Why Inlined Virtual Methods in Java Can Hurt Performance
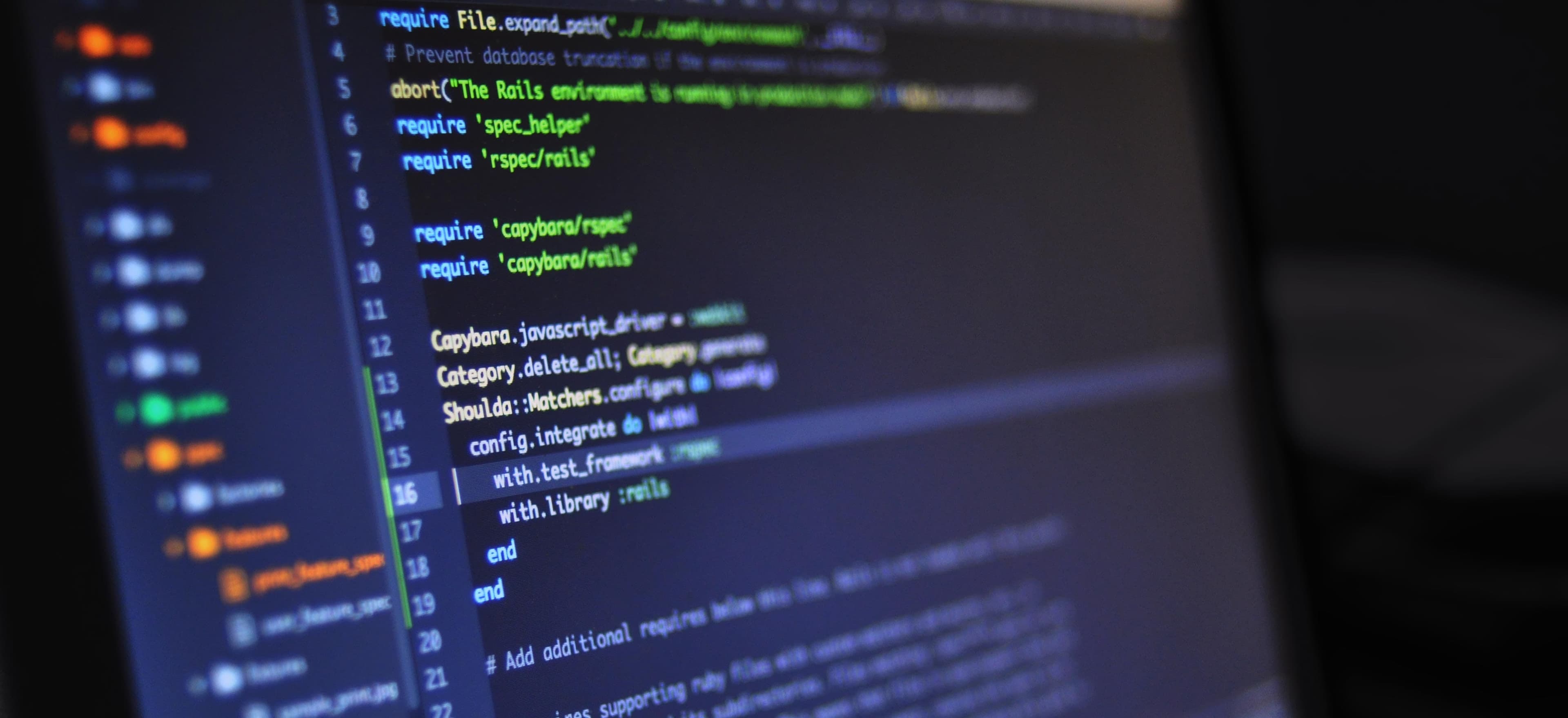
- Published on
Why Inlined Virtual Methods in Java Can Hurt Performance
Java is widely praised for its performance characteristics, thanks in large part to the optimization techniques employed by the Java Virtual Machine (JVM). One of the key features of the JVM is its ability to perform Just-In-Time (JIT) compilation, which includes the process of inlining methods. However, while inlined methods can improve performance, there are instances where inlined virtual methods can indeed hurt performance. In this blog post, we will discuss the mechanics of inlining, explore its implications in Java, and highlight scenarios where inlining might not be beneficial.
What is Method Inlining?
Method inlining is a compiler optimization technique that replaces a method call with the actual method body. This can help reduce the overhead involved in method calls, particularly for small methods. The primary goal is to enhance execution speed by eliminating the calling convention overhead.
