Finding the Perfect Balance in Goldilocks Microservices
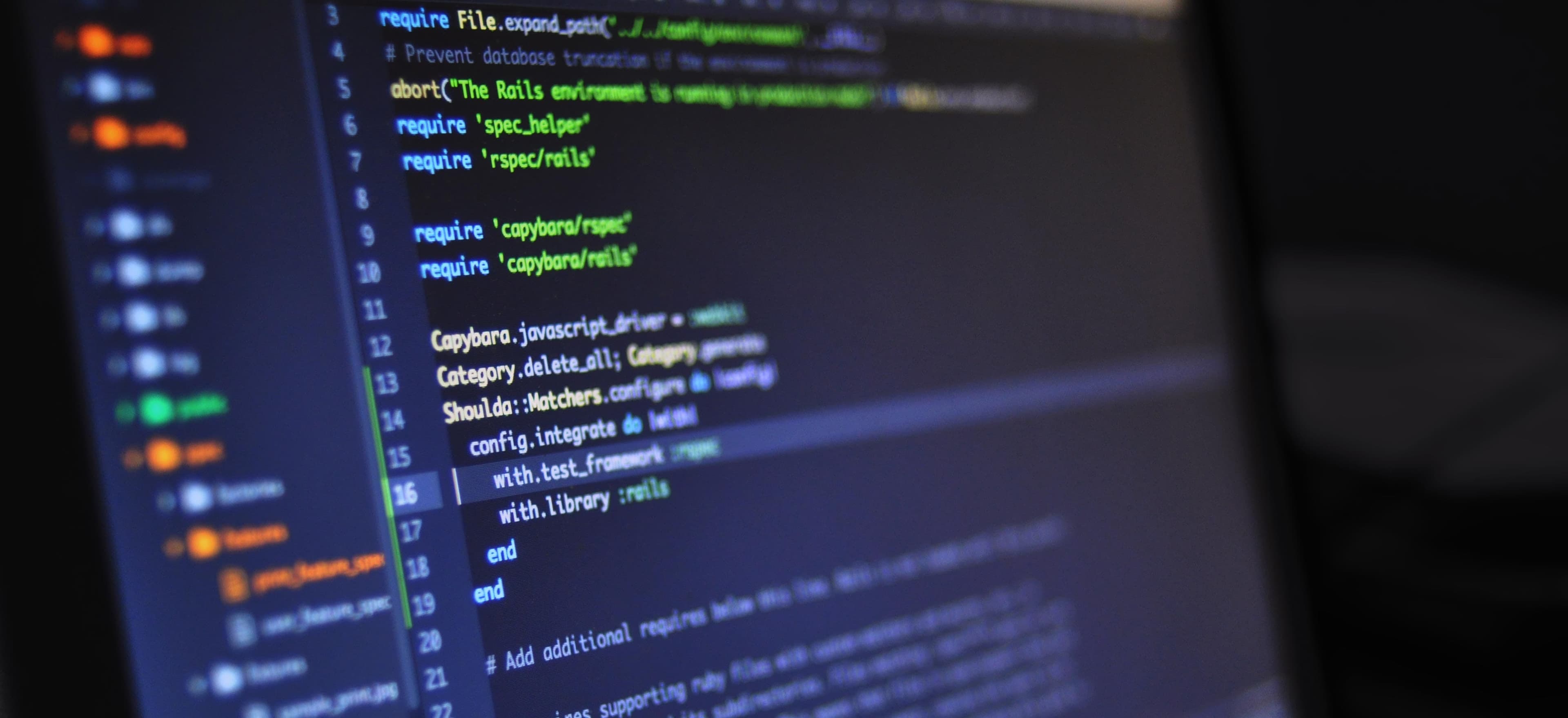
- Published on
Finding the Perfect Balance in Goldilocks Microservices
In the realm of software architecture, microservices have emerged as a popular approach to building scalable and resilient applications. However, managing these services effectively can pose its own unique set of challenges. Enter the concept of "Goldilocks Microservices" — striking the perfect balance between too few and too many services. In this blog post, we will explore best practices for identifying an optimum microservices architecture and implementing it effectively in Java applications.
Understanding Microservices
What Are Microservices?
Microservices are architectural styles that decompose applications into small, independent services that communicate over APIs. Each service serves a specific business function and can be developed, deployed, and scaled independently.
This separation allows for greater flexibility, agility, and the ability to adopt new technologies without affecting the entire system. For instance, one microservice could be written in Java while another is in Python, offering teams the flexibility to choose the best tools for the task at hand.
Why "Goldilocks"?
The name "Goldilocks" refers to the idea of finding an optimal state - not too hot, not too cold, but just right. In microservices, this translates to finding the right number of services. Having too many can lead to complexity and overhead, while too few can cause the application to become monolithic, losing the benefits of microservices.
