Defending Your Application Against Faults: Leveraging Hystrix
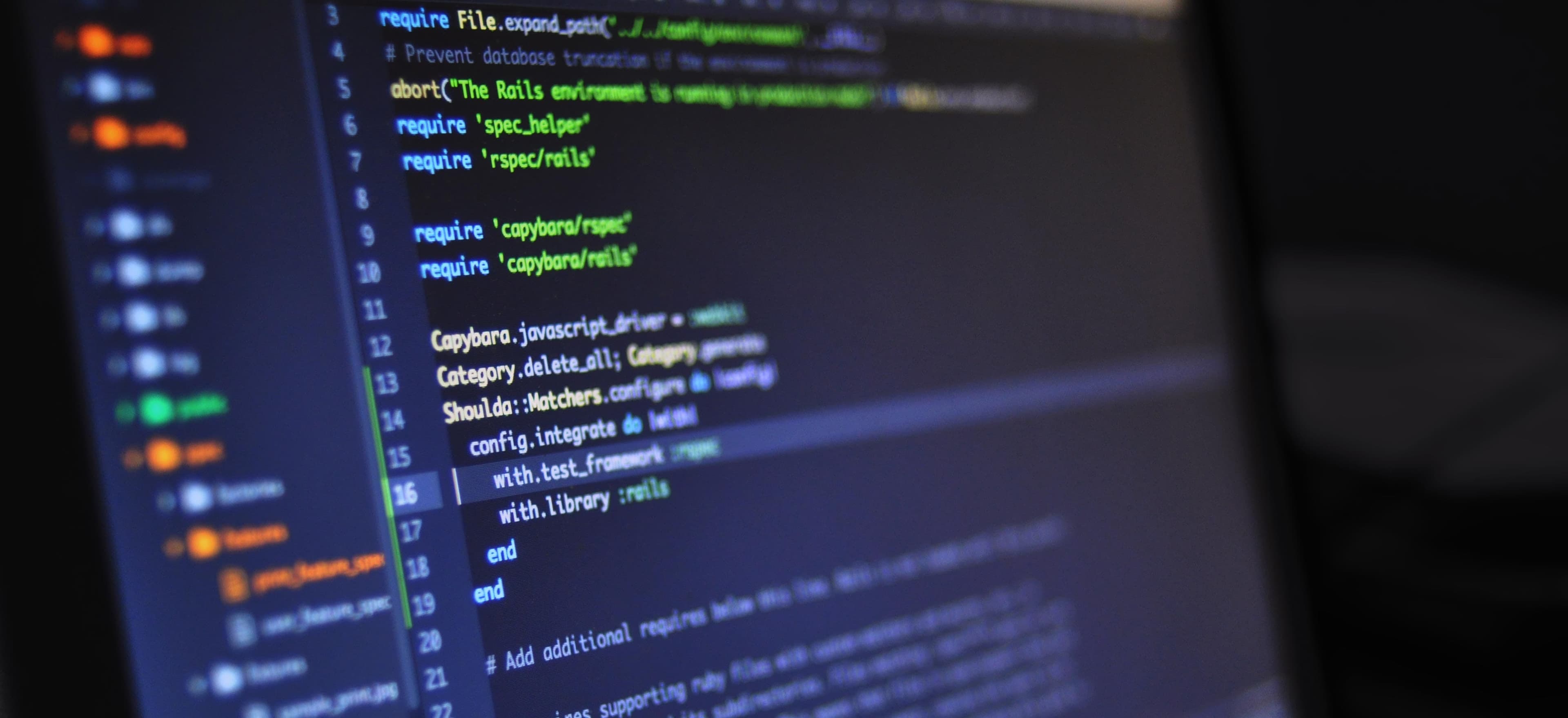
- Published on
Defending Your Application Against Faults: Leveraging Hystrix
In today's interconnected digital landscape, where software systems often depend on multiple external services, the robustness and resilience of an application are of paramount importance. A single point of failure in any of the services can have a cascading effect, leading to degradation or even complete failure of the entire system. This is where circuit breakers come into play as an essential component of fault-tolerant distributed systems.
The Need for Circuit Breakers
Imagine a scenario where a service your application depends on suddenly experiences increased latency or starts returning errors due to overload. Without a circuit breaker mechanism in place, your application might keep making repeated calls to the failing service, further exacerbating the situation and potentially causing a complete system meltdown. What's needed is a safeguard that can detect when a service is failing and proactively prevent further requests from being made to it, thereby allowing the system to gracefully degrade rather than catastrophically fail.
