Common Pitfalls When Deploying Spring Microservices on Kubernetes
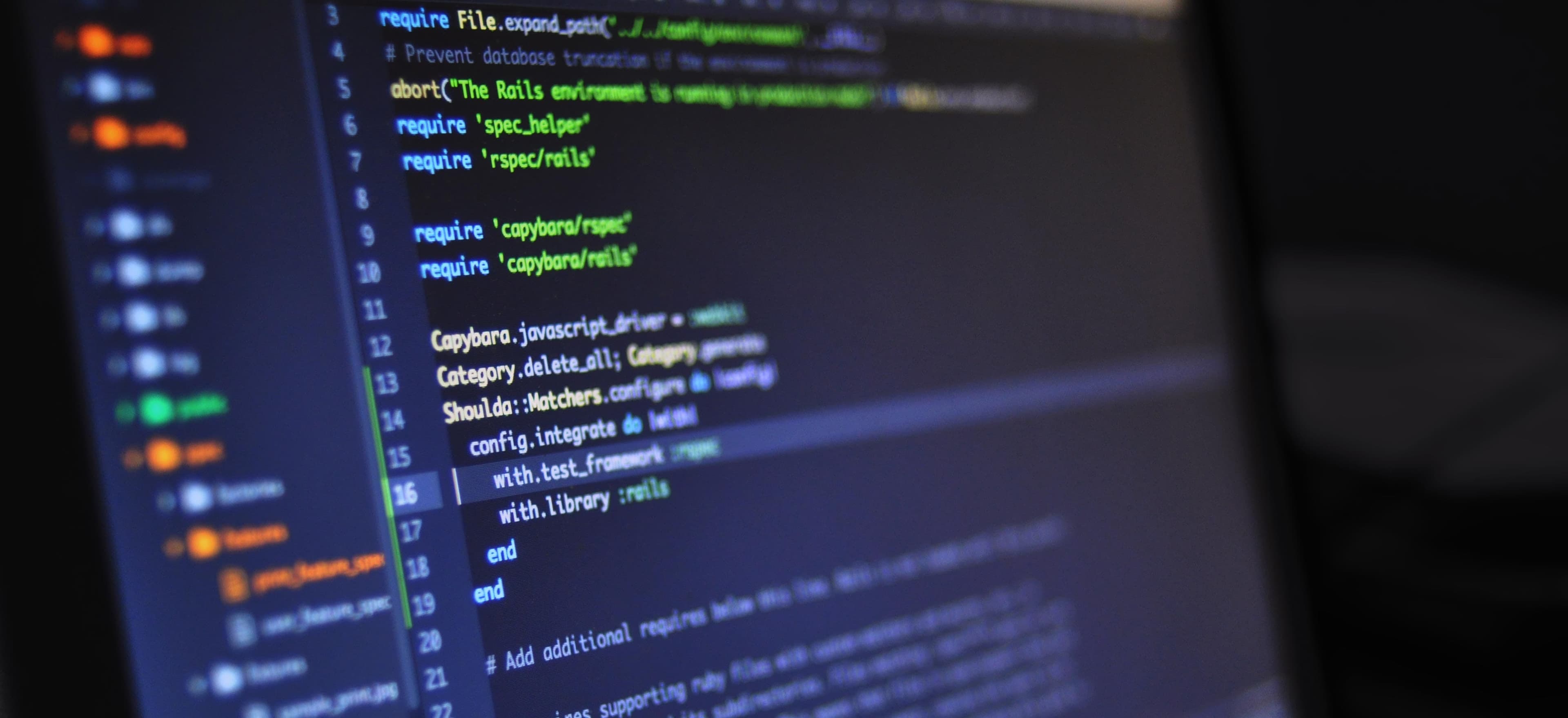
- Published on
Common Pitfalls When Deploying Spring Microservices on Kubernetes
As organizations increasingly shift towards microservices architectures, deploying these services on a cloud-native platform like Kubernetes has become standard practice. While both Spring and Kubernetes offer tremendous advantages, combining them introduces complexities that can lead to various pitfalls. In this article, we will explore common mistakes developers make when deploying Spring microservices on Kubernetes, along with practical solutions to help you avoid these traps.
1. Lack of Understanding of Kubernetes Concepts
The Importance of Kubernetes Fundamentals
Before diving into deploying Spring microservices, it's crucial to understand Kubernetes architecture. Familiarizing yourself with pods, services, and Deployments will give you a solid foundation.
Solutions
- Educational Resources: Invest time in learning about Kubernetes through online courses or documentation.
- Hands-on Experience: Set up a local Kubernetes cluster using tools like Minikube or Docker Desktop.
Example Code Snippet
To create a simple Deployment, you can use the following YAML configuration:
apiVersion: apps/v1
kind: Deployment
metadata:
name: spring-demo
spec:
replicas: 3
selector:
matchLabels:
app: spring-demo
template:
metadata:
labels:
app: spring-demo
spec:
containers:
- name: spring-demo
image: myrepository/spring-demo:latest
ports:
- containerPort: 8080
Why This Matters
Understanding these foundational elements ensures that you can effectively manage your deployments, scale services, and maintain availability in your microservices architecture.
