Selenium 4 Alpha: Key Features and Potential Pitfalls
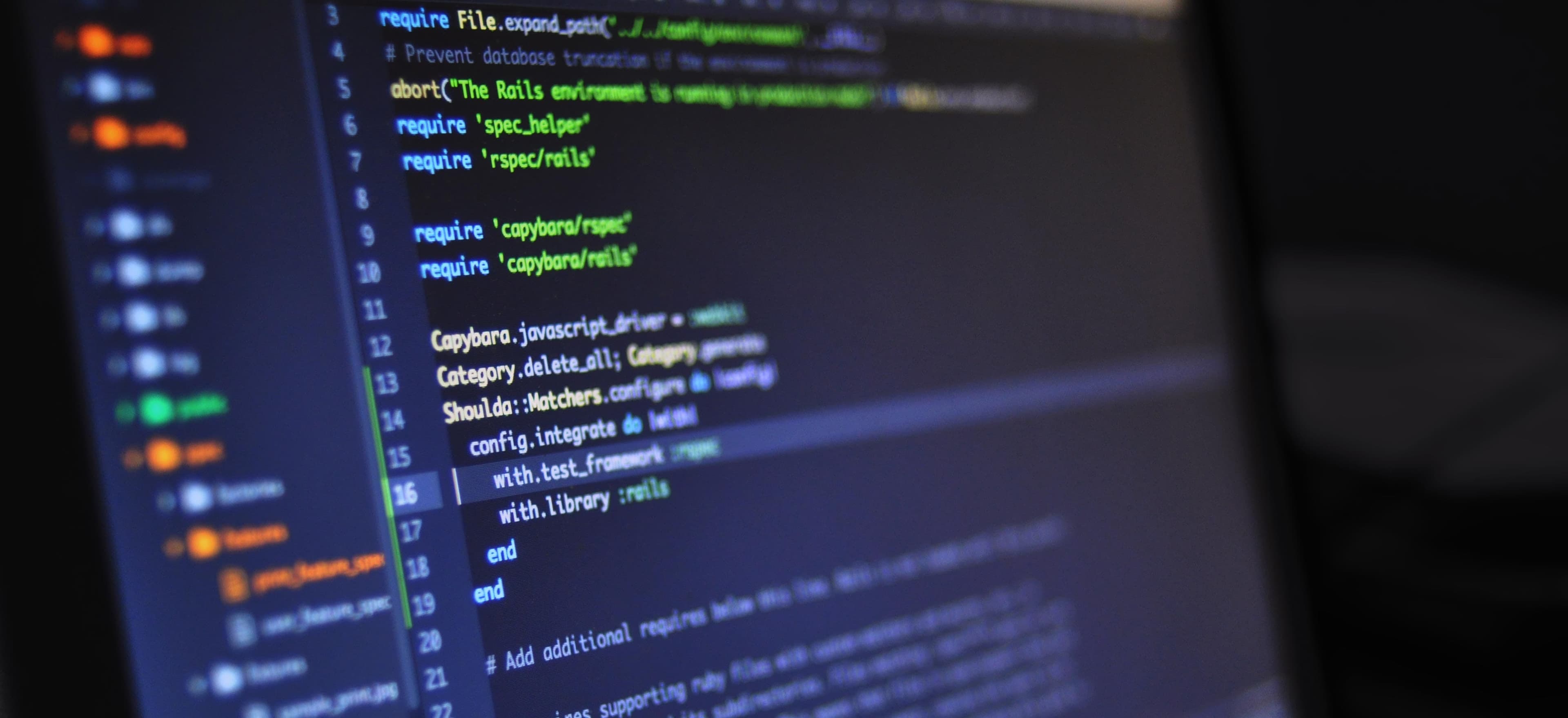
- Published on
Selenium 4 Alpha: Key Features and Potential Pitfalls
Selenium is widely recognized as the premier tool for web browser automation, and with the introduction of Selenium 4 Alpha, developers are ushered into a new era of controls, features, and functionalities. This blog post delves into the key features of Selenium 4 and shines a light on potential pitfalls developers should be aware of when transitioning from Selenium 3 to Selenium 4.
What is Selenium?
Before diving deeply into the evolution of Selenium, let's cover the basics. Selenium is an open-source tool that enables you to automate web applications across various browsers. Developers primarily use it for testing purposes, but it can also be employed for web scraping and automating repetitive tasks.
