Common Pitfalls When Starting with Spring MVC Test Framework
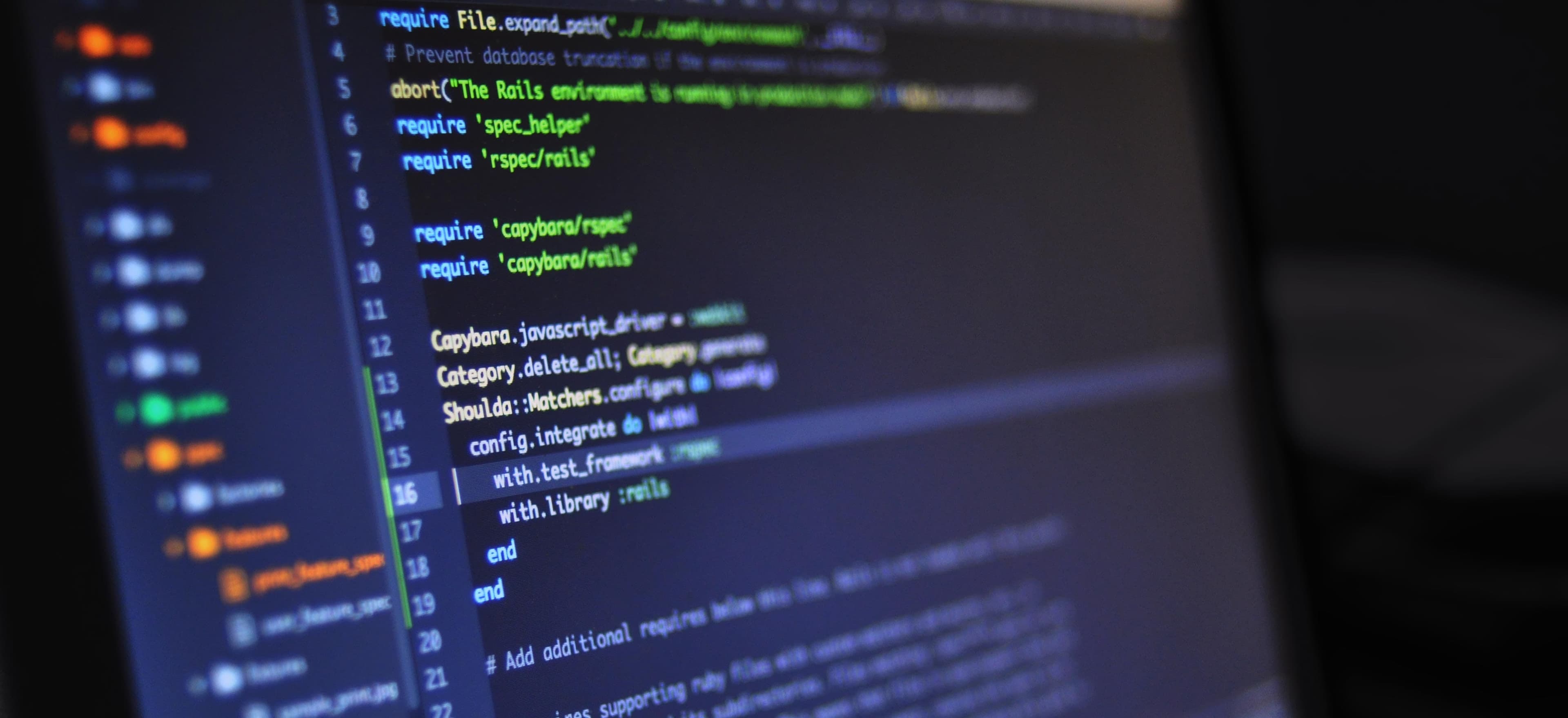
- Published on
Common Pitfalls When Starting with Spring MVC Test Framework
The Spring Framework has established itself as a go-to for building enterprise Java applications. When it comes to testing Spring MVC applications, the Spring MVC Test framework offers powerful capabilities. However, newcomers often encounter pitfalls that can lead to frustration. In this post, we will explore those common pitfalls while providing tips and code snippets to help you avoid them.
What is Spring MVC Test Framework?
The Spring MVC Test framework is part of the Spring Test module, designed for testing Spring MVC applications in an isolated environment. It allows developers to perform integration testing of web controllers without the need for deploying a full web server, making unit tests easier and faster.
