Taming OutOfMemoryError in Chaos Engineering Tests
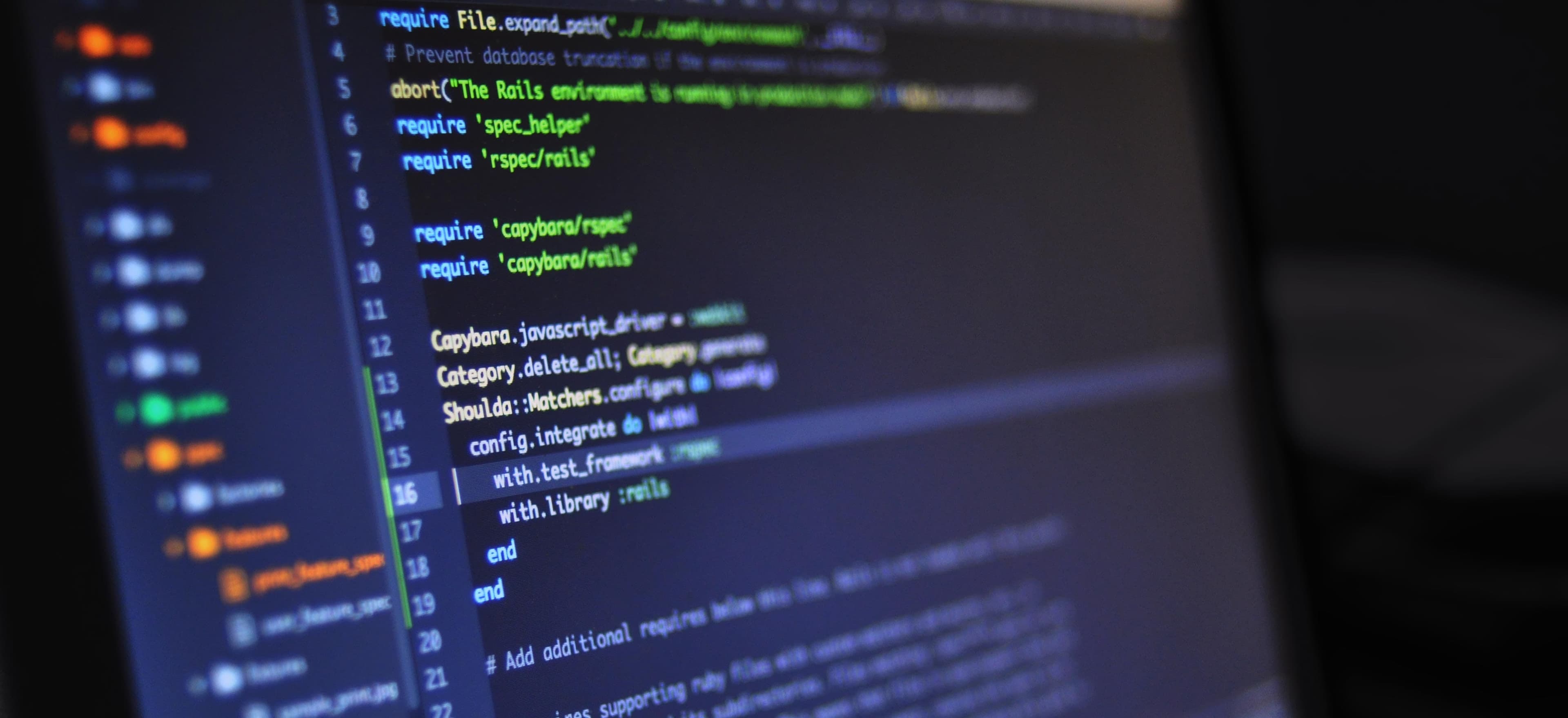
- Published on
Taming OutOfMemoryError in Chaos Engineering Tests
In today’s rapidly evolving software landscape, chaos engineering emerges as a crucial instrument for enhancing system resilience. It involves intentionally injecting faults into a system to identify weaknesses before they manifest in a production environment. However, such practices can inadvertently lead to OutOfMemoryError, a common yet critical issue. In this blog post, we will explore how to manage and prevent OutOfMemoryError during chaos engineering tests, arming you with the knowledge to conduct more effective and sustainable tests.
What is OutOfMemoryError?
Before we dive deeper, it's essential to understand what OutOfMemoryError is. In Java, it occurs when the Java Virtual Machine (JVM) cannot allocate an object because it is out of memory. This can happen for various reasons:
- The heap size is too small for the application's memory needs.
- Memory leaks can lead to the gradual consumption of all available memory.
- Rogue processes or unexpected workloads during chaos tests.
Understanding this error is a prerequisite to taming it.
