Challenges of Integration Testing Scoped Beans in CDI
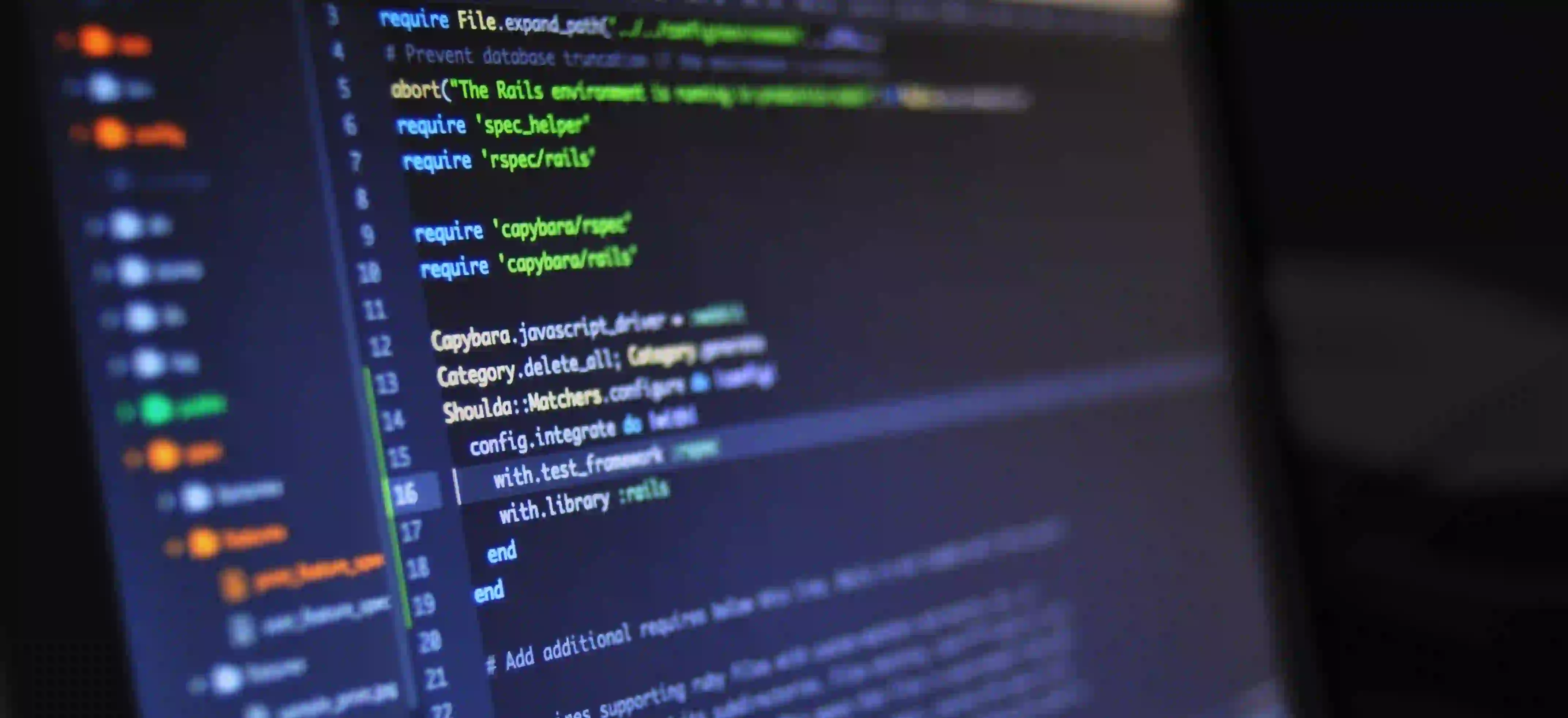
Challenges of Integration Testing Scoped Beans in CDI
Context and Dependency Injection (CDI) is a powerful feature of Java EE that helps manage the life cycle of beans and their dependencies in a systematic manner. While CDI simplifies development significantly, integration testing scoped beans can be particularly challenging. This blog post presents the common hurdles faced when conducting integration tests with scoped beans in CDI and offers insights into addressing these issues effectively.
Understanding Scoped Beans in CDI
Scoped beans in CDI are categorized by their lifecycle. They can have different scopes such as:
- Request Scope: A bean is created for each HTTP request.
- Session Scope: A bean exists during a user session.
- Application Scope: A single instance of a bean exists for the life of the application.
This scoping mechanism is crucial as it aligns beans with the lifecycle of an application, ensuring efficient resource management and enhancing modularity.
