JConsole provides a graphical interface to view memory, threads, and CPU usage.
Code Snippet: Using JMX with Java
To leverage JMX in your application, you can enable it while starting your JVM:
java -Dcom.sun.management.jmxremote -Dcom.sun.management.jmxremote.port=9999 \
-Dcom.sun.management.jmxremote.authenticate=false \
-Dcom.sun.management.jmxremote.ssl=false \
-jar yourapp.jar
Why: This configuration allows you to connect to your application remotely using JConsole or any other JMX client to monitor its performance in real-time.
Common Fixes for High CPU Usage
Once you've diagnosed the issue, you can implement the following fixes to reduce CPU usage.
1. Optimize Algorithms
If your algorithm is inefficient, re-evaluating your approach may yield significant improvements.
Example: Using a HashMap Instead of a List
When searching for items frequently, consider substituting a List with a HashMap to improve lookup performance.
// Using a List
List<String> items = new ArrayList<>();
items.add("item1");
items.add("item2");
boolean exists = items.contains("item1"); // O(n)
// Using a HashMap
Map<String, Boolean> itemsMap = new HashMap<>();
itemsMap.put("item1", true);
itemsMap.put("item2", true);
boolean existsInMap = itemsMap.containsKey("item1"); // O(1)
Why: The HashMap look-up time is O(1), while the List’s is O(n). Optimizing this can lead to reduced CPU usage, especially in larger datasets.
2. Avoid Thread Contention
Thread contention occurs when multiple threads try to access a shared resource. Minimize this by using more granular synchronization or concurrency utilities from java.util.concurrent
.
Example: Using Locks
Rather than synchronizing an entire method, you can use more fine-grained control with locks:
private final Lock lock = new ReentrantLock();
public void safeMethod() {
lock.lock();
try {
// Critical section of code
} finally {
lock.unlock();
}
}
Why: Using locks allows different threads to work on non-conflicting aspects of a method simultaneously, reducing CPU usage caused by extensive locking.
3. Tune Garbage Collection
Garbage collection is crucial in Java, but it can become a CPU hog, especially in memory-intensive applications. You can use different GC algorithms depending on your application's needs.
Example: Using G1 Garbage Collector
You can specify the G1 garbage collector, which is designed for applications with larger heaps:
java -XX:+UseG1GC -jar yourapp.jar
Why: The G1 collector performs concurrent garbage collection and minimizes pause times, leading to better overall performance and lower CPU spikes.
4. Monitor & Optimize Infinite Loops
In some cases, you might unknowingly create an infinite loop. Always ensure terminating conditions are met.
Example: Fixing an Infinite Loop
// Potential infinite loop
while (true) {
// Perform operations...
}
// Corrected version
while (conditionNotMet) {
// Perform operations...
}
Why: Efficiently managing loops helps prevent unnecessary CPU cycles being consumed due to a runaway process.
5. Review Third-Party Libraries
Sometimes, the issue lies not within your code, but rather in the libraries you are using. Review them and ensure they are optimized or explore alternatives.
6. Scale Your Architecture
In some cases, infrastructure can limit application performance. Consider scaling vertically (more powerful machine) or horizontally (more machines) to manage increased loads.
To Wrap Things Up
High CPU usage in Java applications can lead to severe performance issues. By meticulously diagnosing the problem and applying effective fixes, such as optimizing algorithms, avoiding thread contention, tuning garbage collection, and ensuring your code is bug-free, you can substantially improve application performance.
If you find high CPU usage difficult to tackle, consider investing time in profiling tools like VisualVM or JProfiler to gain deeper insights.
Remember, performance optimization is a continuous process. Regularly revisiting your code and monitoring your application will help you maintain an optimal state. Stay informed of the latest advancements in Java performance management to ensure your applications run smoothly.
By implementing these strategies, not only do you ensure a more responsive application, but you also enhance the user experience significantly. Happy coding!
Additional Resources
- Java Performance Tuning
- JVM Garbage Collection: An Overview
- Concurrency in Java: A Comprehensive Guide