Common JSON Processing Pitfalls in GlassFish 4.0
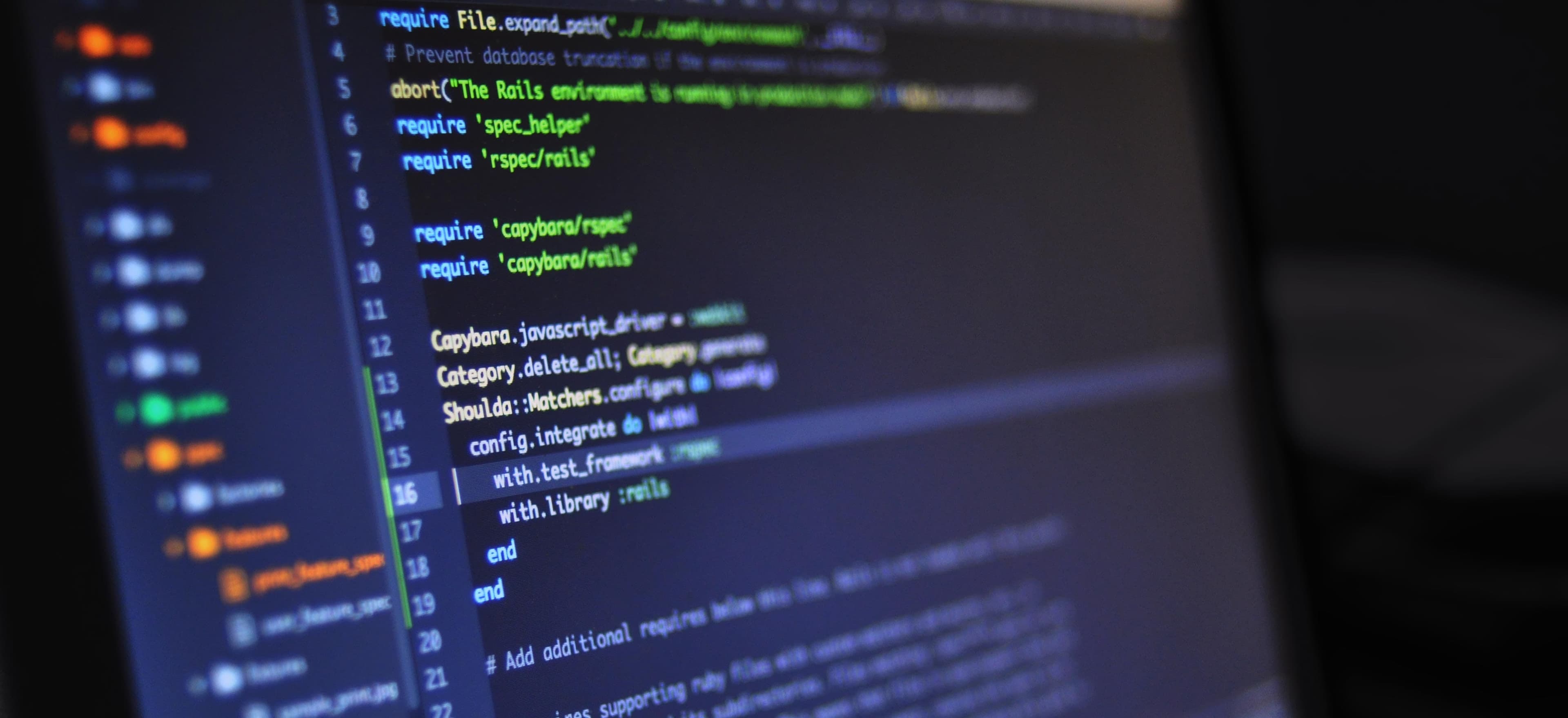
- Published on
Common JSON Processing Pitfalls in GlassFish 4.0
When working with Java web applications, particularly when using GlassFish 4.0 as your server, JSON processing is crucial. As JSON has become the de facto standard for data interchange over the web, understanding its implications in your Java applications is vital. However, developers often encounter various pitfalls when working with JSON in GlassFish. In this blog post, we will explore common JSON processing pitfalls, provide code examples, and discuss solutions to avoid these issues.
Understanding JSON in Java
Java provides numerous libraries and APIs for processing JSON data. One of the most popular APIs is Java API for JSON Processing (JSR 353), which makes it easy to handle JSON both in client and server-side applications. GlassFish 4.0 comes pre-packaged with a JSON processing API, simplifying data exchange.