Common Pitfalls
1. Underestimating the Importance of Context Configuration
One of the most common mistakes beginners make is neglecting the context configuration. The Spring context is a pivotal part of the application, and if it is not set up correctly, your tests can fail or behave unpredictably.
@RunWith(SpringJUnit4ClassRunner.class)
@ContextConfiguration(classes = {WebConfig.class})
public class MyControllerTest {
// Test cases go here
}
Why This Is Important:
The @ContextConfiguration
annotation helps to load the configuration context for the test. Failing to provide the correct context can lead to NullPointerExceptions
or misconfigured beans, making it critical to ensure your context configurations match those used in your application.
2. Misunderstanding Mocking and Stubbing
Another common pitfall is not taking advantage of mocking. Many developers try to use real services instead of mocks when testing controller logic, leading to slow and flaky tests.
@MockBean
private MyService myService;
@Test
public void testGetUser() throws Exception {
User user = new User(...);
when(myService.findUserById(1L)).thenReturn(user);
mockMvc.perform(get("/users/1"))
.andExpect(status().isOk())
.andExpect(jsonPath("$.name", is("John Doe")));
}
Why This Is Important:
By using @MockBean
, you can isolate the controller by replacing real service calls with mocks. This leads to faster executions and isolates the test to focus only on your controller, resulting in more reliable tests.
3. Ignoring Request Builder Options
Sometimes beginners overlook the versatility of the MockMvcRequestBuilders
. It has a rich set of features to build requests, and failing to utilize these can lead to more complicated tests.
mockMvc.perform(post("/users")
.contentType(MediaType.APPLICATION_JSON)
.content("{ \"name\": \"John\", \"age\": 30 }"))
.andExpect(status().isCreated());
Why This Is Important:
Utilizing the contentType
and content
methods properly ensures that you are simulating a real-world request accurately. This leads to better validation of your controllers' logic.
4. Lack of Proper Assertions
New testers sometimes use insufficient or vague assertions in their tests, leading to less comprehensive validation. It's crucial to assert not just the HTTP status but also the expected content of the response.
mockMvc.perform(get("/users/1"))
.andExpect(status().isOk())
.andExpect(content().contentType(MediaType.APPLICATION_JSON))
.andExpect(jsonPath("$.name", is("John Doe")))
.andExpect(jsonPath("$.age", is(30)));
Why This Is Important:
By using detailed assertions, you verify not just the success of the request but also that the returned data is correct. This will prevent regression in future updates.
5. Overlooking Exception Handling Tests
Failing to test how your application handles errors can lead to severe issues in production. Ensuring that your controllers return proper error messages and status codes is critical.
@Test
public void testGetUserNotFound() throws Exception {
when(myService.findUserById(1L)).thenThrow(new UserNotFoundException());
mockMvc.perform(get("/users/1"))
.andExpect(status().isNotFound())
.andExpect(content().string("User not found."));
}
Why This Is Important:
Testing for exceptions ensures that your application can handle edge cases gracefully, providing the correct feedback to users and maintaining system stability.
6. Failing to Utilize Test Slicing
While it’s good practice to test the entire application, newcomers often make the mistake of testing the entire stack when all they need is to test the web layer. @WebMvcTest
is a handy annotation for this.
@WebMvcTest(MyController.class)
public class MyControllerTest {
// Setup and test cases go here
}
Why This Is Important:
By using @WebMvcTest
, you can focus only on the web layer without loading other beans, allowing for faster unit tests and reducing complexity.
7. Forgetting to Clean Up Test Data
It’s not uncommon to lose track of test data created during tests, leading to flaky tests if they rely on stale data. Utilize the @After
annotation to clean up.
@After
public void tearDown() {
myService.clearTestData();
}
Why This Is Important:
Cleaning up ensures that each test runs with a clean slate. It prevents tests from affecting each other, which can cause unexpected results.
Final Thoughts
Starting with the Spring MVC Test Framework can be challenging, but awareness of these common pitfalls can streamline your journey. As applications become more complex, the importance of comprehensive testing cannot be overstated. The Spring MVC Test framework provides a powerful toolkit, and leveraging it effectively can lead to robust and efficient applications.
For more detailed information, you can check the official Spring documentation on testing and explore various examples. Happy testing!