Boost Your Testing Efficiency with Selenium 4 Relative Locators
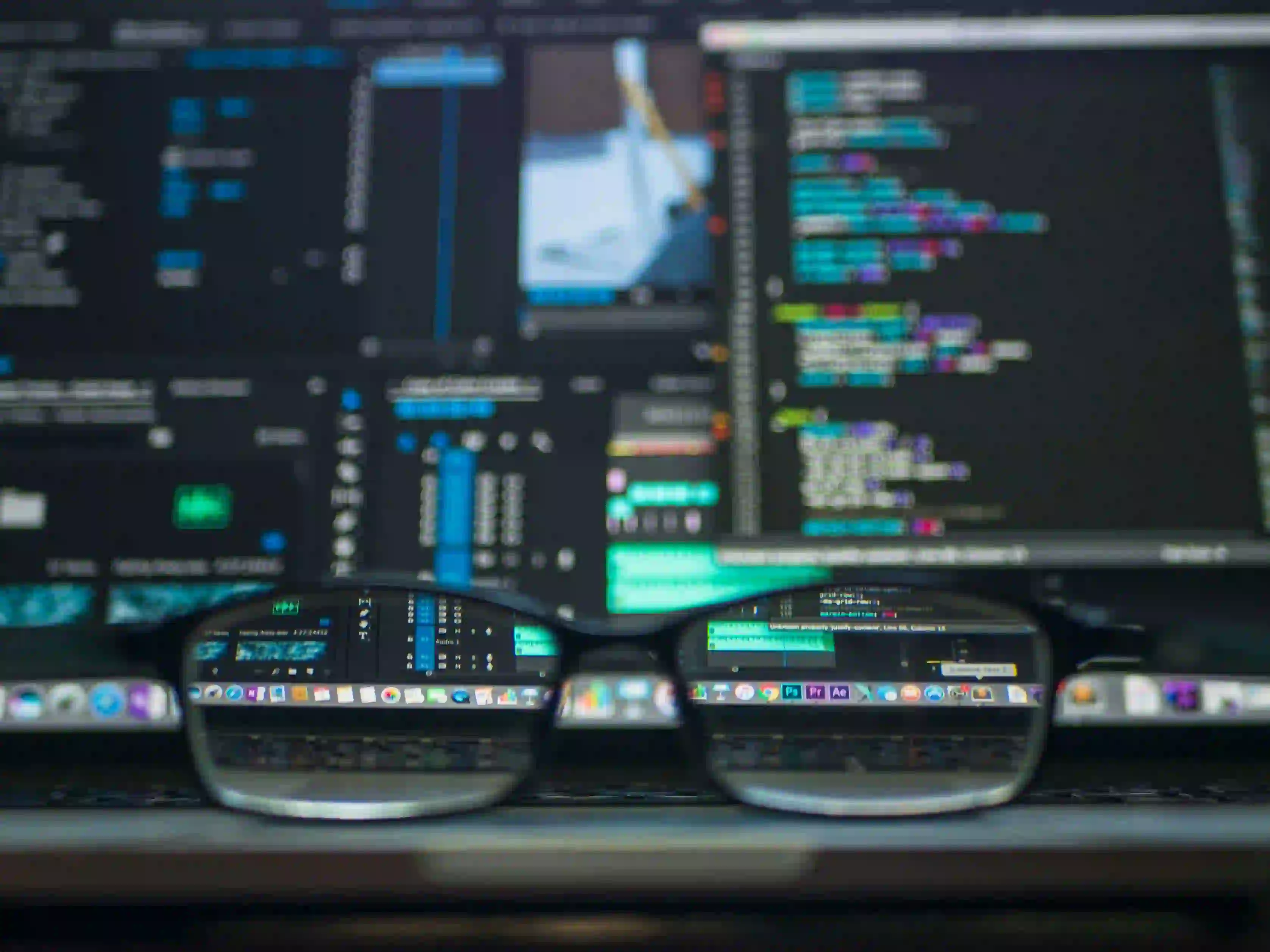
Boost Your Testing Efficiency with Selenium 4 Relative Locators
Selenium has long been the go-to tool for web application testing, and the release of Selenium 4 brings with it exciting new features that enhance the testing experience. Among these features are relative locators, a powerful and flexible way to identify web elements on a page. In this blog post, we will explore what relative locators are, why they can significantly boost your testing efficiency, and how to implement them effectively in your Selenium tests.
Understanding Relative Locators
Relative locators allow testers to locate elements based on their position relative to other elements on a web page. This method comes particularly handy in complex layouts where conventional locators such as ID, class name, or XPath may be insufficient or cumbersome.
Why Use Relative Locators?