Getting Started with JSON Processing
Before diving into pitfalls, let’s look at a basic example of JSON processing in Java using the javax.json package:
import javax.json.Json;
import javax.json.JsonObject;
public class JSONExample {
public static void main(String[] args) {
// Creating a JSON object
JsonObject jsonObject = Json.createObjectBuilder()
.add("name", "John Doe")
.add("age", 30)
.build();
// Printing the JSON object
System.out.println(jsonObject.toString());
}
}
Why This Example?
In this snippet, we create a JSON object with two key-value pairs: name
and age
. The Json.createObjectBuilder()
method is a fluent interface that allows chaining methods in a clean manner. JSON objects are printed as strings automatically, but note the importance of escaping special characters in real-world applications.
Common Pitfalls in JSON Processing
1. Improper Handling of Special Characters
When working with JSON, special characters such as quotes, backslashes, and control characters can cause issues during serialization and deserialization.
// Example of potential issues with special characters
String unsafeString = "This is a test string with a quote \" and a backslash \\";
JsonObject jsonObject = Json.createObjectBuilder()
.add("message", unsafeString)
.build();
System.out.println(jsonObject);
The Problem
In the above code, if unsafeString
contains unescaped characters, it may break the JSON structure.
Solution
Utilize correct escaping in your strings. Use libraries like Jackson or Gson that handle escapes automatically during serialization.
2. Incorrect Data Type Handling
JSON supports only a limited range of data types: strings, numbers, objects, arrays, booleans, and null.
// Example of mixing types improperly
JsonObject jsonObject = Json.createObjectBuilder()
.add("id", 123) // valid
.add("price", "20.5") // valid as string
.add("exists", null) // valid
.add("timestamp", new Date()) // NOT valid, will throw an error
.build();
The Problem
Attempting to add a Java object that isn’t supported by JSON will throw an exception. Date
objects need special handling.
Solution
Convert unsupported types to a valid JSON type. In this case, format Date objects as strings with a specified format.
import java.text.SimpleDateFormat;
import java.util.Date;
String formattedDate = new SimpleDateFormat("yyyy-MM-dd'T'HH:mm:ss").format(new Date());
JsonObject jsonObject = Json.createObjectBuilder()
.add("date", formattedDate)
.build();
3. Nesting JSON Objects
Nesting JSON objects can lead to complex structures that may be difficult to manage and deserialize properly.
JsonObject nestedJson = Json.createObjectBuilder()
.add("user", Json.createObjectBuilder()
.add("name", "Jane")
.add("age", 25)
.build())
.build();
System.out.println(nestedJson);
The Problem
While nesting provides a cleaner organization for related data, it can complicate serialization and deserialization. Handling deeply nested structures can become cumbersome and error-prone.
Solution
Flatten your JSON structure where possible. If nesting is essential, utilize libraries such as Jackson, which can map nested structures to Java objects easily.
4. Ignoring Error Handling
When processing JSON, it is crucial to implement robust error handling mechanisms to manage unexpected data formats or parsing errors.
import javax.json.Json;
import javax.json.JsonObject;
import javax.json.JsonReader;
import java.io.StringReader;
String jsonString = "{ \"name\": \"John Doe\", \"age\": thirty }"; // Invalid JSON
try (JsonReader reader = Json.createReader(new StringReader(jsonString))) {
JsonObject jsonObject = reader.readObject();
System.out.println(jsonObject.toString());
} catch (Exception e) {
System.err.println("Error parsing JSON: " + e.getMessage());
}
The Problem
Failing to include error handling will lead to runtime exceptions crashing your application.
Solution
Always wrap your JSON processing within try-catch blocks to handle exceptions gracefully. It provides insights into potential issues during parsing.
Testing JSON Data
Testing is essential in any software development life cycle. Use JUnit along with mock data to validate your JSON-related functionality.
import org.junit.jupiter.api.Test;
import javax.json.Json;
import static org.junit.jupiter.api.Assertions.*;
public class JSONTest {
@Test
public void testJsonCreation() {
String expected = "{\"name\":\"John Doe\",\"age\":30}";
String actual = Json.createObjectBuilder()
.add("name", "John Doe")
.add("age", 30)
.build()
.toString();
assertEquals(expected, actual);
}
}
Why Test?
Testing ensures that your JSON operations perform as expected, allowing you to manage the complexity of your application more effectively.
Lessons Learned
Understanding common pitfalls in JSON processing in GlassFish 4.0 is crucial for developers looking to create smooth and error-free applications. By paying attention to special characters, appropriate data types, nesting structures, error handling, and testing, you can significantly enhance your application's robustness.
For further reading, consider exploring The Java EE Tutorial for in-depth information on developing applications with Java EE technologies, including JSON processing.
Incorporate these best practices into your development workflow, and you'll be better equipped to handle JSON processing challenges in your GlassFish applications.
Feel free to comment below if you have any questions or would like further information on a specific topic!