Avoiding Common Pitfalls with Hazelcast MapLoader
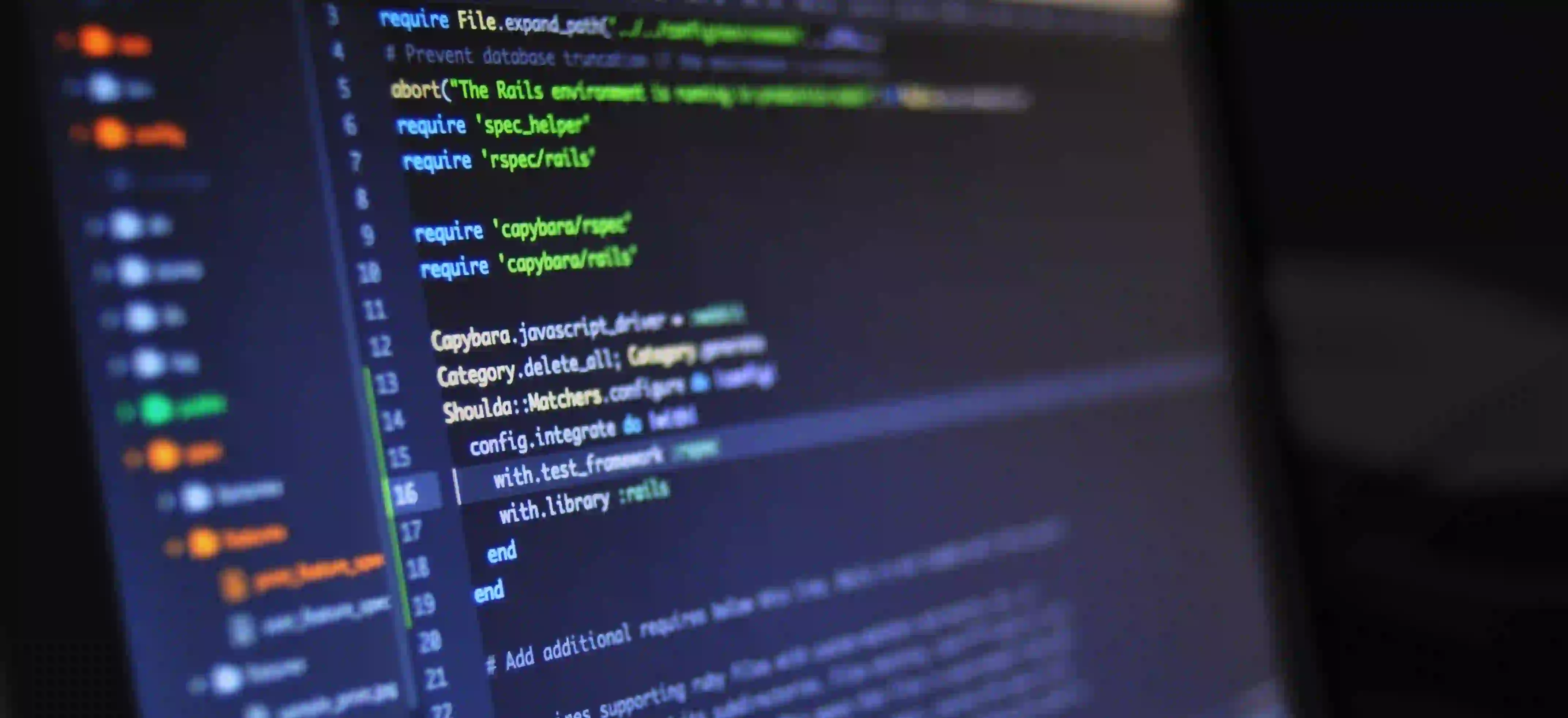
Avoiding Common Pitfalls with Hazelcast MapLoader
Hazelcast is a powerful in-memory data grid that allows developers to scale their applications and provide fast data access. One of its key features is the MapLoader
, which allows you to load data from an external store, such as a database, into the Hazelcast distributed map. However, like any powerful tool, there are common pitfalls developers may encounter when using Hazelcast's MapLoader
. This article will guide you through these pitfalls and provide strategies to avoid them, ensuring you can utilize MapLoader
effectively in your applications.
What is a MapLoader?
Before we dive into pitfalls, let's define what a MapLoader
is in the context of Hazelcast. A MapLoader
allows you to provide an implementation that can load entries from an external source, ensuring that the data in a Hazelcast map is always up-to-date.
