Speeding Up App Testing: Overcoming CI/CD Bottlenecks
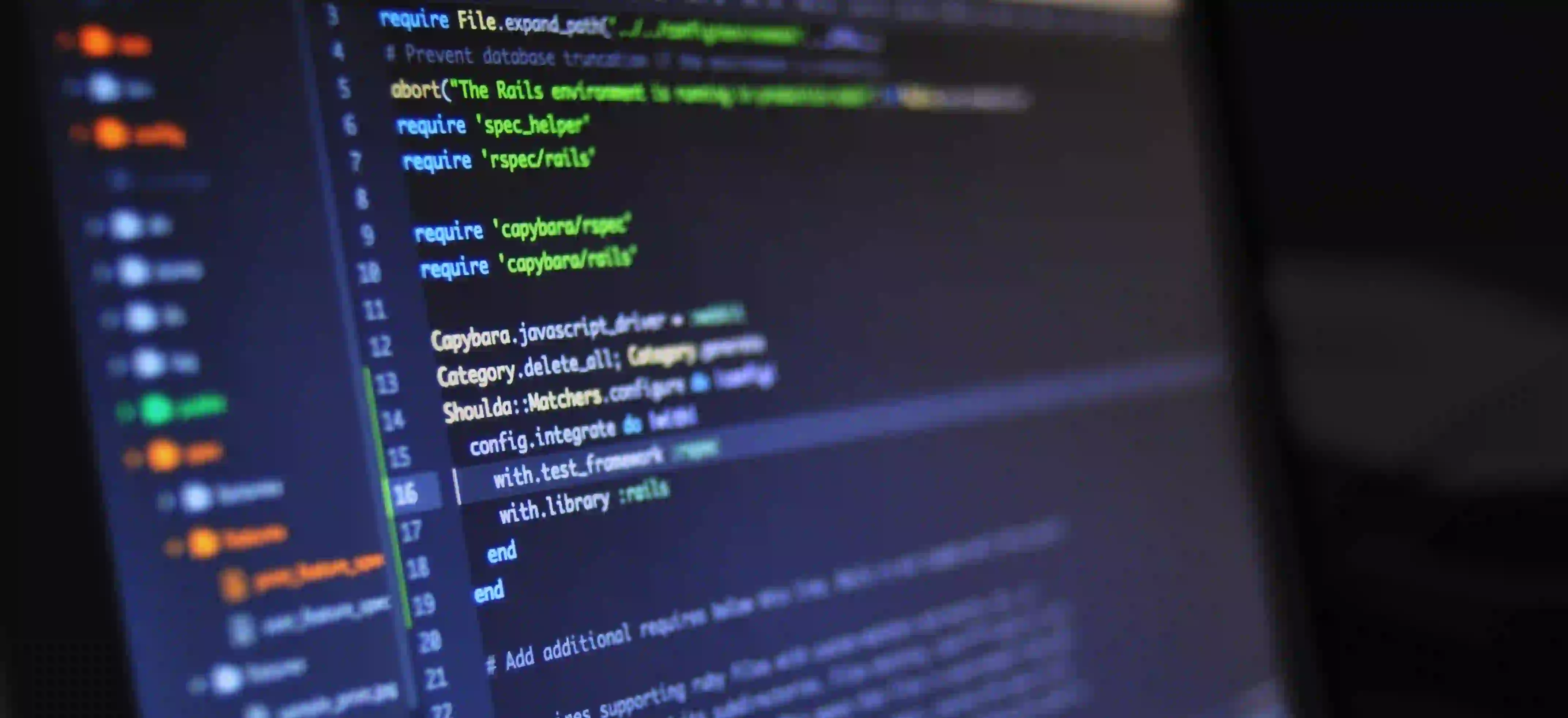
Speeding Up App Testing: Overcoming CI/CD Bottlenecks
In today's fast-paced development environment, Continuous Integration (CI) and Continuous Deployment (CD) have become vital in the software delivery lifecycle. However, as the scale of applications grows, teams often encounter bottlenecks in their testing processes that can slow down deployment cycles. This post explores how to overcome these challenges, ultimately speeding up app testing and enhancing overall productivity.
Understanding CI/CD Bottlenecks
What is CI/CD?
CI/CD is a set of practices designed to enhance code quality through automated testing and deployment. CI focuses on automating the integration of code changes from multiple contributors into a single software project. CD extends this by automating the deployment of verified code into production.
Common Bottlenecks in CI/CD
- Long Running Tests: Tests may take excessive time to run, leading to delays in feedback loops.
