The Risks of Using Getter Dependency Injection in DI
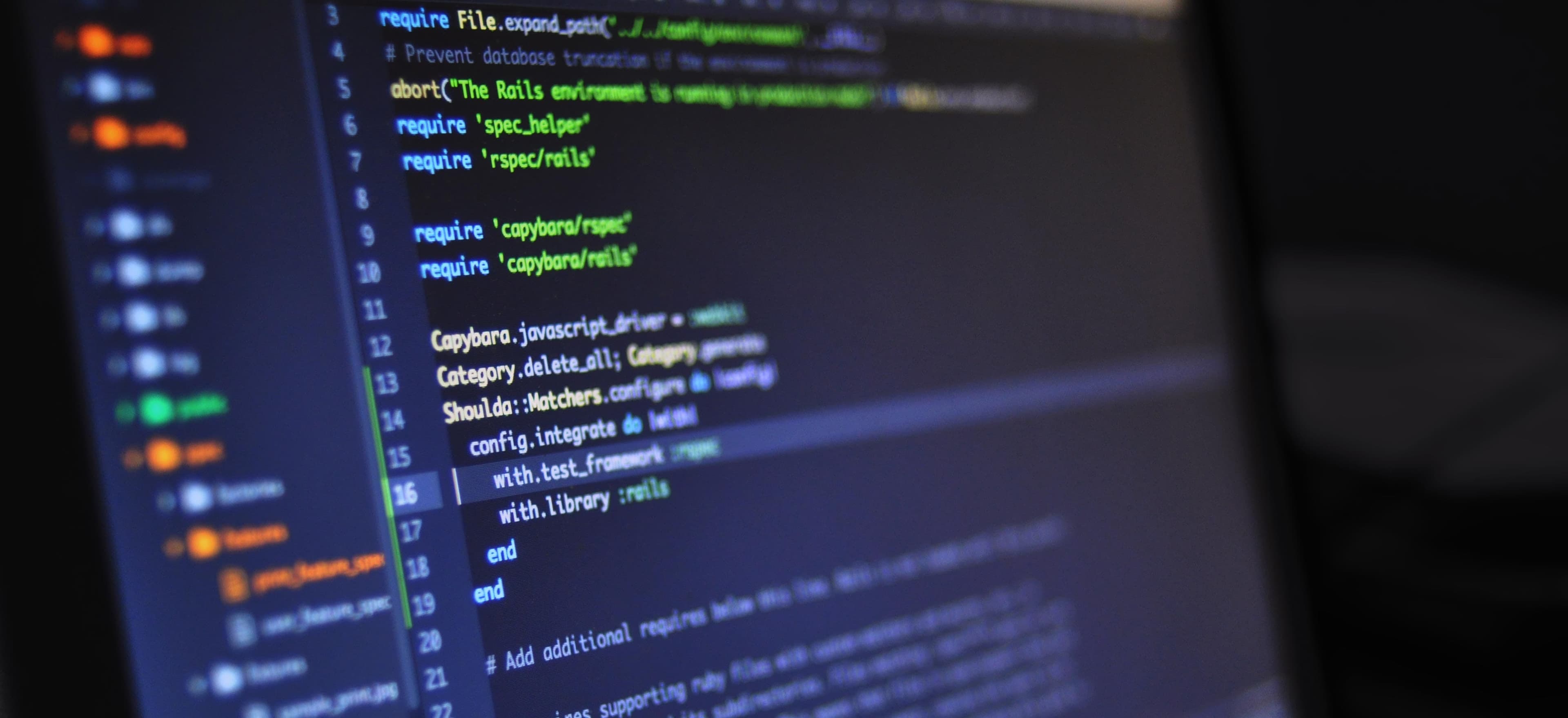
- Published on
The Risks of Using Getter Dependency Injection in DI
Dependency Injection (DI) is a powerful design pattern embraced by Java developers for creating loosely coupled, maintainable, and testable code. While it offers various methods of injecting dependencies, one approach that stands out is the use of getter methods. However, this technique comes with its set of risks and drawbacks that merit in-depth discussion.
In this blog post, we will explore the concept of Getter Dependency Injection, the issues it brings, and better alternatives to manage dependencies in Java applications. We'll delve into practical code examples, highlighting the pitfalls and offering better design practices.
What is Dependency Injection?
Before diving into the specifics of Getter Dependency Injection, let's review the primary concept of Dependency Injection.
DI is a software design pattern that enables a class to receive its dependencies from external sources rather than creating them internally. This promotes loose coupling between classes, leading to more maintainable code.
