Overcoming Continuous Deployment Challenges in DevOps
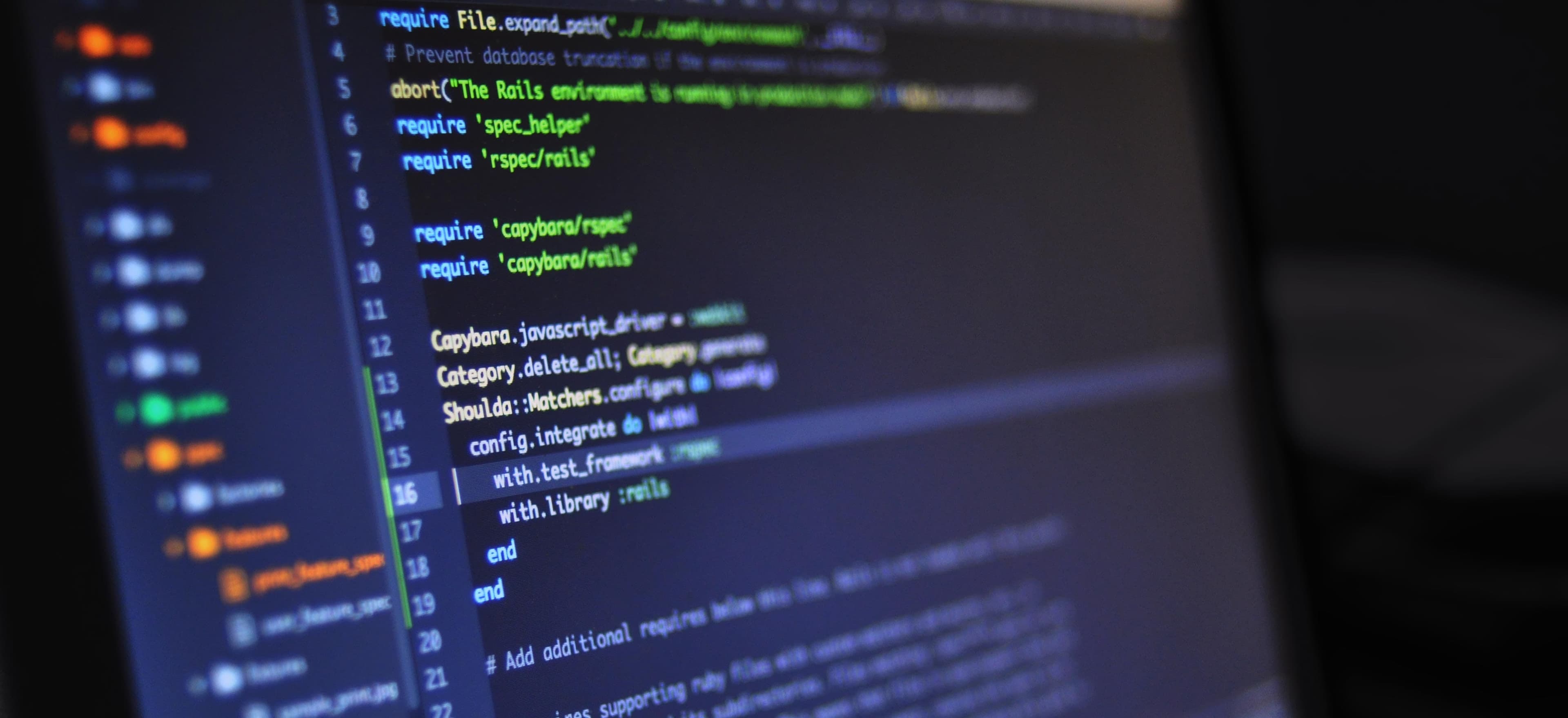
- Published on
Overcoming Continuous Deployment Challenges in DevOps
Continuous Deployment (CD) is a critical component of the DevOps methodology that enables development teams to automatically release their code changes into production with minimal manual intervention. While the benefits of CD are significant—including faster time to market, improved quality of releases, and enhanced team collaboration—it also comes with its unique set of challenges. In this blog post, we'll explore common obstacles to successful continuous deployment and present practical solutions to overcome them.
Understanding Continuous Deployment
Before diving into challenges, it's important to understand what continuous deployment entails. Continuous deployment is the practice of automatically deploying code to production as soon as it passes automated tests. This approach allows organizations to capture feedback quickly, adapt to changes, and remain competitive in today’s fast-paced software landscape.