2. Ignoring Configuration Management
Centralized Vs. Decentralized Configuration
One of the core features of Spring is its ability to manage configuration using application.properties
. However, when deploying to Kubernetes, developers often overlook the need for centralized configuration management.
Solutions
- Spring Cloud Config: Use Spring Cloud Config to keep your configurations in sync. It allows your services to pull configurations from a central place.
- Kubernetes ConfigMaps: Use Kubernetes ConfigMaps to store your configuration files and environment variables.
Example Code Snippet for Using ConfigMap
Create a ConfigMap in YAML to manage configurations centrally:
apiVersion: v1
kind: ConfigMap
metadata:
name: spring-demo-config
data:
application.properties: |
spring.datasource.url=jdbc:mysql://mysql:3306/springdb
spring.datasource.username=root
spring.datasource.password=secret
Why This Matters
Having a centralized configuration system prevents configuration drift and ensures that all your services are running with the same settings, which is vital for consistency in microservices.
3. Neglecting Health Checks
The Role of Health Checks
Failing to configure health checks can lead to cascading failures, as Kubernetes may not detect unhealthy Pods promptly.
Solutions
- Liveness Probes: Specify liveness probes in your Deployment YAML to check whether the application is running.
- Readiness Probes: Use readiness probes to tell Kubernetes when your app is ready to receive traffic.
Example Code Snippet
Adding health checks to your Deployment can be done as follows:
spec:
containers:
- name: spring-demo
image: myrepository/spring-demo:latest
ports:
- containerPort: 8080
livenessProbe:
httpGet:
path: /actuator/health
port: 8080
initialDelaySeconds: 30
periodSeconds: 10
readinessProbe:
httpGet:
path: /actuator/health
port: 8080
initialDelaySeconds: 15
periodSeconds: 10
Why This Matters
Implementing health checks minimizes downtime and ensures that Kubernetes can make informed decisions about your Pods, thus preventing service interruptions.
4. Overlooking Security Best Practices
Security in Microservices
Each microservice can become a potential attack vector. Hence, relying solely on the security of your Spring application is insufficient.
Solutions
- Role-Based Access Control (RBAC): Enable RBAC in your Kubernetes cluster to restrict user permissions.
- Service Mesh: Implement a service mesh like Istio or Linkerd for enhanced communication security between microservices.
Example Code Snippet for RBAC
A simple RBAC Policy can look like this:
apiVersion: rbac.authorization.k8s.io/v1
kind: Role
metadata:
namespace: default
name: spring-demo-role
rules:
- apiGroups: [""]
resources: ["pods"]
verbs: ["get", "watch", "list"]
Why This Matters
Implementing security best practices ensures that your microservices architecture remains secure against evolving threats.
5. Inadequate Logging and Monitoring
Importance of Monitoring
Microservices are inherently distributed, making traditional logging obsolete. Without proper logging and monitoring, debugging becomes a nightmare.
Solutions
- Distributed Tracing: Implement tracing with tools like Spring Cloud Sleuth and Zipkin.
- Centralized Logging: Use platforms like ELK (Elasticsearch, Logstash, Kibana) or Grafana to centralize logs.
Example Code Snippet for Sleuth
Adding Spring Cloud Sleuth to your Spring Boot application can be done in your pom.xml
:
<dependency>
<groupId>org.springframework.cloud</groupId>
<artifactId>spring-cloud-starter-sleuth</artifactId>
</dependency>
Why This Matters
Centralized logging and monitoring allow you to easily troubleshoot issues and better understand performance across your microservices.
6. Improper Resource Management
Resource Allocation in Kubernetes
Many developers forget to define resource requests and limits, leading to unpredictable behavior.
Solutions
- Resource Requests and Limits: Specify CPU and memory requests and limits in your Pod specifications.
Example Code Snippet
Below is how you can define resources in your Deployment YAML:
spec:
containers:
- name: spring-demo
image: myrepository/spring-demo:latest
ports:
- containerPort: 8080
resources:
requests:
memory: "512Mi"
cpu: "500m"
limits:
memory: "1Gi"
cpu: "1000m"
Why This Matters
Proper resource management helps ensure that each service gets the resources it needs while avoiding resource contention among services.
To Wrap Things Up
Deploying Spring microservices onto a Kubernetes platform can deliver unparalleled flexibility and scalability. However, awareness of the common pitfalls can significantly ease your deployment process. Remember to invest time in understanding the intricacies of both technologies, implement best practices for security and monitoring, and properly manage configurations and resources. Following these guidelines will help optimize your application’s performance, maintainability, and scalability.
For more effective learning, be sure to explore reference materials, such as the Spring Cloud documentation and the Kubernetes documentation, to deepen your understanding of these technologies as you work on your microservices. Happy coding!