-
Enhanced Readability: Relative locators are designed to be more intuitive. Instead of reading through a long XPath expression, you can describe the position of an element in relation to other elements, which makes your code easier to understand and maintain.
-
Increased Resilience: Web layouts change frequently. Relative locators can be more adaptable to these changes. If the structure around an element changes, the relative locator may still find it successfully, where a conventional locator might fail.
-
Simplicity: Often, writing complex XPaths can lead to mistakes or confusion in skin tests. Relative locators use simplistic language which can save time when writing and debugging tests.
How to Use Relative Locators in Selenium 4
Prerequisites
Before we dive into the code snippets, ensure you have the following set up:
- Java JDK installed.
- Maven installed for dependency management.
- Selenium 4 Maven dependencies added to your
pom.xml
:
<dependency>
<groupId>org.seleniumhq.selenium</groupId>
<artifactId>selenium-java</artifactId>
<version>4.0.0</version>
</dependency>
Code Snippet
Here's a simple example demonstrating how to use relative locators in Selenium 4. For this example, assume you are testing a login form:
import org.openqa.selenium.By;
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.WebElement;
import org.openqa.selenium.chrome.ChromeDriver;
import org.openqa.selenium.support.locators.RelativeLocator;
public class RelativeLocatorsExample {
public static void main(String[] args) {
System.setProperty("webdriver.chrome.driver", "path/to/chromedriver");
WebDriver driver = new ChromeDriver();
driver.get("http://example.com/login");
// Locate the username input field by its label
WebElement usernameLabel = driver.findElement(By.xpath("//label[text()='Username']"));
WebElement usernameField = driver.findElement(RelativeLocator.with(By.tagName("input")).toRightOf(usernameLabel));
// Enter username
usernameField.sendKeys("testuser");
// Locate the password input field by its label
WebElement passwordLabel = driver.findElement(By.xpath("//label[text()='Password']"));
WebElement passwordField = driver.findElement(RelativeLocator.with(By.tagName("input")).toRightOf(passwordLabel));
// Enter password
passwordField.sendKeys("password123");
driver.quit();
}
}
Commentary
In this code snippet:
- We initialize the WebDriver and navigate to a hypothetical login page.
- We locate the username input field relative to its label using
RelativeLocator.with(By.tagName("input")).toRightOf(usernameLabel)
. This is elegant and straightforward, as it clearly indicates that you are finding the input field to the right of the label. - Similarly, we apply the same logic for the password input field.
This clear, readable syntax increases the maintainability of your test code.
Types of Relative Locators
Selenium 4 provides the following methods for relative locators:
toLeftOf
toRightOf
above
below
near
These options give you flexibility in describing an element's location relative to another, enhancing your ability to navigate complex DOM structures.
Examples of Using Different Relative Locators
Let's explore how to use these different locators effectively. Below are additional examples of how to find elements using various relative positions.
Locating an Element Above
Suppose you have a web form with a “Submit” button located above a “Clear” button, you can use:
WebElement clearButton = driver.findElement(By.id("clear"));
WebElement submitButton = driver.findElement(RelativeLocator.with(By.tagName("button")).above(clearButton));
Locating an Element Below
In a scenario with a message displayed below an input field:
WebElement inputField = driver.findElement(By.id("input"));
WebElement message = driver.findElement(RelativeLocator.with(By.tagName("div")).below(inputField));
Locating a Nearby Element
You might want to locate an element that is close to another without specifying exact positions:
WebElement referenceElement = driver.findElement(By.className("reference"));
WebElement nearbyElement = driver.findElement(RelativeLocator.with(By.tagName("span")).near(referenceElement));
Best Practices for Using Relative Locators
While relative locators are convenient, here are a few best practices to keep in mind:
- Limit Use: Use relative locators primarily in scenarios where traditional locators struggle; don't abandon them entirely.
- Test Resilience: Ensure that your tests continue to pass as the UI evolves. Conduct regular maintenance to confirm that relative locators are still accurately targeting the intended elements.
- Combine Locators: Using relative locators in combination with conventional locators can yield the best results in more complex scenarios.
A Final Look
The introduction of relative locators in Selenium 4 offers significant advantages in terms of both readability and reliability. By adopting these new features, you can streamline your test automation efforts, making your code more intuitive and adaptable to future changes.
To dive deeper into Selenium and its various methods, check out the Selenium Documentation. As we move towards more responsive and fluid web designs, understanding innovative testing techniques like relative locators will help you stay ahead of the curve.
Selenium 4 is not just an update; it redefines how we think about element identification. Embrace it, and you will undoubtedly boost your testing efficiency.
Feel free to leave your thoughts, suggestions, or questions in the comments below. Happy testing!