Understanding HashMap Collision Resolution Techniques
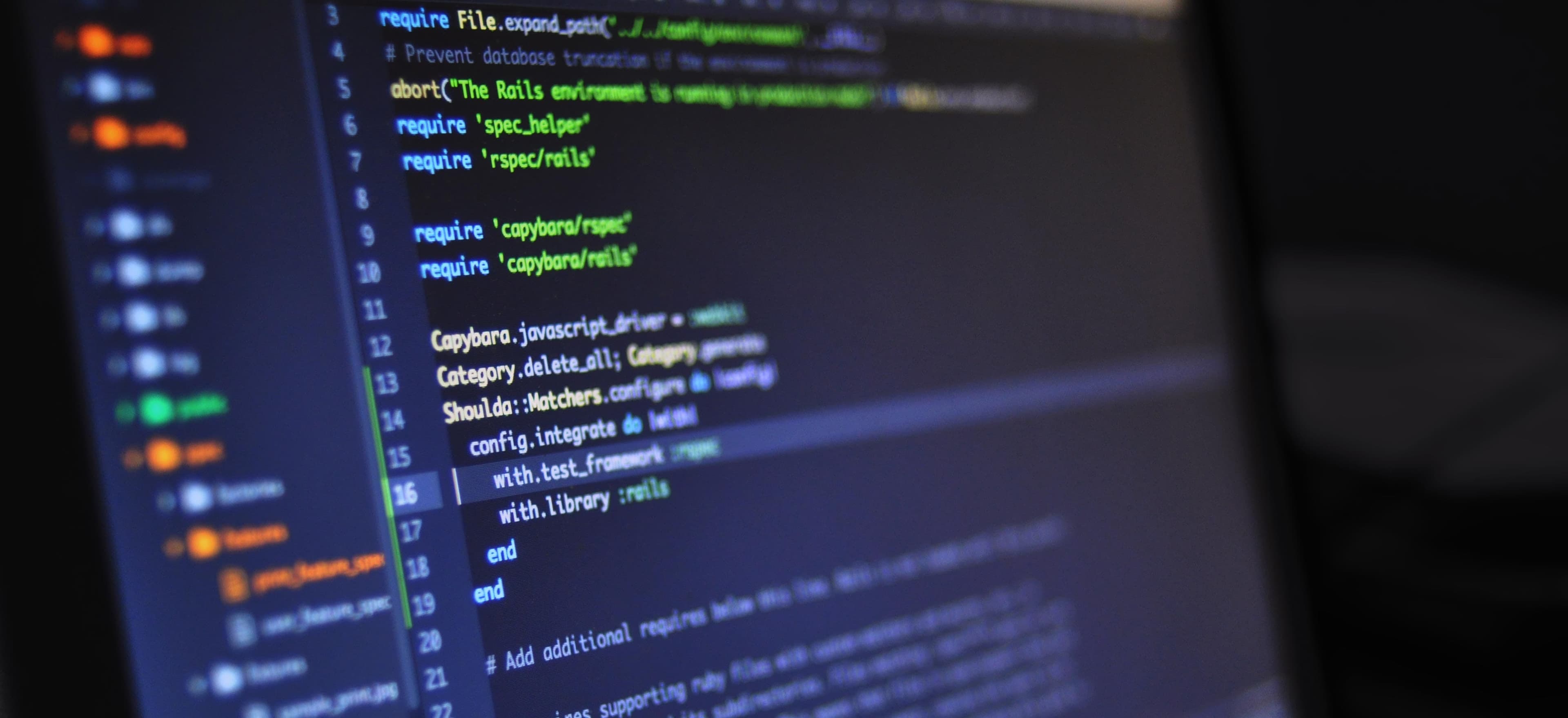
- Published on
Understanding HashMap Collision Resolution Techniques
In Java, the HashMap
class allows us to store key-value pairs and provides constant-time performance for basic operations like get and put. However, when different keys map to the same bucket in the hash table, a collision occurs. Resolving collisions is crucial to ensure the HashMap
operates efficiently. In this article, we will discuss the various collision resolution techniques used in Java's HashMap
to handle collisions.
What is a HashMap Collision?
A collision in a HashMap
occurs when two or more distinct keys hash to the same index or bucket in the underlying array. This typically happens due to the limited range of hash codes and the use of a modulo operation to map the hash code to an index in the array.
To handle collisions, HashMap
uses several techniques to store and retrieve data efficiently. Let's explore these collision resolution techniques:
1. Separate Chaining
Separate chaining is one of the most commonly used collision resolution techniques. In this technique, each bucket of the array is actually a linked list of entries. When a collision occurs, the entries are appended to the same bucket.
Example of Separate Chaining
public class Entry<K, V> {
K key;
V value;
Entry<K, V> next;
// Constructor, getters, and setters
}
In this example, the Entry
class represents an entry in the HashMap
. The next
field allows chaining entries together. When a collision occurs, a new entry is appended to the existing entry's next
pointer, forming a linked list.
The benefit of separate chaining is that it handles an arbitrary number of collisions for a given bucket. However, if not implemented carefully, it can lead to performance degradation when the linked lists become too long.
2. Open Addressing
Open addressing is another technique used to resolve collisions in a HashMap
. In open addressing, when a collision occurs, the algorithm probes the hash table for an empty slot to place the new key-value pair.
Example of Open Addressing
public class LinearProbingHashMap<K, V> {
private int capacity;
private K[] keys;
private V[] values;
// Constructor and other methods
private int hash(K key) {
return (key.hashCode() & 0x7fffffff) % capacity;
}
private void put(K key, V value) {
int i;
for (i = hash(key); keys[i] != null; i = (i + 1) % capacity) {
if (keys[i].equals(key)) {
values[i] = value;
return;
}
}
keys[i] = key;
values[i] = value;
}
// Other methods for get, delete, etc.
}
In this example, the LinearProbingHashMap
class uses linear probing as the open addressing technique. When a collision occurs, the algorithm checks the next slot in the array until it finds an empty slot for the new key-value pair.
Open addressing is more memory-efficient compared to separate chaining, as it avoids the overhead of managing linked lists. However, it can suffer from clustering issues, leading to degraded performance.
A Final Look
In conclusion, understanding how HashMap
handles collisions is crucial for Java developers to write efficient and performant code. Both separate chaining and open addressing have their strengths and weaknesses, and choosing the right collision resolution technique depends on various factors such as expected load factor, key distribution, and the number of key-value pairs.
Understanding these collision resolution techniques not only enhances our understanding of HashMap
but also allows us to make informed decisions when designing and implementing data structures in Java.
To deepen your understanding of HashMap
and related topics, check out "The Java™ Tutorials" and "Effective Java" by Joshua Bloch.
Happy coding!