How Cached Prepared Statements Can Lead to Memory Issues in Cassandra
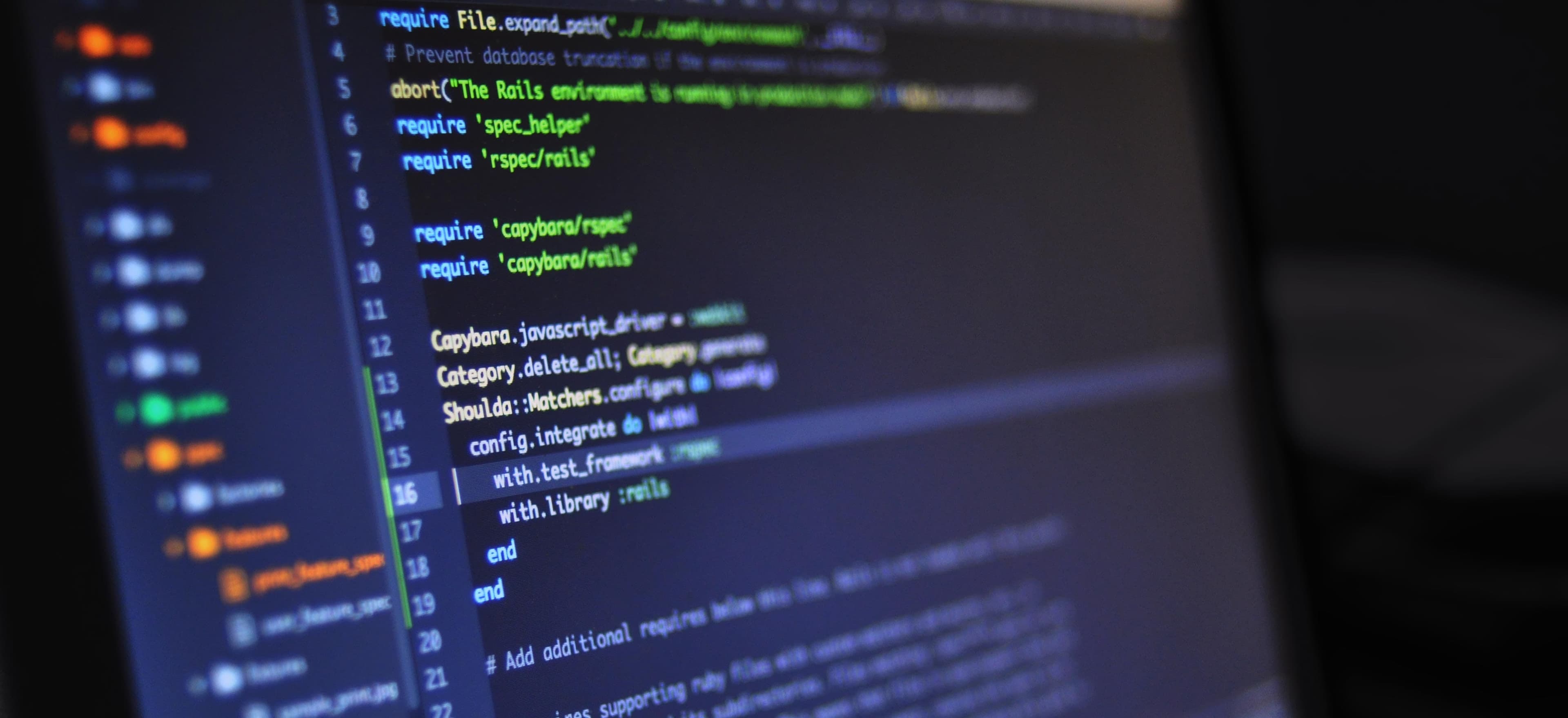
- Published on
How Cached Prepared Statements Can Lead to Memory Issues in Cassandra
Cassandra is a highly distributed NoSQL database known for its scalability and high availability. One of its key features is the ability to prepare statements, which can significantly improve the performance of query execution. However, relying heavily on cached prepared statements can lead to memory issues if not managed carefully. In this blog post, we will explore how cached prepared statements work in Cassandra, the potential memory pitfalls they create, and strategies to mitigate these issues.
Understanding Prepared Statements
A prepared statement is a feature that allows you to pre-compile and cache a query plan. This means that when you execute the statement multiple times with different parameters, Cassandra does not need to parse and compile the query each time. This can greatly reduce latency and improve throughput in applications with high read/write operations.
