Common CORS Issues in Spring Boot and How to Fix Them
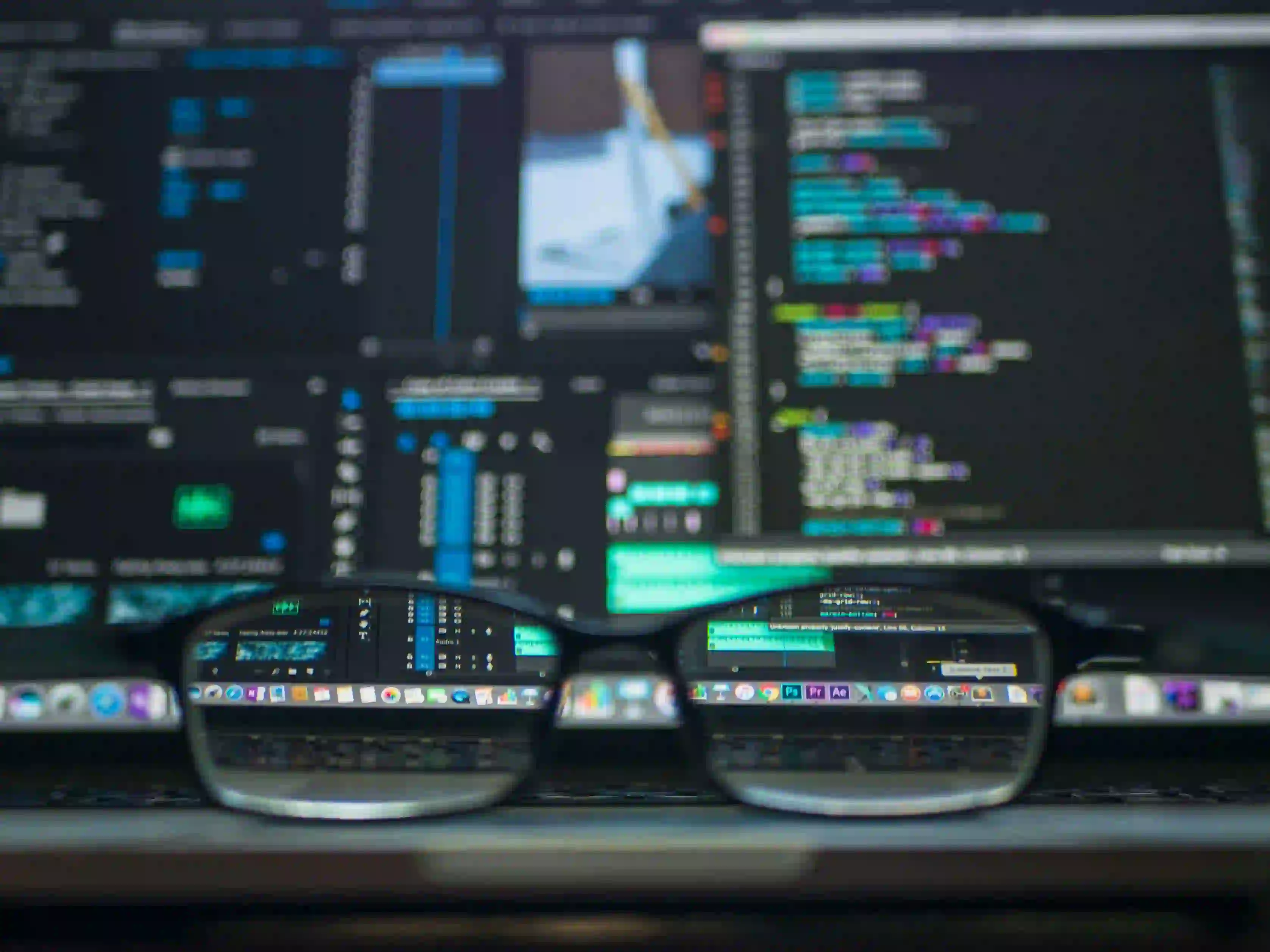
Common CORS Issues in Spring Boot and How to Fix Them
CORS, or Cross-Origin Resource Sharing, is a critical security feature that allows or restricts resources requested on a web page from a different origin than the one the page was hosted on. In a Spring Boot application, managing CORS effectively is vital for the security and performance of your web applications. In this post, we will explore common CORS issues faced in Spring Boot and how to resolve them.
What is CORS?
CORS is a mechanism that uses HTTP headers to tell browsers to give a web application running at one origin (domain) access to selected resources from a different origin. The Same-Origin Policy, which is the default behavior, restricts how documents or scripts loaded from one origin can interact with resources from another origin.
