Troubleshooting Hibernate 3.5 Integration with Spring 3 and JPA 2
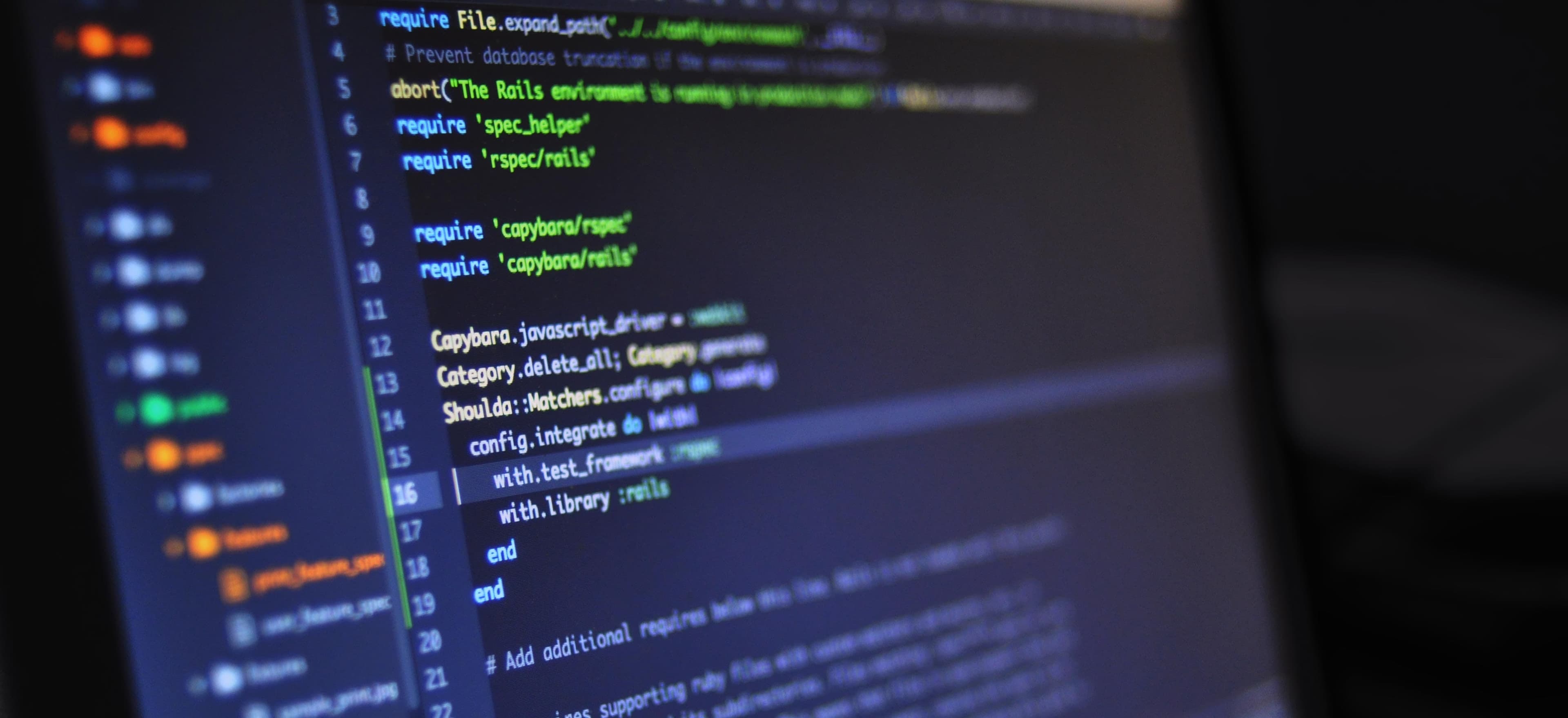
- Published on
Troubleshooting Hibernate 3.5 Integration with Spring 3 and JPA 2
When working with Java development, it's common to use Hibernate as an Object/Relational Mapping (ORM) tool to map Java objects to database tables. In this blog post, we'll explore the integration of Hibernate 3.5 with the Spring 3 framework and JPA 2, focusing on troubleshooting common issues that developers may encounter.
Understanding the Integration
Before diving into troubleshooting, it's essential to understand how Hibernate 3.5 integrates with Spring 3 and JPA 2.
Hibernate 3.5 and JPA 2
Hibernate supports the Java Persistence API (JPA) and provides its implementation, allowing developers to utilize JPA annotations for mapping entities. With the release of JPA 2, developers gained additional features such as criteria queries, validation, and more, further enhancing the capabilities of Hibernate.