Key Features of Selenium 4 Alpha
1. Enhanced WebDriver
One of the major improvements in Selenium 4 Alpha is the enhancement of the WebDriver API. The new API offers a more streamlined experience. Here's an example:
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.chrome.ChromeDriver;
public class WebDriverExample {
public static void main(String[] args) {
// Set the path for ChromeDriver
System.setProperty("webdriver.chrome.driver", "path/to/chromedriver");
// Initiate the WebDriver
WebDriver driver = new ChromeDriver();
// Launch the browser and navigate to a URL
driver.get("https://www.example.com");
// Interact with the web page
System.out.println("Title of the page is: " + driver.getTitle());
// Close the browser
driver.quit();
}
}
Why this matters: While in previous versions, setting up the environment could be cumbersome, Selenium 4 simplifies this through better handling of the WebDriver API. It increases productivity by reducing boilerplate code and minimizing initialization errors.
2. W3C Compliance and Improved Browsers Support
Selenium 4 is fully W3C compliant, meaning that it adheres to the standards set for web browser automation, which leads to better support across browsers. This marks a significant shift from previous versions that occasionally experienced compatibility issues.
3. New Features for Improved User Experience
Selenium 4 introduces several new features aimed at enhancing the user experience. These features include:
- Relative Locators: This allows you to locate elements relative to other elements on the page.
import org.openqa.selenium.By;
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.WebElement;
import org.openqa.selenium.chrome.ChromeDriver;
import org.openqa.selenium.support.locators.RelativeLocator;
public class RelativeLocatorExample {
public static void main(String[] args) {
System.setProperty("webdriver.chrome.driver", "path/to/chromedriver");
WebDriver driver = new ChromeDriver();
driver.get("https://www.example.com");
WebElement element = driver.findElement(RelativeLocator.with(By.tagName("input")).toRightOf(By.tagName("label")));
element.sendKeys("Input Text");
driver.quit();
}
}
Why this matters: Relative locators help improve the readability of your test scripts and reduce the need for expensive and brittle XPath or CSS selectors.
4. Improved Features for Modern Web Applications
Selenium 4 has added crucial improvements to handle modern web applications confidently by introducing:
-
Support for Selenium Grid: The Selenium Grid functionality is improved for better orchestration and management of tests across different environments.
-
DevTools Protocol: This offers capabilities directly linked into the browser for enhanced debugging and performance profiling.
5. Better Documentation and an Improved IDE
Selenium 4 provides improved documentation and a new integrated development environment (IDE). With a user-friendly guide, developers can easily refer to the features and best practices, reducing the learning curve.
Potential Pitfalls
As with any software upgrade, there are potential pitfalls associated with Selenium 4 that developers must navigate carefully.
1. Transitioning from Selenium 3
Transitioning from Selenium 3 could require significant changes in your test scripts. The commands may behave differently or may have been deprecated. Therefore, reviewing the release notes and migration guides is critical.
2. Compatibility Issues
While Selenium 4 boasts improved browser compatibility, some issues remain when dealing with older browsers or outdated WebDriver versions. Always ensure that the version you are using is up-to-date and compatible with your target browsers.
3. Early Adoption Challenges
As an alpha release, some features may not be fully polished. Bugs or incomplete functionalities may surface, leading to potential failures in automated tests. It is crucial to maintain a stable version of Selenium 3 alongside until you have thoroughly tested Selenium 4 in your environment.
4. Documentation Gaps
Despite the improvements in documentation, there may be areas where details are sparse. Contributing back to the community by reporting ambiguities or missing information can help improve future documentation.
Bringing It All Together
Overall, Selenium 4 Alpha presents exciting new features and improvements that are promising for the future of web automation. From enhanced usability and robust browser support to exciting new functionalities, there is much to look forward to. However, due diligence is necessary regarding the potential pitfalls, especially when migrating from an established framework like Selenium 3.
As always, staying informed and updated on the latest changes through Selenium's official documentation and community forums can greatly aid in a smooth transition to Selenium 4.
For further reading, you can check the official release details in the Selenium Github repository.
By understanding these features and precautions, developers can leverage the power of Selenium 4 to enhance their web automation experience effectively.