For instance, consider the following simple method:
public int add(int a, int b) {
return a + b;
}
If this method is frequently called, the JVM may decide to inline it during JIT compilation:
// Instead of calling add(a, b)
int result = a + b; // Inlined version
How JIT Compilation Works
The JIT compiler compiles Java bytecode into native machine code at runtime. The JVM uses profiling data to determine which methods are frequently executed. It compiles these methods into optimized machine code, applying various optimization techniques, including inlining.
Pros of Method Inlining
-
Reduced Call Overhead: Inlining eliminates the overhead of method calls. This leads to faster execution, especially for small or frequently called methods.
-
Increased Optimization Opportunities: Inlined methods allow the compiler to optimize more effectively since it has visibility into the entire method body, making it easier to perform other optimizations like constant folding and dead code elimination.
-
Complex Method Optimization: When multiple methods are inlined, the compiler may also optimize them collectively, further enhancing performance.
The Dark Side of Inlining
Despite its advantages, inlining comes with its own set of challenges and potential pitfalls.
1. Increased Code Size
When methods are inlined, the overall code size can increase dramatically, especially if a small method is called from multiple locations.
public void foo() {
bar();
bar();
bar(); // If bar() is inlined, it increases the code size significantly
}
If bar()
has a size of 10 bytecodes, inlining it three times in foo()
increases the code size by 30 bytecodes. This can lead to larger compiled classes and increased memory usage.
2. Cache Issues
With increased code size comes the potential for cache issues. Modern CPUs utilize several levels of cache for performance optimization. A larger code footprint may lead to cache misses, diluting the performance gains from inlining.
The Performance Trade-off
The performance implications of inlined virtual methods can vary based on the following factors:
1. Method Size and Complexity
Inlining works best for small and simple methods. Inlining larger methods can significantly increase the compiled code size, leading to the aforementioned cache issues.
// A simple method that is a good candidate for inlining
public int add(int a, int b) {
return a + b;
}
// A large method that is not a good candidate for inlining
public void complexCalculation() {
// Imagine a complex operation with many instructions
}
2. Frequency of Use
Methods that are called frequently are ideal candidates for inlining. However, rarely used methods may cause unnecessary code bloat.
3. Virtual Dispatch Overhead
In Java, methods are often virtual, meaning the exact method being executed could differ based on the object's runtime type. This virtual dispatch can complicate inlining, especially if the method belongs to a subclass.
The JVM can perform an optimization called "devirtualization," which allows it to inline some virtual method calls by knowing the specific type of the object at runtime. However, if the object can change types, the potential for decreased performance increases due to the associated complexity of managing multiple versions.
When Inlined Virtual Methods Hurt Performance
Let's examine specific scenarios where inlining virtual methods can adversely affect performance:
1. Extensive Use of Inheritance
In a complex class hierarchy, the JVM might struggle to determine the appropriate method to inline due to multiple override possibilities. This can lead to excessive inlining of virtual method calls, causing the compiled code to bloat and cache inefficiencies.
2. High Polymorphism
If your application makes extensive use of interfaces and abstract classes, the JVM may end up inlining methods from many derived classes. With numerous potential instances, the resulting code size can skyrocket, undermining performance.
3. Hot Method Invocations
Hot methods are those that are executed frequently. If you have a scenario where a method is called in a loop, and it eventually gets inlined, the overhead incurred from the larger code size may exceed the benefits.
for(int i = 0; i < 1000000; i++) {
hotMethod(); // If very large, this could cause cache misses
}
Best Practices to Consider
To mitigate the pitfalls of inlined virtual methods, consider the following practices:
-
Profile Your Code: Always profile your application to identify hotspots and understand where to focus optimization efforts.
-
Balance Between Size and Speed: Rigorously evaluate the trade-offs between code size and execution speed. Not all methods warrant inlining.
-
Use Annotations: If employing a framework that allows annotations, consider using tools like
@Inline
or@NoInline
to have finer control over method calls. -
Limit Inheritance Depth: When designing your classes, try to limit inheritance depth. This can help reduce the complexity of virtual dispatch at runtime.
-
Avoid Complex Method Bodies: Keep methods simple. If a method is too complex, it is likely not a good candidate for inlining.
Key Takeaways
While method inlining is a powerful optimization technique employed by the JVM, it is crucial to recognize that inlined virtual methods can sometimes hurt performance. By understanding the factors that influence these optimizations and implementing best practices, developers can leverage the benefits of inlining while minimizing potential downsides.
For further reading, consider these resources:
- Java Performance: The Definitive Guide
- Understanding Inlining in the JVM
By being mindful of inlining practices and understanding the JVM's behavior, developers can optimize their Java applications more effectively while ensuring performance remains at an optimal level.