Why Is Integration Testing Important?
Integration testing checks how components work together. For scoped beans, it ensures that the desired behavior persists across various layers of the application, making sure all the scopes function as expected. However, due to the nature of scoped beans, testing them introduces a unique set of challenges.
Challenges in Integration Testing Scoped Beans
1. Context Configuration
Challenge: Setting up proper contexts for integration testing can be cumbersome. You need to ensure the CDI context is active and initialized correctly.
Solution: Leverage an embedded container for testing, such as Weld or OpenLiberty CDI. Here’s an example of how to initiate a CDI container using Weld:
import org.jboss.weld.junit4.embedded.WeldStandaloneJunit4;
import org.jboss.weld.environment.se.Weld;
import org.jboss.weld.environment.se.WeldContainer;
public class CDIIntegrationTest {
private WeldContainer container;
@Before
public void setUp() {
container = new Weld().initialize(); // Initialize the Weld container
}
@After
public void tearDown() {
container.shutdown(); // Properly shutdown the container
}
}
Why: This setup allows for a clean start of the CDI contexts, encapsulating the lifecycle of your beans, which is essential for accurate testing results.
2. Bean Lookup and Injection
Challenge: When testing with scoped beans, directly injecting beans in unit tests can yield unexpected results, especially if the context is not correctly instantiated.
Solution: Utilize @Inject
for injecting dependencies in your tests and ensure that the container is launched properly.
@Inject
private MyScopedBean myScopedBean;
@Test
public void testMyScopedBeanFunctionality() {
// Interact with myScopedBean
assertNotNull(myScopedBean); // Verify the bean is injected properly
// Further assertions and functionality tests...
}
Why: Injection using CDI allows test cases to remain declarative and concise, while making sure that the lifecycle of the beans is respected.
3. Testing Bean Scopes
Challenge: Testing scope-related behavior can be challenging especially as you transit between sessions or requests. For instance, does a session-scoped bean maintain its state across multiple interactions?
Solution: Use a testing framework to simulate different scopes. For example:
import javax.enterprise.context.RequestScoped;
@RequestScoped
public class RequestScopedBean {
private String value;
public String getValue() {
return value;
}
public void setValue(String value) {
this.value = value;
}
}
// In your test
@Test
public void testRequestScopedBean() {
RequestScopedBean bean = container.select(RequestScopedBean.class).get();
// Set initial value
bean.setValue("Hello, CDI");
// After simulating a request scope end, get a new bean instance
RequestScopedBean newBean = container.select(RequestScopedBean.class).get();
assertNull(newBean.getValue()); // Ensure value is not carried over
}
Why: By explicitly managing the lifecycle, it is easier to verify that scoped beans behave correctly in differing contexts.
4. Concurrent Context Issues
Challenge: Handling concurrent requests can lead to complications, especially with session-scoped beans where shared state may cause contention problems, leading to inconsistent test results.
Solution: Use synchronization techniques effectively or opt for thread-safe implementations when necessary:
@SessionScoped
public class ConcurrentSessionBean implements Serializable {
private String value;
public synchronized void setValue(String value) {
this.value = value;
}
public synchronized String getValue() {
return value;
}
}
Why: By explicitly adding synchronization, concurrent modifications can be managed, thus ensuring the tests have consistent results.
5. Dependency and Side Effects
Challenge: Scoped beans often depend on or interact with other beans, which can introduce side effects that muddy your test results.
Solution: Employ mocks for interacting beans and isolate the tests. Libraries like Mockito can be used effectively:
import static org.mockito.Mockito.*;
@Test
public void testScopedBeanWithMockDependency() {
// Create a mock for the dependent bean
DependentBean mockDependent = mock(DependentBean.class);
when(mockDependent.someMethod()).thenReturn("Mocked Value");
// Inject mocked dependency into the test bean
MyScopedBean myScopedBean = new MyScopedBean(mockDependent);
// Now test myScopedBean without relying on full implementations
assertEquals("Mocked Value", myScopedBean.callDependentMethod());
}
Why: By using mocks, it becomes simpler to focus on the behavior of the bean under test without external influences, helping maintain focus.
My Closing Thoughts on the Matter
Testing scoped beans in CDI brings both benefits and challenges. Understanding these challenges and employing proper solutions enables developers to enhance the reliability of their applications effectively.
For further reading on CDI and the best practices for testing, you can explore Jakarta EE Documentation and Weld Documentation.
As you delve deeper into CDI and Bean lifecycle management, you're standing on a solid foundation that will lead to more robust applications. Happy coding!