Key Benefits of Continuous Deployment
- Faster Release Cycles: With automated deployment, updates are released frequently, reducing the time between writing code and delivering it to users.
- Immediate Feedback Loop: Continuous deployment allows developers to receive immediate feedback from end-users, enhancing product quality.
- Reduced Risk: Smaller, more frequent releases mitigate the risk associated with deploying large sets of changes at once.
Common Challenges in Continuous Deployment
As organizations adopt continuous deployment, they may encounter the following challenges:
1. Automation Complexity
Automation is at the heart of continuous deployment. However, the complexity involved in setting up automated pipelines can be daunting. It requires proper infrastructure, tools, and expertise.
Solution: Begin with a phased approach. Start by automating the simplest parts of your deployment pipeline and expand gradually:
# Example of a simple CI/CD pipeline configuration using GitHub Actions
name: CI/CD Pipeline
on:
push:
branches:
- main
jobs:
build:
runs-on: ubuntu-latest
steps:
- name: Checkout code
uses: actions/checkout@v2
- name: Set up JDK
uses: actions/setup-java@v2
with:
java-version: '11'
- name: Build with Maven
run: mvn clean install
- name: Run Tests
run: mvn test
- name: Deploy
run: ./deploy.sh
In this YAML configuration, we define a basic pipeline using GitHub Actions that triggers on a push to the main branch. This simplicity allows teams to focus on core functionalities before adding complexity.
2. Handling Legacy Systems
Many organizations work with legacy systems that were not built with automation in mind. Integrating CD with such systems can be challenging and may require significant refactoring.
Solution: Conduct a thorough analysis of the legacy systems to understand their limitations. Incrementally refactor components and apply microservices architecture where feasible to facilitate smoother deployments.
3. Testing Challenges
Reliable tests are essential for a successful continuous deployment strategy. Without thorough automated testing, the risk of deploying faulty code increases substantially.
Solution: Implement a robust testing strategy that includes unit tests, integration tests, and end-to-end tests. Consider using a test automation framework like JUnit (for Java) or Selenium (for web applications).
Here's an example of a simple JUnit test case:
import static org.junit.jupiter.api.Assertions.*;
import org.junit.jupiter.api.Test;
public class CalculatorTest {
@Test
public void testAddition() {
Calculator calculator = new Calculator();
assertEquals(5, calculator.add(2, 3), "2 + 3 should equal 5");
}
}
This JUnit test validates the addition function of a hypothetical Calculator
class. Automated tests like these provide frequent assurance that the codebase remains in a deployable state.
4. Environment Consistency
Different environments (development, staging, production) can lead to discrepancies that break deployments. Inconsistent configurations can cause unpredictable behavior.
Solution: Use containerization technologies like Docker to ensure consistency across environments. Containers encapsulate applications and their dependencies, allowing them to run reliably across various computing environments.
An example of a Dockerfile for a simple Java application might look like this:
# Use an official Java runtime as a parent image
FROM openjdk:11
# Set the working directory in the container
WORKDIR /app
# Copy the local jar to the container
COPY target/myapp.jar myapp.jar
# Run the jar file
ENTRYPOINT ["java", "-jar", "myapp.jar"]
By using this Dockerfile, developers create a standardized environment that can be deployed in any stage of the pipeline.
5. Cultural Resistance
Successful CD requires a shift in mindset across the organization. Development, operations, and even business teams must embrace collaboration and communication.
Solution: Invest in training and foster a culture that promotes flexibility and rapid feedback. Encourage your teams to share insights and collaborate on problem-solving without fear of failure.
Lessons Learned
Adopting continuous deployment can be transformative for development teams, offering numerous advantages from faster deployment to enhanced collaboration. However, the challenges associated with automation, legacy systems, testing, environment consistency, and organizational culture cannot be overlooked.
By addressing these challenges head-on with thoughtful strategies, your organization can reap the full benefits of continuous deployment. Start small, iterate frequently, and create a culture of shared responsibility to ensure long-term success in your DevOps journey.
For an in-depth understanding of DevOps and Continuous Deployment, consider visiting The DevOps Handbook for comprehensive insights.
Feel free to leave your comments or questions below and share your experiences in implementing continuous deployment practices!