Embracing Chaos Engineering
Chaos engineering is about risking it all to build resilient systems. As described in The Principles of Chaos Engineering, the fundamental philosophy is:
- Create a Hypothesis: Assume that a particular aspect of the system will fail.
- Run Experiments in Production: Inject the failure in a controlled manner.
- Observe and Measure Impacts: Use monitoring tools to track system behavior.
- Learn from the Results: Adapt and improve based on observations.
However, if your tests lead to OutOfMemoryError, the learnings can become overshadowed by system crashes. That is where our focus lies.
Why OutOfMemoryError Happens During Chaos Tests
During chaos engineering tests, systems are subjected to environments they wouldn’t typically encounter. Here are several reasons why OutOfMemoryError can surface:
- Increased Load: Chaos tests often simulate a higher user load or more data transactions than usual, pushing memory limits.
- Unmanaged Resource Allocation: Test scenarios can inadvertently lead to excessive object creation without proper management.
- Memory Leaks: Services might retain references to objects longer than needed, preventing garbage collection.
To combat this, we can implement several strategies.
Strategies for Managing OutOfMemoryError
1. Optimize JVM Settings
The JVM settings can significantly influence memory usage. Configuring the heap memory is crucial to establish a baseline that fits your application's needs.
java -Xms512m -Xmx2g -jar yourapplication.jar
- -Xms: Sets the initial heap size.
- -Xmx: Defines the maximum heap size.
Why? Allocating proper initial and maximum heap sizes reduces the chance of memory errors due to sudden load spikes.
2. Implement Efficient Memory Management
Proper memory management involves:
- Object Pooling: Reuse objects instead of creating new ones. For example, if you have a heavy data structure:
public class ObjectPool {
private List<SomeObject> available = new ArrayList<>();
private List<SomeObject> inUse = new ArrayList<>();
public SomeObject acquire() {
if (!available.isEmpty()) {
SomeObject obj = available.remove(available.size() - 1);
inUse.add(obj);
return obj;
}
return new SomeObject(); // create new instance if pool is empty
}
public void release(SomeObject obj) {
inUse.remove(obj);
available.add(obj);
}
}
Why? By reusing objects, you minimize new instances, reducing memory consumption.
3. Monitor and Diagnose Memory Usage
Regularly monitoring memory can give insights into potential issues before they escalate. Integrate tools like:
- VisualVM: A visual tool for monitoring and analyzing Java applications.
- JConsole: A monitoring tool that provides information about performance and resource consumption.
Use the following code snippet to visualize memory usage:
import java.lang.management.ManagementFactory;
import java.lang.management.MemoryMXBean;
public class MonitorMemory {
public static void main(String[] args) {
MemoryMXBean memoryMXBean = ManagementFactory.getMemoryMXBean();
System.out.println("Heap Memory Usage: " + memoryMXBean.getHeapMemoryUsage());
System.out.println("Non-Heap Memory Usage: " + memoryMXBean.getNonHeapMemoryUsage());
}
}
Why? Monitoring memory can help identify memory leaks and heavy usage patterns.
4. Implement Proper Garbage Collection Strategies
Understand the garbage collection (GC) process. Tuning it can prevent memory issues. You can leverage different garbage collection algorithms based on your application's needs:
- G1 Garbage Collector for low-latency applications.
- CMS for applications requiring concurrent collection.
Specify GC parameters in your startup command:
java -XX:+UseG1GC -jar yourapplication.jar
Why? Tailoring the garbage collection process can improve performance and reduce memory errors.
5. Conduct Controlled Tests Gradually
Instead of bombarding your system with a massive load immediately, explore a gradual testing approach. Start small and increase the complexity stage by stage, coupled with monitoring to catch any emerging memory issues early on.
Why? This approach allows you to gauge the system's limits without overwhelming it.
Reflecting on Chaos Engineering Outcomes
Chaos testing should not be an experience marred by crashes and errors. Instead, it should lead to invaluable insights that empower teams to make their systems robust. By combining vigilant monitoring, efficient coding practices, and smart JVM configurations, you can minimize the risk of encountering OutOfMemoryError during chaos tests.
Final Thoughts
As organizations weave chaos engineering into their development lifecycles, understanding and managing memory utilization becomes paramount. OutOfMemoryError, while common, need not be a painful roadblock. With the discussed strategies, you can fortify your system against the unforeseen challenges that arise during chaos tests, transforming potential doom into a learning experience.
Let's embrace the chaos while preparing to conquer it effectively!
Additional Resources
- The Twelve-Factor App
- Java Garbage Collection Basics
- Monitoring Java Applications
By implementing the outlined strategies, you can not only enhance your resilience but also set a positive precedent for future chaos engineering tests. Happy coding!