Mitigating Buffer Overload with Back Pressure
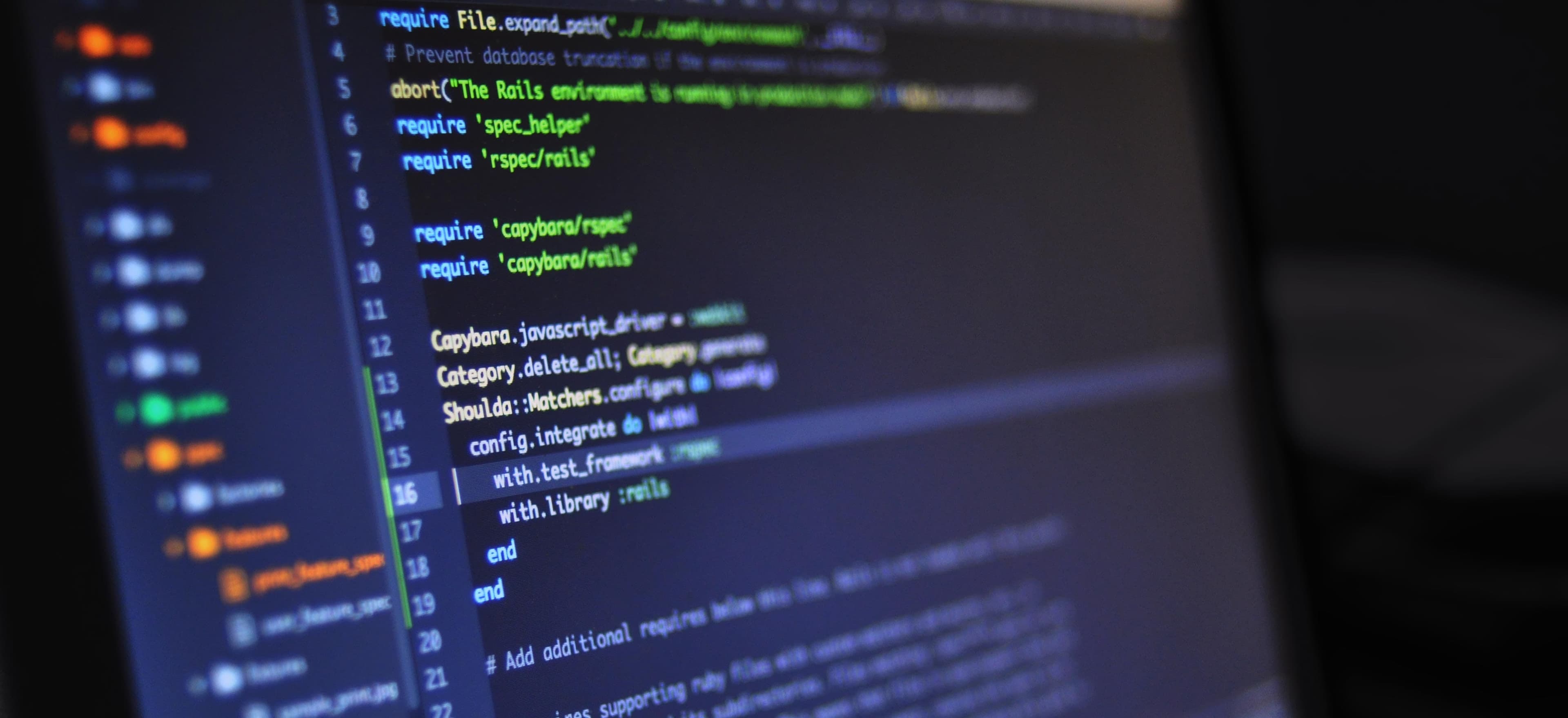
- Published on
Mitigating Buffer Overload with Back Pressure
When developing applications in Java, it's crucial to prevent buffer overload, which can lead to performance degradation, latency, and even system crashes. One effective way to address this issue is by implementing back pressure. In this article, we'll explore what back pressure is, why it's essential, and how to implement it in Java applications.