When you implement the IMap
interface along with a MapLoader
, you enable your map to load data lazily or on-demand. This means that if your data set is vast and not all entries are regularly accessed, the MapLoader
can retrieve the necessary data only when required.
Common Pitfalls of Using MapLoader
Here are some common pitfalls developers face when using MapLoader
, as well as best practices to avoid them.
1. Not Implementing Proper Caching Logic
Issue: One of the most common mistakes is failing to implement caching logic correctly. If your MapLoader
fetches data every time it is needed without considering if the data is already cached, it will severely affect performance.
Solution: Implement caching within your MapLoader
. Use a proper caching strategy (like TTL – Time to Live) to manage the lifecycle of your data. This will reduce the number of costly database calls.
Example:
public class MyMapLoader implements MapLoader<Long, MyEntity> {
private final Cache<Long, MyEntity> localCache = new ConcurrentHashMap<>();
@Override
public MyEntity load(Long key) {
// Check the local cache first
if (localCache.containsKey(key)) {
return localCache.get(key);
}
// Otherwise, fetch from the database
MyEntity entity = fetchFromDatabase(key);
// Cache the result for future requests
localCache.put(key, entity);
return entity;
}
private MyEntity fetchFromDatabase(Long key){
// Database fetching logic here.
}
}
In this example, before reaching out to the database, the code first checks if the data already exists in the local cache, significantly improving performance.
2. Ignoring Exception Handling
Issue: Network issues, data corruption, or connection timeouts can occur, leading to exceptions when loading data. Failing to handle these properly may lead to application crashes or data inconsistency.
Solution: Implement robust exception handling within your MapLoader
. This means not just catching exceptions but also logging them for further analysis and implementing fallback mechanisms.
Example:
@Override
public MyEntity load(Long key) {
try {
return fetchFromDatabase(key);
} catch (SQLException e) {
// Log the SQLException
Logger.error("Failed to load entry with key: " + key, e);
// Return null or a default value
return null;
}
}
By logging exceptions, you can quickly identify issues in your data retrieval process, allowing for more manageable debug sessions.
3. Not Synchronizing State Across Nodes
Issue: In a distributed system, data consistency is crucial. If one node updates its data and other nodes don’t recognize that update, this can lead to stale data scenarios.
Solution: Ensure that the MapLoader
implementation considers synchronization. You might use Hazelcast's built-in mechanisms, such as EntryListener
, to keep data in sync across nodes.
Example:
public class MyMapStore implements MapStore<Long, MyEntity> {
@Override
public void store(Long key, MyEntity value) {
// Store into the database
saveToDatabase(key, value);
// Notify other nodes to refresh their cache or state
notifyAllNodes(key);
}
private void notifyAllNodes(Long key) {
// Publish an event to notify other nodes
}
}
4. Neglecting Performance Testing
Issue: Changes made to the data retrieval strategies or scaling the data set without testing can lead to unpredicted performance degradation.
Solution: Always perform load testing and measure the performance of your MapLoader
. Use tools like JMeter or Gatling to simulate several concurrent requests and monitor how your Hazelcast cluster responds.
# Load test command example
mvn gatling:test
5. Mismanaging Thread Safety
Issue: When multiple threads access shared data without synchronization, it can cause inconsistent or corrupt data. This is a critical concern with mutable objects.
Solution: Always ensure that your MapLoader
implementations use thread-safe collections or proper synchronization mechanisms.
Example:
private final Map<Long, MyEntity> synchronizedMap = Collections.synchronizedMap(new HashMap<>());
@Override
public MyEntity load(Long key) {
synchronized(synchronizedMap) {
return synchronizedMap.get(key);
}
}
Closing Remarks
Hazelcast's MapLoader
can significantly enhance the performance and scalability of your application when implemented correctly. By preventing common pitfalls such as improper caching, inadequate exception handling, lack of data synchronization, poor performance testing, and thread safety issues, developers can maximize the benefits of using Hazelcast.
Understanding these challenges will bolster your ability to create a robust application. As you delve deeper into Hazelcast and its features, consider checking out the Hazelcast documentation and the Hazelcast GitHub repository for advanced functionalities and practical examples.
By adhering to best practices and paying careful attention to the implementation of MapLoader
, you will ensure that your applications utilize Hazelcast's capabilities efficiently and safely, paving the way for high-performance, reliable systems.