Enter Hystrix
This is where Hystrix, a latency and fault tolerance library provided by Netflix, comes to the rescue. Hystrix is designed to stop cascading failures in a distributed environment, providing a fail-fast mechanism that can help improve the overall resiliency of your system. It achieves this by isolating points of access between the services, stopping the spread of failures across the system, and providing fallback options when things go awry.
Key Features of Hystrix
-
Circuit Breaker: Hystrix monitors the availability and latency of services. If the error rate exceeds a certain threshold, it opens the circuit, preventing calls to the failing service for a specified duration.
-
Fallback: Hystrix allows you to define fallback logic that will be executed when a service call fails or times out.
-
Thread and Semaphore Isolation: Hystrix provides multiple strategies for isolating access points to remote services, preventing high latency or failure from impacting the calling services.
-
Request Caching and Collapsing: Hystrix has built-in support for request caching and collapsing, which can help optimize the performance of your application.
Now, let's dive into some code examples to see how Hystrix can be integrated into a Java application to protect it against faults.
Integrating Hystrix into a Java Application
First, let's add the Hystrix library to your Maven project by including the following dependency:
<dependency>
<groupId>com.netflix.hystrix</groupId>
<artifactId>hystrix-core</artifactId>
<version>1.5.18</version>
</dependency>
With Hystrix added to the project, let's look at a practical example of using it to protect a critical service call.
Using Hystrix Commands
In Hystrix, the primary mechanism for encapsulating remote calls and applying resilience patterns is through the concept of Hystrix Commands. A Hystrix Command represents a single request and its associated fallback logic. Let's start by creating a simple Hystrix Command to encapsulate a remote service call.
import com.netflix.hystrix.HystrixCommand;
import com.netflix.hystrix.HystrixCommandGroupKey;
import com.netflix.hystrix.HystrixCommandKey;
public class RemoteCallCommand extends HystrixCommand<String> {
private final String fallbackGreeting = "Hello, Guest";
public RemoteCallCommand() {
super(HystrixCommand.Setter
.withGroupKey(HystrixCommandGroupKey.Factory.asKey("RemoteServiceGroup"))
.andCommandKey(Hystjson
.andCommandKey(HystrixCommandKey.Factory.asKey("RemoteServiceCall")));
}
@Override
protected String run() throws Exception {
// Make the actual remote service call here
// Return the result
}
@Override
protected String getFallback() {
return fallbackGreeting;
}
}
In this example, we've created a RemoteCallCommand
that extends the HystrixCommand
class, encapsulating the remote service call within the run
method. If the call fails, the getFallback
method will return a predefined fallback response.
Implementing Circuit Breaker with Hystrix
Now, let's take a look at how to utilize the circuit breaker feature of Hystrix to protect our application from cascading failures.
import com.netflix.hystrix.HystrixCommand;
import com.netflix.hystrix.HystrixCommandGroupKey;
public class CircuitBreakerCommand extends HystrixCommand<String> {
public CircuitBreakerCommand() {
super(HystrixCommand.Setter
.withGroupKey(HystrixCommandGroupKey.Factory.asKey("CircuitBreakerGroup")));
}
@Override
protected String run() throws Exception {
// Make the remote service call
// Return the result
}
}
In this example, the CircuitBreakerCommand
encapsulates the remote service call, and Hystrix will apply its circuit breaker logic based on the configuration provided. If the error threshold is crossed, the circuit will open, and subsequent calls to the run
method will automatically trigger the fallback logic.
Final Thoughts
In a world where distributed systems are the norm, building fault-tolerant applications is crucial. Hystrix provides a powerful toolkit to help you defend your application against faults and build resilient, responsive, and scalable systems. By incorporating circuit breakers, fallback mechanisms, and error isolation strategies, Hystrix empowers you to handle the complexities of distributed systems with confidence.
By leveraging Hystrix in your Java applications, you can proactively protect your services and ensure that failures in one part of the system do not bring down the entire application. Its ease of integration and powerful features make it a vital tool for any developer building distributed, resilient systems.
To delve deeper into Hystrix and its full range of capabilities, visit the official Hystrix documentation and start unlocking the potential of fault tolerance in your applications.