Strategically Navigating MVP Development
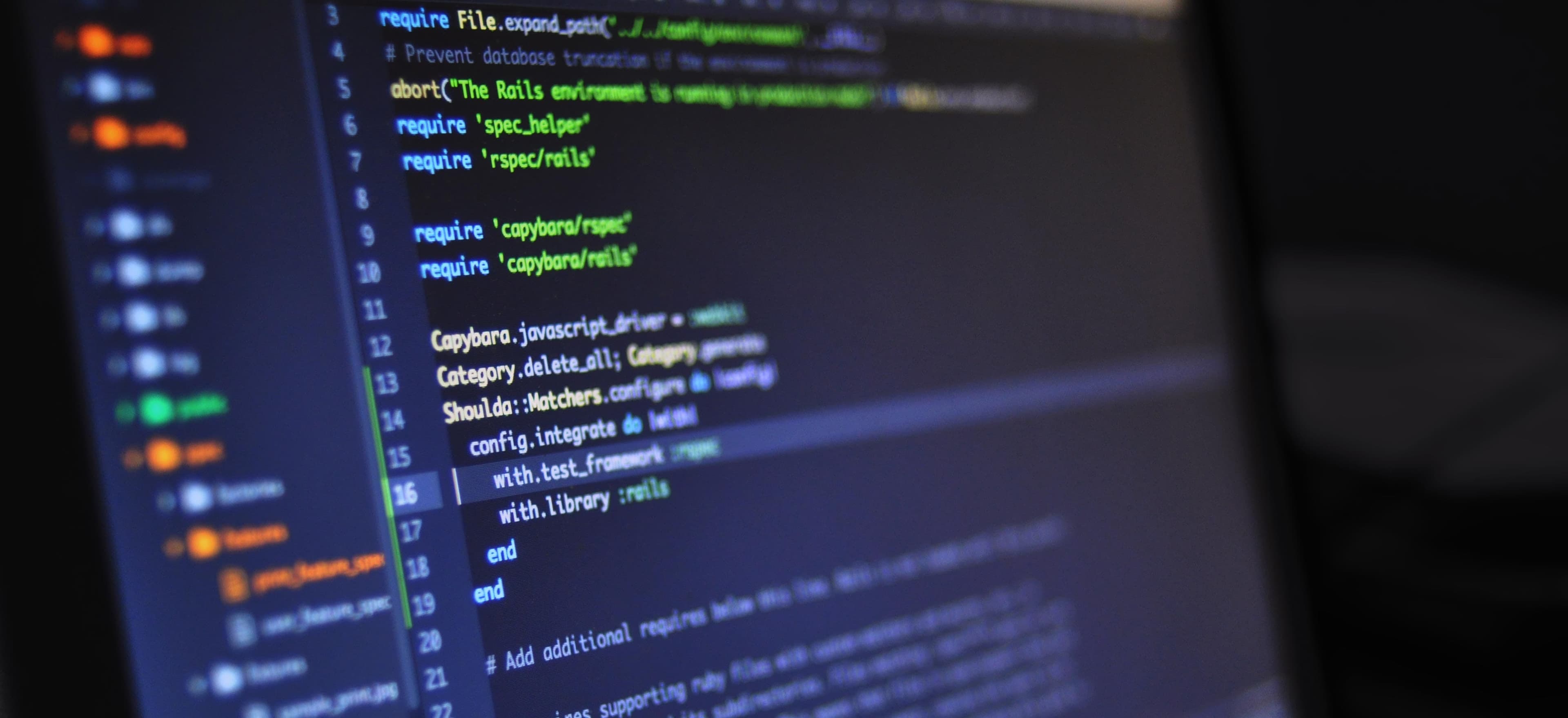
- Published on
Strategically Navigating MVP Development
In the realm of software development, especially with Java, the Model-View-Presenter (MVP) architectural pattern has gained significant popularity due to its ability to segregate the user interface into three interconnected components. This separation of concerns not only enhances maintainability but also facilitates the testing process and promotes a more organized codebase.
