Debunking Garbage Collection Myths You Still Believe
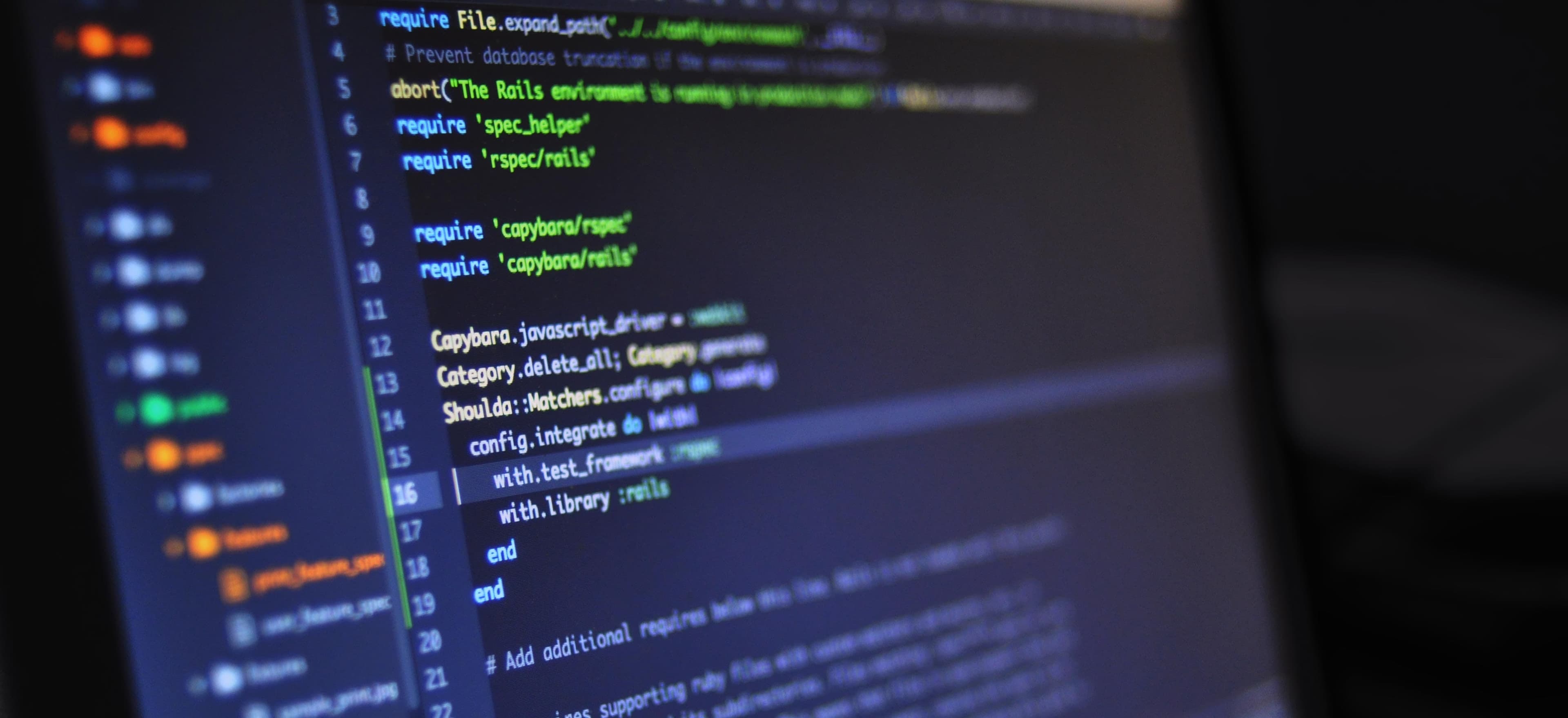
- Published on
Debunking Garbage Collection Myths You Still Believe
Garbage collection (GC) is an important topic in Java programming that often leads to misconceptions and myths. Understanding how it works can dramatically improve your application's performance, reduce memory leaks, and enhance your coding practices.
Are you ready to debunk some common garbage collection myths in Java? Let's dive in!
Myth 1: Garbage Collection Happens Automatically and Has No Overhead
The Reality
While it's true that Java's garbage collector runs automatically, it does not mean it comes without a cost. The process requires CPU cycles, which can introduce overhead that impacts application performance.
Garbage collection has two main types of overhead:
- Stop-the-world (STW) pauses: These are moments when the application halts to allow the GC process to clean up memory. Although stop-the-world events are generally brief, they can lead to noticeable delays, particularly in applications with high memory usage.
- Memory fragmentation: As objects are allocated and deallocated, the available memory may become fragmented, impacting performance.
