Handling JSON Response in JSF
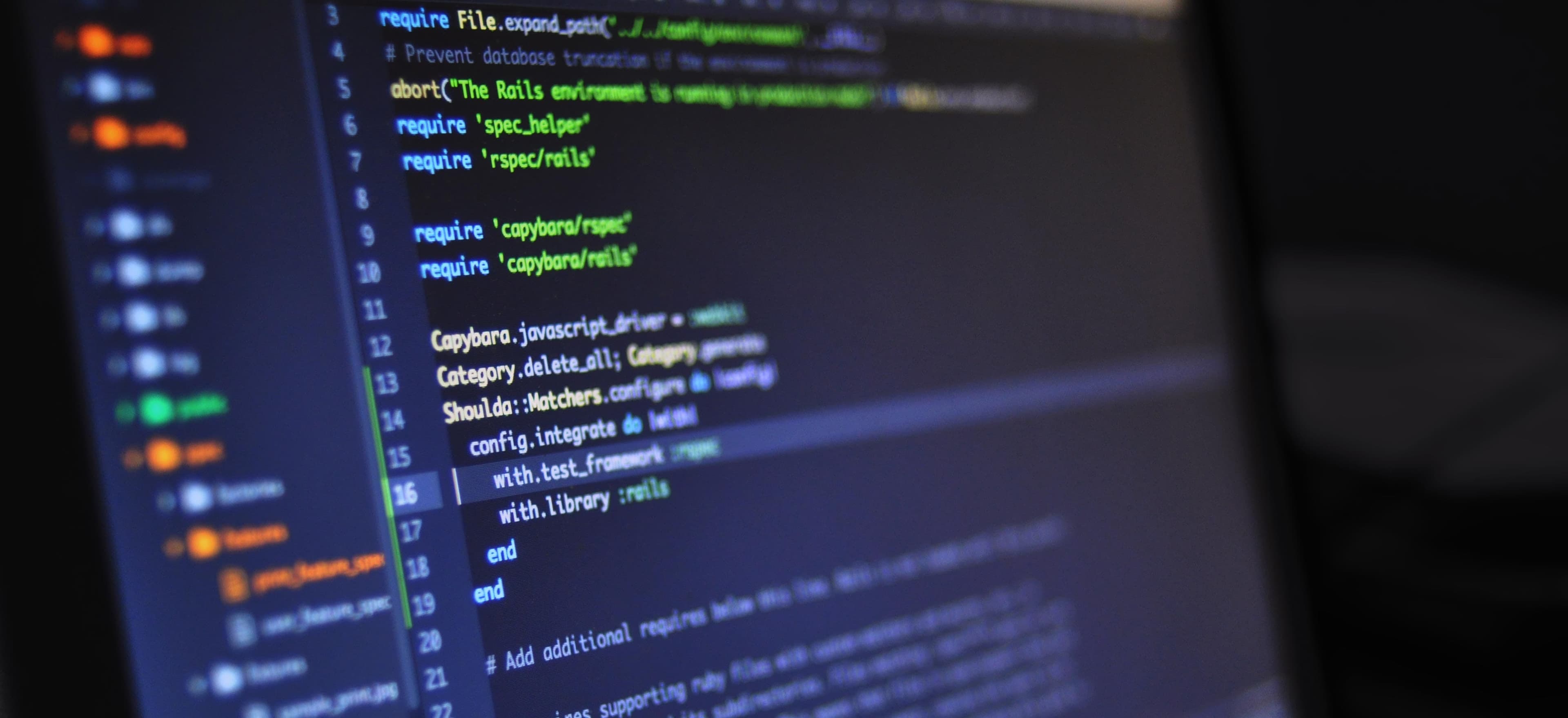
- Published on
Mastering JSON Response Handling in Java Server Faces (JSF)
In the realm of web development, Java Server Faces (JSF) has garnered immense popularity due to its robust features and seamless integration with enterprise-level applications. One crucial aspect of web development is handling JSON responses, which enables the seamless exchange of data between the front-end and back-end systems. In this comprehensive guide, we will delve into the intricacies of handling JSON responses in JSF, equipping you with the knowledge to effortlessly manage inbound and outbound JSON data within your JSF applications.