Understanding Back Pressure
Back pressure is a strategy used in reactive systems to manage the flow of data in a way that prevents the overflow of buffers or the exhaustion of resources. It enables components in a system to communicate and coordinate the rate at which data is produced and consumed.
In the context of Java programming, back pressure is particularly relevant in asynchronous and reactive programming paradigms, where data streams are prevalent. Without back pressure, a fast data producer could overwhelm a slower consumer, leading to buffer overflow and system instability.
The Importance of Back Pressure in Java Applications
In Java applications, especially those involving streams of data or asynchronous processing, the absence of back pressure can result in various issues, including:
- Buffer Overload: Without back pressure, a fast producer can inundate a slow consumer with data, leading to buffer overload and potential memory exhaustion.
- Increased Latency: When a system is overwhelmed with data, it can cause processing delays and increased latency, impacting the overall performance and responsiveness of the application.
- Resource Exhaustion: Uncontrolled data flow can lead to the exhaustion of system resources, potentially causing the application to become unresponsive or crash.
Given these potential pitfalls, implementing back pressure in Java applications is essential for maintaining system stability and preventing performance degradation.
Implementing Back Pressure in Java
Now let's delve into how back pressure can be implemented in Java applications. One of the prevalent libraries for dealing with reactive streams and back pressure in Java is Project Reactor, which provides support for reactive programming in Java.
Using Project Reactor for Back Pressure
Project Reactor, an implementation of the Reactive Streams specification, offers built-in support for back pressure. It introduces the concept of Flux
and Mono
for handling streams, along with operators that enable controlling the flow of data.
Example: Implementing Back Pressure with Project Reactor
import reactor.core.publisher.Flux;
import java.time.Duration;
public class BackPressureExample {
public static void main(String[] args) {
Flux.range(1, 1000)
.delayElements(Duration.ofMillis(100))
.onBackpressureDrop()
.subscribe(data -> System.out.println("Received: " + data));
}
}
In this example, we create a Flux
that emits integers from 1 to 1000. We introduce back pressure handling by using the delayElements
operator to simulate a slow consumer. Additionally, we apply the onBackpressureDrop
operator, which discards excess data when the subscriber can't keep up.
Custom Back Pressure Strategies
Aside from using libraries like Project Reactor, it's also possible to implement custom back pressure strategies in Java. This can be achieved by leveraging constructs such as Semaphore
or custom queue implementations to control the flow of data between producers and consumers.
Example: Custom Back Pressure Strategy
import java.util.concurrent.ArrayBlockingQueue;
import java.util.concurrent.BlockingQueue;
public class CustomBackPressureExample {
public static void main(String[] args) {
BlockingQueue<Integer> queue = new ArrayBlockingQueue<>(10);
Runnable producer = () -> {
// Produce data and add to the queue
// Handle back pressure if the queue is full
};
Runnable consumer = () -> {
// Consume data from the queue
// Handle back pressure if the queue is empty
};
// Execute producer and consumer threads
}
}
In this custom back pressure example, we utilize an ArrayBlockingQueue
to control the flow of data between the producer and the consumer. The producer and consumer threads are responsible for handling back pressure based on the state of the queue.
A Final Look
In Java applications, mitigating buffer overload with back pressure is essential for maintaining system stability, preventing performance degradation, and ensuring efficient data flow. By understanding the significance of back pressure and implementing it using tools like Project Reactor or custom strategies, developers can build robust and resilient applications that effectively manage the flow of data.
Implementing back pressure not only enhances the reliability of Java applications but also contributes to the overall efficiency and responsiveness of reactive systems. Therefore, it is imperative for Java developers to prioritize the incorporation of back pressure strategies in their applications to mitigate buffer overload effectively.