For example, if your JavaScript application at http://example.com
tries to fetch data from http://api.example.com
, the browser will block this request unless CORS headers are present on the server.
Common CORS Issues
1. Simple CORS Issues
The first and simplest issue developers face involves not having any CORS configurations in place. This often leads to CORS Policy: No 'Access-Control-Allow-Origin' header is present on the requested resource.
errors in your console.
2. Preflight Requests Fail
When a web application makes a complex request (like PUT or DELETE), the browser sends a preflight OPTIONS request first. If this request fails or is not handled correctly, it leads to failures in accessing the main resource.
3. Incorrect Headers
Sometimes, responses may have incorrect headers or missing headers required for CORS. For example, not allowing credentials when they are needed can lead to issues where the request is blocked.
4. Whitelisting Origins
When dealing with multiple client domains, a common issue is misconfiguration while whitelisting specific origins. This might lead to access issues for legitimate clients.
How to Fix CORS Issues in Spring Boot
Spring Boot provides excellent support for CORS configuration, making it easy to resolve common issues related to cross-origin requests. Let’s walk through various ways to set up CORS properly.
Method 1: Global CORS Configuration Using WebMvcConfigurer
One of the preferred methods to configure CORS for your entire application is by implementing the WebMvcConfigurer
interface.
import org.springframework.context.annotation.Bean;
import org.springframework.context.annotation.Configuration;
import org.springframework.web.servlet.config.annotation.CorsRegistry;
import org.springframework.web.servlet.config.annotation.WebMvcConfigurer;
@Configuration
public class WebConfig implements WebMvcConfigurer {
@Override
public void addCorsMappings(CorsRegistry registry) {
registry.addMapping("/**") // Allow all paths
.allowedOrigins("http://example.com", "http://another-example.com") // Whitelist these origins
.allowedMethods("GET", "POST", "PUT", "DELETE", "OPTIONS") // The allowed HTTP methods
.allowCredentials(true) // Allow credentials
.maxAge(3600); // Cache preflight response for 1 hour
}
}
Explanation
-
addMapping("/**")
: This allows CORS for all endpoints in the application. -
allowedOrigins(...)
: This is where you specify which origins can access your resources. Be restrictive here for better security. -
allowedMethods(...)
: This enumerates the allowed HTTP methods, which is crucial for appropriately handling requests. -
allowCredentials(true)
: This allows cookies and HTTP authentication to be included in CORS requests. -
maxAge(...)
: This defines the duration the preflight request can be cached. It reduces the number of preflight requests.
Method 2: CORS Configuration on Controller Level
You can also configure CORS on specific controllers or handler methods if you require more granular control.
import org.springframework.web.bind.annotation.CrossOrigin;
import org.springframework.web.bind.annotation.RestController;
import org.springframework.web.bind.annotation.RequestMapping;
@RestController
@CrossOrigin(origins = "http://example.com", maxAge = 3600)
public class MyController {
@RequestMapping("/api/data")
public String getData() {
return "Secure data"; // This endpoint is accessible from specified origin
}
}
Explanation
Using the @CrossOrigin
annotation provides straightforward React configuration tailored to a specific controller or method. It’s simpler for REST APIs with tight access.
Method 3: Configuring CORS in application.properties
For newer versions of Spring Boot (2.4 and above), you might also configure CORS via properties. This may not cover all scenarios, but it is useful for a quick setup.
spring.web.cors.allowedOrigins=http://example.com
spring.web.cors.allowedMethods=GET,POST,PUT,DELETE,OPTIONS
spring.web.cors.allow-credentials=true
Diagnosing CORS Issues
-
Check Console Logs: React or Angular applications often log CORS issues clearly, helping you identify the missing headers quickly.
-
Browser Developer Tools: Use Chrome’s or Firefox’s developer tools to inspect network requests and see the headers sent and received.
-
Use Tools Like Postman: It can mimic requests manually, bypassing CORS, allowing you to troubleshoot on the server side directly.
Additional Resources
Bringing It All Together
CORS issues in Spring Boot can be easily managed with proper configurations, enabling smooth communication between different origins. By implementing global configurations or local overrides, you can adapt your application to handle cross-origin requests effectively.
Understanding how CORS works and applying the aforementioned strategies will save you time and effort while enhancing the security of your web applications. Always bear in mind that while allowing cross-origin requests can facilitate interaction between various web applications, it should be done cautiously to mitigate potential security risks.
Feel free to explore and modify the code snippets provided to fit your application's requirements, and soon you will have a well-functioning CORS setup in your Spring Boot applications. Happy coding!