- Environment Issues: Differences between testing, staging, and production environments can lead to inconsistent results.
- Test Dependencies: Tests that depend on external systems can slow down the entire pipeline.
- Flaky Tests: Tests that produce unreliable results can lead to wasted time debugging issues that are not real.
- Infrastructure Saturation: Limited testing infrastructure can cause queuing, leading to increased cycle times.
Strategies to Overcome Bottlenecks
1. Parallel Testing
One of the most effective ways to reduce test execution time is through parallel testing. By distributing test execution across multiple machines or containers, you can significantly decrease the overall time required.
Example of Parallel Testing with JUnit and Maven:
<plugin>
<groupId>org.apache.maven.plugins</groupId>
<artifactId>maven-surefire-plugin</artifactId>
<version>2.22.2</version>
<configuration>
<parallel>methods</parallel>
<threadCount>10</threadCount>
</configuration>
</plugin>
Why? This configuration allows JUnit to run test methods in parallel using ten threads. This approach is crucial for large test suites since it reduces the time taken to run tests drastically, allowing quicker feedback.
2. Test Categorization
Segregate your tests into categories based on their necessity:
- Smoke Tests: Quick checks on major functionalities.
- Unit Tests: Covering specific pieces of code.
- Integration Tests: Testing interactions between different modules.
- End-to-End Tests: Verifying the complete workflow.
By categorizing tests, you can streamline your CI/CD pipeline. Running smoke tests on every commit and delaying slower tests until later stages can save time.
3. Prioritize Test Execution
Not all tests have equal weight. Prioritize tests based on recent code changes. Use tools like Codecov to understand code coverage and determine which tests are mission-critical.
Example Prioritization Logic:
@AfterClass
public static void prioritizeTests() {
List<Test> tests = getTests();
tests.sort((t1, t2) -> {
return Integer.compare(t1.getChangeImpact(), t2.getChangeImpact());
});
}
Why? Prioritizing tests enables the pipeline to focus on critical checks first, potentially halting runs when serious issues are detected early.
4. Embrace Containerization
Utilize technologies like Docker to create consistent testing environments. With containers, you can eliminate differences across environments, reducing the chances of environment-specific failures.
Dockerfile Example:
FROM openjdk:11-jdk
COPY target/myapp.jar /usr/src/myapp/myapp.jar
WORKDIR /usr/src/myapp
CMD ["java", "-jar", "myapp.jar"]
Why? By ensuring the same environment for development and testing, you diminish environment-related bottlenecks, leading to more reliable test outcomes.
5. Implementing Test Automation
Automate as many tests as possible. Use frameworks like Selenium for UI testing, or RestAssured for API testing. This can significantly reduce reliance on manual testing, allowing teams to run tests frequently across the CI/CD pipeline.
Example REST Assured for API Tests:
import io.restassured.RestAssured;
import io.restassured.response.Response;
public class ApiTests {
public void getUserDetails() {
Response response = RestAssured
.given()
.pathParam("userId", 1)
.when()
.get("https://jsonplaceholder.typicode.com/users/{userId}");
System.out.println("Response: " + response.getBody().asString());
}
}
Why? Harnessing API testing frameworks like RestAssured allows your QA process to be efficient, reducing the turnaround time for getting feedback on critical components.
6. Reduce Test Isolation Dependencies
Tests should be as independent as possible. External dependencies often slow down tests. Consider the following techniques:
- Mocking: Use libraries like Mockito to simulate dependencies.
- Stubbing: Create a simplified version of a service for testing purposes.
Example using Mockito:
import static org.mockito.Mockito.*;
public class UserServiceTest {
@Test
public void testFetchUser() {
UserService userService = mock(UserService.class);
when(userService.fetchUser(anyInt())).thenReturn(new User("John Doe"));
assertEquals("John Doe", userService.fetchUser(1).getName());
}
}
Why? Mocking dependencies allows tests to execute faster, making them independent of slower external systems and reducing the chances of failures tied to those systems.
Closing the Chapter
Speeding up app testing can greatly enhance CI/CD efficiency. Identify bottlenecks in your current process and consider implementing parallel testing, environment standardization, automation, and test categorization. Each of these strategies plays a critical role in minimizing delays and improving the quality of your application.
Investing time in refining your CI/CD pipeline will not only foster a culture of rapid iteration and delivery but also lead to better-quality software, providing a competitive edge in the market.
For more insights into CI/CD practices and tools, check out Atlassian CI/CD Resources or GitLab CI/CD.
Feel free to reach out and share your experiences with CI/CD in the comments below!