Troubleshooting WeatherLib Integration in Android App
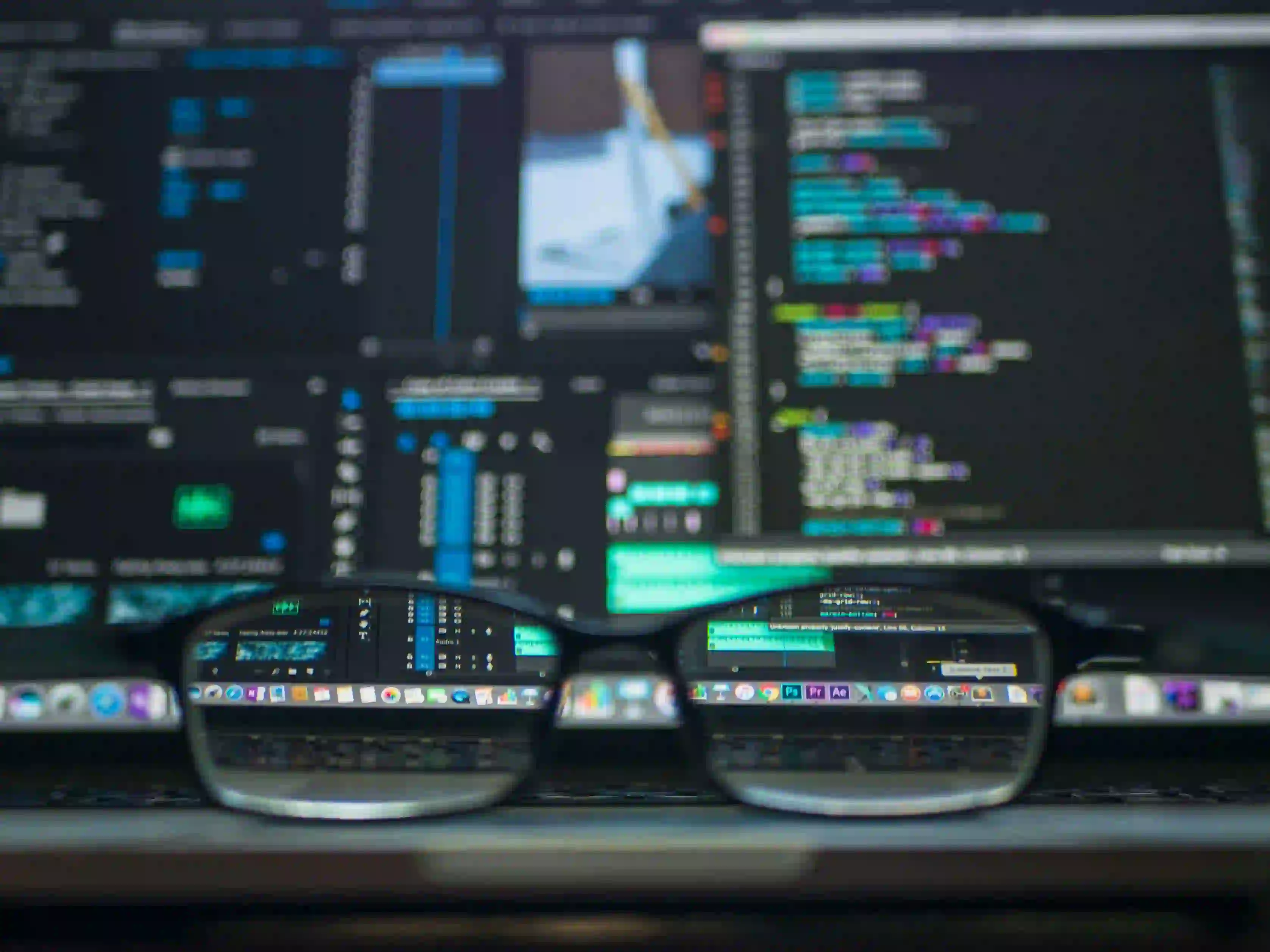
Troubleshooting WeatherLib Integration in Android App
WeatherLib is a powerful open-source library that allows developers to easily integrate weather information into their Android applications. However, integrating third-party libraries can sometimes lead to unexpected issues and bugs. In this article, we will discuss common problems that may arise when integrating WeatherLib into an Android app and provide solutions to troubleshoot and resolve these issues.
1. Gradle Configuration
Problem:
After adding the WeatherLib dependency in the app's build.gradle file, the app fails to build, showing errors related to unresolved dependencies.
Solution:
Ensure that the WeatherLib dependency is correctly added to the dependencies
block in the build.gradle file. Also, verify that the repositories containing the WeatherLib dependency are included in the repositories
block.
repositories {
// other repositories
maven {
url "https://dl.bintray.com/survivingwithandroid/maven"
}
}
dependencies {
// other dependencies
implementation 'com.github.survivingwithandroid:weatherlib:2.0.1'
}
2. Permissions
Problem:
The app is unable to fetch weather data, even after integrating WeatherLib, due to a lack of necessary permissions.
Solution:
Ensure that the app has the appropriate permissions declared in the AndroidManifest.xml
file. WeatherLib requires internet access to fetch weather data, so the INTERNET
permission is essential.
<uses-permission android:name="android.permission.INTERNET" />
3. API Key
Problem:
The WeatherLib integration is complete, but the app displays an error related to an invalid or missing API key.
Solution:
Some weather APIs require an API key for access. If you are using such an API with WeatherLib, ensure that you have registered for an API key and placed it correctly in your app. Additionally, verify that the API key is correctly passed when making weather requests using WeatherLib.
WeatherClient.ClientBuilder builder = new WeatherClient.ClientBuilder();
WeatherClient client = builder.attach(this)
.provider(new OpenweathermapProviderType())
.httpClient(WeatherClientDefault.httpClient) // can be customized
.config(new WeatherConfig())
.build();
client.updateWeatherConfig("your_api_key_here");
4. Internet Connectivity
Problem:
The app is unable to fetch weather data through WeatherLib, even with correct configurations, due to lack of internet connectivity.
Solution:
Ensure that the device has an active internet connection. It's also a good practice to check for internet connectivity programmatically before making weather requests using WeatherLib.
public boolean isInternetAvailable() {
ConnectivityManager connectivityManager = (ConnectivityManager) getSystemService(Context.CONNECTIVITY_SERVICE);
NetworkInfo activeNetworkInfo = connectivityManager.getActiveNetworkInfo();
return activeNetworkInfo != null && activeNetworkInfo.isConnected();
}
5. Proguard Rules
Problem:
After integrating WeatherLib, the app crashes or malfunctions in release builds due to Proguard obfuscating necessary WeatherLib classes and methods.
Solution:
If using Proguard for release builds, ensure that proper Proguard rules are in place to keep the WeatherLib code intact. The WeatherLib documentation or GitHub repository may provide specific Proguard rules to include in the Proguard configuration file.
# WeatherLib Proguard rules
-keep class com.survivingwithandroid.weather.lib.model.** { *; }
-keep interface com.survivingwithandroid.weather.lib.model.** { *; }
The Bottom Line
Integrating WeatherLib into an Android app can greatly enhance its functionality by providing real-time weather information. However, encountering issues during the integration process is not uncommon. By following the troubleshooting steps outlined in this article, you can effectively identify and resolve problems related to Gradle configuration, permissions, API keys, internet connectivity, and Proguard rules, ensuring a smooth integration of WeatherLib into your Android app. For further reference, you can explore the WeatherLib GitHub repository and the official documentation.
Happy coding!