Here’s a simple example of an object allocation:
public class DemoObject {
private String data;
public DemoObject(String data) {
this.data = data;
}
}
// Object creation which triggers GC at some point
public static void main(String[] args) {
for (int i = 0; i < 10000; i++) {
new DemoObject("Data " + i); // The GC will clean up eventually
}
}
In this example, each time we create an instance of DemoObject
, it occupies memory. Over time, many of these instances will become unreachable, triggering garbage collection. However, be aware that memory management comes with its performance costs.
Takeaway
Garbage collection is automatic, but it certainly involves overhead. Understanding its trade-offs can help developers design better applications.
Myth 2: Garbage Collection Guarantees Memory is Freed Immediately
The Reality
It's a common myth that once an object becomes unreachable, the memory it occupies is immediately freed by the garbage collector. However, this is not how Java's garbage collection functions.
When an object becomes unreachable, it is marked for garbage collection. However, actual memory reclamation may occur later, depending on various factors, including the garbage collector's algorithms and the current state of the JVM.
For instance, the following code creates a large object in memory:
public static void main(String[] args) {
int[] largeArray = new int[1000000];
// Do something with largeArray
largeArray = null; // The object now eligible for GC
}
In this scenario, setting largeArray
to null
means the JVM can reclaim that memory, but the actual memory recovery is not guaranteed to happen immediately. The garbage collector might delay this for efficiency, especially if more allocations are forthcoming.
Takeaway
Garbage collection does not guarantee immediate memory reclamation. Understanding this helps set realistic expectations for memory management in Java.
Myth 3: The More Objects You Create, the More Frequent GC Runs
The Reality
Actually, the frequency of garbage collection depends less on the number of objects created and more on the overall heap usage. The JVM employs sophisticated algorithms that determine when to trigger garbage collection based on its memory usage patterns.
Java has different garbage collection algorithms (like Serial, Parallel, CMS, G1, and ZGC) that optimize memory management to minimize GC frequency while maximizing application throughput.
Here’s an example of objects created in a loop:
public static void main(String[] args) {
for (int i = 0; i < 100000; i++) {
new DemoObject("Object " + i);
}
Runtime.getRuntime().gc(); // Hinting JVM to perform GC
}
The above snippet suggests a request for garbage collection (Runtime.getRuntime().gc()
), but this doesn't call for immediate action or guarantee that GC will run. The JVM can choose to ignore this request based on its internal algorithms and the current state.
Takeaway
Garbage collector frequency is contingent on overall heap usage, not merely the number of created objects. Awareness helps you write more efficient code.
Myth 4: Finalizers Are Always Necessary for Cleanup
The Reality
While it's true that finalizers have been part of Java's garbage collection mechanism, relying on them is not the best practice. Finalizers often lead to unpredictable behavior, such as delayed resource release and poor application performance.
Java 9 introduced the method java.lang.ref.Cleaner
, which is a much more reliable and efficient way of managing resource cleanup. Using a cleaner allows you to execute code upon object reaching a certain state without the overhead and unpredictability of finalizers.
Example
Here’s an example comparing finalizers and cleaner:
// Using finalizers (discouraged)
class FinalizerExample {
protected void finalize() {
// Do resource cleanup
}
}
// Using Cleaner (recommended)
import java.lang.ref.Cleaner;
class CleanerExample implements Runnable {
static final Cleaner cleaner = Cleaner.create();
final Cleaner.Cleanable cleanable;
CleanerExample() {
cleanable = cleaner.register(this, this);
}
public void run() {
// Cleanup code here
}
}
Takeaway
Avoid using finalizers in favor of cleaner or other modern resource management techniques. It leads to cleaner and more predictable code.
Myth 5: You Should Always Tune Your GC Settings
The Reality
While it's important to be aware of JVM parameters that affect garbage collection, tuning is not always necessary or beneficial. If your application is running smoothly and memory usage is within expected bounds, excessive parameter tuning can lead to unnecessary complexity and can even degrade performance.
However, if you're facing performance issues related to memory consumption, it may be worth investigating available garbage collection options in your specific case.
Example
Here's how you might adjust the memory settings for your JVM:
java -Xms512m -Xmx2048m -XX:+UseG1GC -jar myapp.jar
-Xms512m
: Initial memory allocation.-Xmx2048m
: Maximum memory allocation.-XX:+UseG1GC
: Enable G1GC, an often efficient garbage collector.
Takeaway
Tuning garbage collection settings should be a measured decision, not a blanket approach. Focus on monitoring your application's performance before making these adjustments.
Final Thoughts
Understanding the ins and outs of garbage collection in Java saves you time and frustration in your coding journey. By debunking these common myths - from the automatic nature of GC to the need for finalizers - you can write cleaner, more efficient code.
Remember that while garbage collection simplifies memory management, it is essential to understand its nuances. Leveraging proper resource management practices and staying informed about how garbage collection operates will only enhance your Java programming skills.
For a deeper understanding, check out the official Java documentation on garbage collection and explore various garbage collection algorithms to see which might fit your application's needs.
Happy coding!
Checkout our other articles