Typically, DI can be achieved in three main ways:
- Constructor Injection: Dependencies are provided through the class constructor.
- Setter Injection: Dependencies are provided through setter methods.
- Interface Injection: Dependencies are provided through an interface.
While these methods are widely acknowledged and used, getter injection involves a problematic approach. Let's discuss what Getter Dependency Injection entails and why it can be detrimental.
What is Getter Dependency Injection?
Getter Dependency Injection refers to the practice of injecting dependencies into a class by calling getters that return these dependencies. Instead of directly assigning dependencies via constructors or setters, the dependencies are fetched through public getter methods at the time of use.
Code Example of Getter Dependency Injection
public class UserService {
public UserRepository getUserRepository() {
return new UserRepository();
}
public void performAction() {
UserRepository repository = getUserRepository();
// Perform some action with the repository
}
}
In this example, the UserService
class retrieves its UserRepository
dependency through the getUserRepository()
method. While this approach might seem straightforward, it is not without its flaws.
The Risks of Using Getter Dependency Injection
1. Violation of Principle of Dependency Injection
One key goal of DI is to allow for easier testing and swapping of implementations. By fetching dependencies via getters, you're coupling your class to specific instances and making unit testing difficult.
When using constructor or setter injection, you can easily provide mock implementations during testing. However, with getter injection, the dependency is hard-coded, and substituting it in tests becomes cumbersome.
2. Hidden Dependencies
Hidden dependencies occur when a class uses dependencies without explicitly stating it. In the example above, UserService
implicitly relies on UserRepository
, but a developer reading UserService
might not understand this without looking into the getUserRepository()
implementation.
Explicitly stating dependencies via constructor or setter injection improves code readability and maintainability. It makes it clear what dependencies a class requires right at the class-level declaration.
3. Lifecycle Management Issues
When using getter methods, dependencies are often created during runtime. This can lead to various issues, such as creating multiple instances of a dependency when only one is needed.
public class UserService {
private UserRepository userRepository;
public UserRepository getUserRepository() {
// Each call returns a new instance
return new UserRepository();
}
public void performAction() {
UserRepository repository = getUserRepository(); // New instance per method call
// Perform some action with the repository
}
}
In this case, every time performAction()
is called, a new instance of UserRepository
is created rather than reusing an existing one.
4. Difficulties in Subclassing
Another risk of using getter injection is that it complicates subclassing. If a subclass needs different dependencies, it may have to override getter methods, potentially leading to fragile code.
When you rely on constructor or setter injection, subclasses can easily call parent constructors or setters to manage their dependencies. This creates a straightforward inheritance model, promoting better extendability.
5. Tight Coupling to Dependency Implementation
By injecting dependencies through getters, you're inherently tying the implementation of UserRepository
into UserService
. Changes to the UserRepository
or an alternative implementation could heavily impact the UserService
class.
In contrast, constructor or setter injection allows you to pass different implementations (such as mocks for testing, or different types of repositories) without modifying the class itself.
Better Alternatives to Getter Dependency Injection
Given the risks associated with Getter Dependency Injection, it is recommended to adopt more robust dependency injection methods. Here are two common alternatives:
1. Constructor Injection
Constructor injection is often seen as the gold standard for dependency injection. It guarantees the immutability of dependencies and ensures that a class has all the necessary dependencies at the point of creation.
public class UserService {
private final UserRepository userRepository;
public UserService(UserRepository userRepository) {
this.userRepository = userRepository;
}
public void performAction() {
// Use userRepository directly
}
}
In this example, UserRepository
is passed into the UserService
via the constructor, making dependencies clear and easily manageable.
2. Setter Injection
Setter injection is another valid approach where the dependencies are provided through public setter methods. This can be advantageous when dependencies are optional.
public class UserService {
private UserRepository userRepository;
public void setUserRepository(UserRepository userRepository) {
this.userRepository = userRepository;
}
public void performAction() {
// Use userRepository directly
}
}
This allows for some flexibility, but it's crucial to ensure that the setUserRepository
method is called before using the UserService
instance.
Final Thoughts
Getter Dependency Injection may appear to offer a quick solution to inject dependencies; however, it poses various risks and pitfalls that can undermine the quality, maintainability, and testability of your code. It creates hidden dependencies, violates principles of DI, and can lead to lifecycle management issues.
By choosing more robust alternatives such as constructor or setter injection, you can craft more reliable, readable, and maintainable Java applications.
If you want to delve deeper into Dependency Injection practices, consider visiting the following resources Spring Framework DI and Effective Java for extensive insights.
Feel free to share your experience with Dependency Injection and any challenges you have faced in the comments below!
Checkout our other articles