Understanding JSON
JSON (JavaScript Object Notation) has emerged as a ubiquitous data interchange format due to its lightweight and human-readable nature. It serves as a fundamental building block for modern web applications, facilitating the efficient transfer of structured data between servers and clients. With its flexible key-value pair structure, JSON has become the preferred choice for transmitting data in web-based environments.
Leveraging JSON in JSF
In JSF, the integration and manipulation of JSON responses are facilitated through the use of suitable libraries and techniques. One of the fundamental approaches involves utilizing the javax.json package to handle JSON data seamlessly. The package offers a comprehensive set of tools for parsing, creating, and manipulating JSON data within the JSF framework, empowering developers to orchestrate a smooth flow of data between the server and client.
Let's dive into a practical example to showcase the implementation of JSON response handling in JSF.
Example: Creating a JSON Response in JSF
import javax.json.Json;
import javax.json.JsonObject;
import javax.json.JsonArray;
public class JsonHandler {
public String generateJsonResponse() {
JsonObject personObject = Json.createObjectBuilder()
.add("name", "John Doe")
.add("age", 30)
.add("isMarried", true)
.build();
JsonArray hobbiesArray = Json.createArrayBuilder()
.add("hiking")
.add("reading")
.add("painting")
.build();
JsonObject jsonResponse = Json.createObjectBuilder()
.add("person", personObject)
.add("hobbies", hobbiesArray)
.build();
return jsonResponse.toString();
}
}
In the above example, we have created a JsonHandler class equipped with a method named generateJsonResponse(). This method constructs a JSON response comprising a person object and an array of hobbies using the javax.json package. The JSON response is then returned as a string, ready to be utilized within the JSF application.
Consuming JSON Response in JSF
Once the JSON response is generated, the subsequent step involves consuming and processing the incoming JSON data within the JSF application. JSF provides a straightforward mechanism to accomplish this task by leveraging the h:outputScript tag, which enables the seamless incorporation of JavaScript functionality within the application.
Let's explore an exemplar JSF page that demonstrates the consumption of a JSON response.
<html xmlns="http://www.w3.org/1999/xhtml"
xmlns:h="http://xmlns.jcp.org/jsf/html"
xmlns:f="http://xmlns.jcp.org/jsf/core">
<h:head>
<title>JSON Response Handling in JSF</title>
<h:outputScript library="js" name="json-handler.js" target="head"/>
</h:head>
<h:body>
<h1>JSON Response Consumption in JSF</h1>
<h:form>
<h:commandButton value="Load JSON Data" onclick="loadJsonData()"/>
</h:form>
</h:body>
</html>
In the above JSF page, the h:outputScript tag is utilized to include an external JavaScript file json-handler.js that contains the logic to handle and process the JSON response. Upon the user's command button interaction, the JavaScript function loadJsonData() is triggered to fetch and handle the JSON response seamlessly.
Closing the Chapter
In conclusion, mastering the art of handling JSON responses in JSF is paramount for building dynamic and responsive web applications. By embracing the nuances of manipulating JSON data within JSF, developers can elevate the functionality and interactivity of their applications, ultimately delivering a seamless user experience.
In this guide, we have meticulously navigated through the essentials of handling JSON responses in JSF, unraveling the process of generating and consuming JSON data within the JSF framework. Armed with this knowledge, you are well-equipped to infuse your JSF applications with the power of JSON, fostering a robust and agile data exchange mechanism.
Embark on your journey of harnessing JSON response handling in JSF, and witness your web applications flourish with enhanced data exchange capabilities!
For further exploration of JSF and JSON integration, feel free to delve into the official documentation and JSF JSON handling libraries. Happy coding!