Spring 3 and Hibernate Integration
Spring Framework provides excellent support for integrating Hibernate with its powerful inversion of control (IoC) and aspect-oriented programming (AOP) features. With Spring 3, the integration with Hibernate is streamlined, allowing for easy configuration of Hibernate session factories, transactions, and more.
Troubleshooting Common Issues
Let's explore some common issues that developers may encounter when integrating Hibernate 3.5 with Spring 3 and JPA 2 and discuss how to address them.
Issue 1: Incorrect Configuration of Session Factory Bean
One common issue is the incorrect configuration of the Hibernate session factory bean in the Spring configuration file. This can lead to errors when attempting to interact with the database.
Solution:
Ensure that the session factory bean is configured properly, with the correct data source and JPA vendor adapter. Here's an example of a session factory bean configuration in the Spring XML file:
<bean id="sessionFactory"
class="org.springframework.orm.hibernate5.LocalSessionFactoryBean">
<property name="dataSource" ref="dataSource" />
<property name="packagesToScan" value="com.example.model" />
<property name="hibernateProperties">
<props>
<prop key="hibernate.dialect">org.hibernate.dialect.MySQL5Dialect</prop>
<prop key="hibernate.show_sql">true</prop>
</props>
</property>
</bean>
In the above code snippet, the session factory bean is configured to use a data source and scan the specified packages for entity classes. Additionally, Hibernate properties such as the dialect and SQL output are set.
Issue 2: Transaction Management Errors
Another common issue is related to transaction management, where developers may encounter errors when dealing with database transactions.
Solution:
Ensure that the transaction manager is configured correctly in the Spring XML file. Here's an example of a transaction manager configuration:
<bean id="transactionManager"
class="org.springframework.orm.hibernate5.HibernateTransactionManager">
<property name="sessionFactory" ref="sessionFactory" />
</bean>
The above configuration sets up a Hibernate transaction manager that references the session factory bean.
Issue 3: Mapping Entity Classes
Developers may face issues with mapping entity classes using JPA annotations, leading to runtime errors when performing database operations.
Solution:
When using JPA annotations for entity mapping, ensure that the annotations are correctly applied to the entity classes. For example:
@Entity
@Table(name = "employees")
public class Employee {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
@Column(name = "id")
private Long id;
// Other entity properties and methods
}
In the above code snippet, the @Entity
, @Table
, @Id
, @GeneratedValue
, and @Column
annotations are used to map the Employee
class to the employees
table in the database.
Issue 4: Database Connection and Dialect Configuration
Incorrect configuration of the database connection and Hibernate dialect can lead to errors when attempting to connect to the database.
Solution:
Ensure that the database connection properties and Hibernate dialect are configured properly. For example, in the Spring XML file, define the data source bean and hibernate properties:
<bean id="dataSource"
class="org.springframework.jdbc.datasource.DriverManagerDataSource">
<property name="driverClassName" value="com.mysql.cj.jdbc.Driver" />
<property name="url" value="jdbc:mysql://localhost:3306/mydb" />
<property name="username" value="username" />
<property name="password" value="password" />
</bean>
In the above code snippet, the data source bean is configured with the MySQL database connection properties.
Issue 5: Maven Dependencies
Missing or incorrect Maven dependencies for Hibernate, Spring, and JPA can result in compilation and runtime errors.
Solution:
Ensure that the pom.xml
file includes the required dependencies for Hibernate, Spring, and JPA. For example:
<dependency>
<groupId>org.hibernate</groupId>
<artifactId>hibernate-core</artifactId>
<version>3.5.6-Final</version>
</dependency>
<dependency>
<groupId>org.springframework</groupId>
<artifactId>spring-orm</artifactId>
<version>3.0.5.RELEASE</version>
</dependency>
<dependency>
<groupId>javax.persistence</groupId>
<artifactId>persistence-api</artifactId>
<version>1.0.2</version>
</dependency>
In the above code snippet, the pom.xml
file specifies the Hibernate, Spring, and JPA dependencies.
A Final Look
In this blog post, we explored the integration of Hibernate 3.5 with Spring 3 and JPA 2, focusing on troubleshooting common issues that developers may encounter. By understanding the integration and addressing common issues such as session factory configuration, transaction management, entity mapping, database connection, and Maven dependencies, developers can overcome challenges and build robust applications with Hibernate, Spring, and JPA.
I hope this post provides valuable insights and solutions for troubleshooting Hibernate 3.5 integration with Spring 3 and JPA 2.
For more in-depth information on Hibernate, Spring, and JPA, check out the following resources: