Converting JSON Array to Java List with Gson: Handling Nested Objects
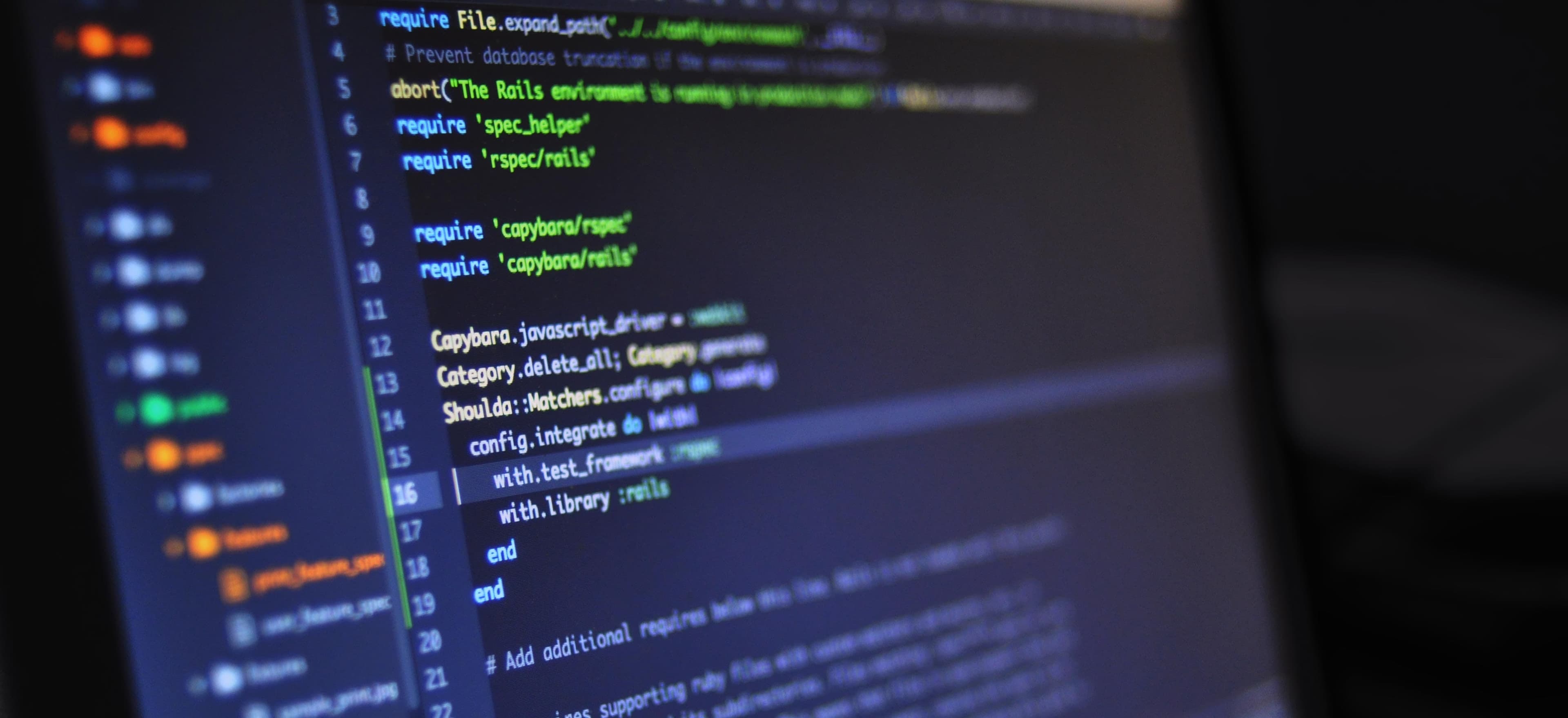
- Published on
Converting JSON Array to Java List with Gson: Handling Nested Objects
In Java, working with JSON data is a common requirement. The Gson library provides a convenient way to convert JSON objects and arrays into Java objects, and vice versa. In this blog post, we'll explore how to use Gson to convert a JSON array into a Java List when dealing with nested objects.
Understanding the Scenario
Imagine you have a JSON array containing nested objects, and you need to deserialize this JSON array into a Java List of objects. Each object within the JSON array may have its own set of properties and nested objects. We'll walk through the process of handling such a scenario using Gson in Java.
Setting Up the Project
To begin, ensure that you have the Gson library added to your project. If you're using Maven, you can include Gson as a dependency in your pom.xml
:
<dependency>
<groupId>com.google.code.gson</groupId>
<artifactId>gson</artifactId>
<version>2.8.7</version>
</dependency>
If you're using Gradle, add the following to your build.gradle
file:
implementation 'com.google.code.gson:gson:2.8.7'
Creating the Java Objects
Let's define the Java objects that correspond to the nested structure of the JSON array. For this example, we'll assume a simple scenario where we have a JSON array of Person
objects, and each Person
has a list of Address
objects.
Person Class
public class Person {
private String name;
private int age;
private List<Address> addresses;
// getters and setters
}
Address Class
public class Address {
private String street;
private String city;
private String country;
// getters and setters
}
Deserializing JSON into Java List
Now, let's see how we can use Gson to deserialize the JSON array into a Java List of Person
objects.
JSON Array Example
[
{
"name": "John Doe",
"age": 30,
"addresses": [
{
"street": "123 Main St",
"city": "Anytown",
"country": "USA"
},
{
"street": "456 Elm St",
"city": "Othertown",
"country": "USA"
}
]
},
{
"name": "Jane Smith",
"age": 25,
"addresses": [
{
"street": "789 Oak St",
"city": "Sometown",
"country": "USA"
}
]
}
]
Using Gson to Deserialize
To deserialize the above JSON array into a Java List of Person
objects, we can use the following code:
Gson gson = new Gson();
Type personListType = new TypeToken<List<Person>>() {}.getType();
List<Person> personList = gson.fromJson(jsonArrayString, personListType);
In the above code:
- We create a Gson instance to work with JSON data.
- We define a
Type
usingTypeToken
to represent a list ofPerson
objects. - We use
fromJson
method to deserialize the JSON array string into a List ofPerson
objects.
Handling Nested Objects
Gson automatically handles the deserialization of nested objects as long as the structure of the JSON matches the structure of the Java objects. In our example, the Person
class has a List
of Address
objects, and Gson will handle the deserialization of this nested list of objects.
By using this approach, we can seamlessly handle complex JSON structures with nested objects and arrays, allowing us to work with the data in a familiar Java object-oriented manner.
The Closing Argument
In this blog post, we've explored how to use Gson to convert a JSON array into a Java List of objects, specifically when dealing with nested objects. We've seen how to define the Java objects, deserialize the JSON array, and handle nested objects effortlessly using Gson.
Gson simplifies the process of working with JSON data in Java and provides a powerful and flexible way to handle complex JSON structures. By understanding the nuances of deserialization and leveraging the capabilities of Gson, you can efficiently work with JSON data in your Java applications.
If you're looking for a robust and efficient way to handle JSON data in your Java applications, Gson is undoubtedly a valuable tool to consider.
Start deserializing nested JSON objects into Java objects with ease using Gson, and empower your applications to seamlessly work with JSON data!
Remember, Gson makes JSON handling in Java a breeze!