Example of a Prepared Statement
Here’s how you can create and use a prepared statement in Java with the DataStax Java driver for Cassandra:
import com.datastax.oss.driver.api.core.CqlSession;
import com.datastax.oss.driver.api.core.cql.PreparedStatement;
import com.datastax.oss.driver.api.core.cql.Binding;
import com.datastax.oss.driver.api.core.cql.ResultSet;
public class PreparedStatementExample {
public static void main(String[] args) {
try (CqlSession session = CqlSession.builder().build()) {
// Create a prepared statement
PreparedStatement preparedStatement = session.prepare("INSERT INTO users (id, name) VALUES (?, ?)");
// Bind values and execute the prepared statement
ResultSet resultSet = session.execute(preparedStatement.bind(1, "John Doe"));
// Output success message
System.out.println("Insert successful: " + resultSet.wasApplied());
}
}
}
Why Use Prepared Statements?
- Performance: By reusing a prepared statement, Cassandra caches the execution plan, thereby reducing the overhead of repeatedly parsing and compiling queries.
- Security: Prepared statements help prevent SQL injection attacks by treating user input as parameters rather than executable code.
Caching Prepared Statements
Cassandra will cache these prepared statements to improve performance. However, this caching introduces potential memory issues, especially when applications generate a large number of unique prepared statements. Let's delve into why this happens.
Memory Issues with Cached Prepared Statements
1. Rapidly Growing Cache
Each unique prepared statement consumes memory. If your application dynamically generates a large number of unique statements, the cache can grow significantly.
For example, consider an application that generates a unique prepared statement for every user interaction, resulting in thousands or even millions of unique statements that are kept in memory.
2. Out of Memory Errors
When the cache grows too large, it can lead to OutOfMemoryError exceptions, causing your application to crash. If your application doesn't have adequate memory resources allocated, even a relatively small increase in the number of prepared statements can trigger a memory overflow.
3. Inefficient Memory Usage
If prepared statements are cached indefinitely, it can lead to memory being occupied by queries that are rarely accessed. This can degrade performance across the board, affecting overall application behavior.
Monitoring Prepared Statements
It is essential to monitor your application's usage of prepared statements to understand memory consumption. Cassandra provides several metrics that can help you in this regard:
- prepared_statements: Number of prepared statements in memory.
- prepared_statements_cache_size: Amount of memory allocated to the prepared statements cache.
Example Monitoring Configuration
You can enable monitoring by configuring the metrics in your Cassandra cassandra.yaml
file:
# Enable JMX monitoring
jmx_port: "7199"
# Enable the metrics system
metrics_enabled: true
You can then use JMX tools like VisualVM or JConsole to monitor your application's prepared statement cache.
Best Practices to Mitigate Memory Issues
To avoid memory issues associated with cached prepared statements in Cassandra, follow these best practices:
1. Limit the Number of Prepared Statements
Implement a limit to the number of unique prepared statements your application can create. Setting a cap on the number of cached prepared statements encourages reuse and keeps memory consumption manageable.
import java.util.HashMap;
import java.util.Map;
public class PreparedStatementManager {
private static final int MAX_PREPARED_STATEMENTS = 100;
private Map<String, PreparedStatement> statementCache = new HashMap<>();
public PreparedStatement getPreparedStatement(CqlSession session, String query) {
if (!statementCache.containsKey(query)) {
if (statementCache.size() >= MAX_PREPARED_STATEMENTS) {
evictOldestPreparedStatement();
}
statementCache.put(query, session.prepare(query));
}
return statementCache.get(query);
}
private void evictOldestPreparedStatement() {
String oldestKey = statementCache.keySet().iterator().next();
statementCache.remove(oldestKey);
}
}
2. Clear Cached Statements Regularly
Implement a mechanism to clear or evict unused statements from the cache. You could use strategies based on time lived (TTL) or least recently used (LRU) principles.
3. Use SQL Instead of Unique Queries
Review your application's logic to determine if you can consolidate multiple similar queries into fewer prepared statements with varying parameters. This reduces the number of unique queries that need to be cached.
4. Set Up Appropriate JVM Settings
Tune the Java Virtual Machine (JVM) settings to allocate the appropriate memory for your application. Pay attention to the heap size and garbage collection settings.
java -Xms512m -Xmx2048m -XX:+UseG1GC YourApp
A Final Look
Cached prepared statements can significantly enhance query performance in Cassandra. However, improper management can lead to substantial memory issues that can cripple an application. By monitoring prepared statement usage and implementing strategies to limit their growth, you can maintain efficient performance while mitigating risks associated with memory overload.
For further reading, explore the following resources:
- Cassandra Documentation
- DataStax Java Driver
By adopting these best practices, you can leverage the power of prepared statements in Cassandra while avoiding common pitfalls associated with their caching. Stay tuned for more insights on optimizing performance and resource management in Cassandra.