In this article, we will delve into the core principles and advantages of MVP architecture in Java, explore its key components, and illustrate its application with concrete examples. By the end, you will have a comprehensive understanding of how to strategically navigate MVP development to create robust, scalable, and maintainable Java applications.
Understanding MVP Architecture
MVP is based on the Model-View-Controller (MVC) pattern and stands for Model-View-Presenter. It aims to separate the responsibilities of presenting the data to the user from the data itself, providing a clear separation between application logic and the user interface. This clear separation makes the codebase more maintainable, testable, and easier to understand.
Key Components of MVP
-
Model: This component represents the data and the business logic of the application. It encapsulates the state and behavior of the application, serving as the core of the MVP architecture.
-
View: The view is responsible for presenting the user interface to the user. It observes the model and updates itself accordingly, without containing any application logic.
-
Presenter: The presenter acts as an intermediary between the model and the view. It retrieves the data from the model, processes it, and updates the view accordingly. The presenter also handles user input and triggers the appropriate actions in the model.
Advantages of MVP Architecture in Java
Implementing MVP architecture in Java offers several advantages that contribute to the overall robustness and scalability of the application.
-
Testability: MVP separates the concerns of the application, making it easier to write unit tests for the presenter and model components, as they are not directly tied to the user interface.
-
Maintainability: With a clear separation of concerns, it becomes easier to maintain and extend the codebase. Changes in one component, such as the model, do not directly impact the other components, leading to a more maintainable codebase.
-
Scalability: MVP architecture allows for better scalability as new features can be added without impacting the existing codebase. Each component can be modified or extended independently, making the application more adaptable to change.
Implementing MVP Architecture in Java
Let's demonstrate the implementation of MVP architecture in a simple Java application. We will create a basic user login functionality to illustrate the interaction between the model, view, and presenter.
Model
public class UserModel {
private String username;
private String password;
public boolean authenticateUser(String username, String password) {
// Business logic for user authentication
return username.equals("admin") && password.equals("password");
}
}
The UserModel
encapsulates the user data and provides a method to authenticate the user based on the provided credentials.
View
import java.util.Scanner;
public class UserView {
private UserPresenter presenter;
public void setPresenter(UserPresenter presenter) {
this.presenter = presenter;
}
public void requestUserCredentials() {
Scanner scanner = new Scanner(System.in);
System.out.print("Enter username: ");
String username = scanner.next();
System.out.print("Enter password: ");
String password = scanner.next();
presenter.authenticateUser(username, password);
}
public void displayAuthenticationResult(boolean isAuthenticated) {
if (isAuthenticated) {
System.out.println("User authenticated successfully");
} else {
System.out.println("Authentication failed");
}
}
}
The UserView
interacts with the user, prompts for user credentials, and displays the authentication result.
Presenter
public class UserPresenter {
private UserModel model;
private UserView view;
public UserPresenter(UserModel model, UserView view) {
this.model = model;
this.view = view;
this.view.setPresenter(this);
}
public void authenticateUser(String username, String password) {
boolean isAuthenticated = model.authenticateUser(username, password);
view.displayAuthenticationResult(isAuthenticated);
}
}
The UserPresenter
acts as the intermediary between the UserModel
and UserView
, coordinating the authentication process.
In this example, the model encapsulates the user data and authentication logic, the view interacts with the user, and the presenter orchestrates the interaction between the model and view.
Key Takeaways
In conclusion, MVP architecture offers a structured approach to developing Java applications, promoting code maintainability, testability, and scalability. By separating the concerns of the application into model, view, and presenter components, developers can navigate the development process strategically, leading to more robust and maintainable software.
When embarking on Java application development, considering the implementation of MVP architecture can significantly enhance the overall quality and reliability of the software. By adhering to the principles of MVP, you can build Java applications that are not only efficient and scalable but also easier to maintain and extend.
Now equipped with a solid understanding of MVP architecture in Java, you are well-prepared to leverage this powerful pattern in your future Java development endeavors.
For more in-depth examples and advanced practices, consider exploring the official Java documentation and industry best practices from Java developers.