Striking the Right Balance
Achieving the perfect balance requires a combination of several principles, which we will explore in this article. We'll focus on:
- Domain-Driven Design (DDD)
- Independent Deployment
- Resilience and Fault Tolerance
- Data Management in Microservices
Domain-Driven Design (DDD)
What is DDD?
Domain-Driven Design is a concept that helps you align your software design with the business domain it serves. By understanding the domain, you can identify the boundaries of your microservices.
How to Apply DDD in Microservices
Start by engaging stakeholders to define the core business functions. This can be done through techniques like Event Storming or using the ubiquitous language approach.
Here’s an example of how to identify service boundaries using Java:
// Example of an Order Service
public class Order {
private String id;
private String productId;
private int quantity;
// Constructors, Getters, and Setters omitted for brevity
}
public class OrderService {
public Order createOrder(String productId, int quantity) {
Order order = new Order(UUID.randomUUID().toString(), productId, quantity);
// Save the order to the database
return order;
}
}
Commentary
In this simple service, we represent an Order
entity. The OrderService
can be independently scaled or deployed, allowing for rapid iteration on order processing. This design follows DDD principles by focusing on a single, well-defined responsibility.
Independent Deployment
One of the beauties of microservices is the ability to deploy services independently. This reduces the complexity that comes with deploying a monolithic application.
How to Achieve Independent Deployment
Using tools like Docker and Kubernetes, you can manage microservices in isolated containers. This supports rolling updates and reduces downtime.
Here’s a simple Dockerfile for a Java microservice:
# Dockerfile for Spring Boot application
FROM openjdk:11-jdk-slim
VOLUME /tmp
COPY target/myapp.jar myapp.jar
ENTRYPOINT ["java", "-jar", "/myapp.jar"]
Commentary
Notice how the Dockerfile specifies the base image as OpenJDK 11. It then copies the built .jar
file into the container, which simplifies deployment and ensures consistency across environments.
Resilience and Fault Tolerance
The Importance of Resilience
In a microservices architecture, a failure in one service shouldn't bring down the entire application. Implementing resilience patterns, such as Circuit Breakers, can help mitigate this risk.
Implementing Circuit Breaker in Java
To manage service failures effectively, we can leverage the Resilience4j library in our Java microservices. Here's an example of a service that uses a circuit breaker:
import io.github.resilience4j.circuitbreaker.CircuitBreaker;
import io.github.resilience4j.circuitbreaker.CircuitBreakerConfig;
public class PaymentService {
private CircuitBreaker circuitBreaker;
public PaymentService() {
CircuitBreakerConfig config = CircuitBreakerConfig.custom()
.failureRateThreshold(50)
.waitDurationInOpenState(Duration.ofMillis(1000))
.build();
this.circuitBreaker = CircuitBreaker.of("paymentService", config);
}
public String processPayment(String orderId) {
return circuitBreaker.executeSupplier(() -> {
// Simulate payment processing
return "Payment processed for order " + orderId;
});
}
}
Commentary
In the above code, we configure a circuit breaker for the processPayment
method, which helps safeguard against failures (e.g., payment gateway service outages). This approach promotes resilience, allowing other services to continue functioning even if the payment service fails temporarily.
Data Management in Microservices
The Challenge of Data Management
One of the critical challenges in microservices is database management. Each service should ideally own its data. However, this leads to potential challenges regarding data consistency and transaction management.
Approaches to Data Management
-
Database per service: Each microservice manages its own database, ensuring loose coupling.
-
Event sourcing: By capturing changes in the application state as a sequence of events, you can maintain data consistency across services.
-
Saga Pattern: It manages complex business transactions across multiple services, allowing compensation actions if a step fails.
Example: Using Events for Data Management
Here’s an illustration of a simple event publishing mechanism in Java using Spring Cloud Stream:
@EnableBinding(Source.class)
public class OrderEventPublisher {
private final Source source;
public OrderEventPublisher(Source source) {
this.source = source;
}
public void publishOrderCreatedEvent(Order order) {
source.output().send(MessageBuilder.withPayload(order).build());
}
}
Commentary
In this example, the OrderEventPublisher
publishes an Order
event to a message broker like Kafka. Other services can subscribe to these events, promoting decoupling and allowing services to evolve independently.
Final Considerations
Finding the perfect balance in microservices architecture is akin to the Goldilocks principle. By applying Domain-Driven Design, ensuring independent deployment, implementing resilience patterns, and managing data efficiently, we can achieve an optimal microservices strategy that is not too complex or simplistic, but just right.
For further reading on microservices, consider exploring Martin Fowler's article on Microservices or visit Spring.io for microservices.
Investing time to fine-tune your microservices strategy will lead to long-term benefits, including improved scalability and agility, ultimately driving business success. Remember, finding the perfect balance is a continual process of reflection and adjustment, mirroring both the nature of software development and your organization's unique needs. Happy coding!