Optimizing Task Scheduling in Android
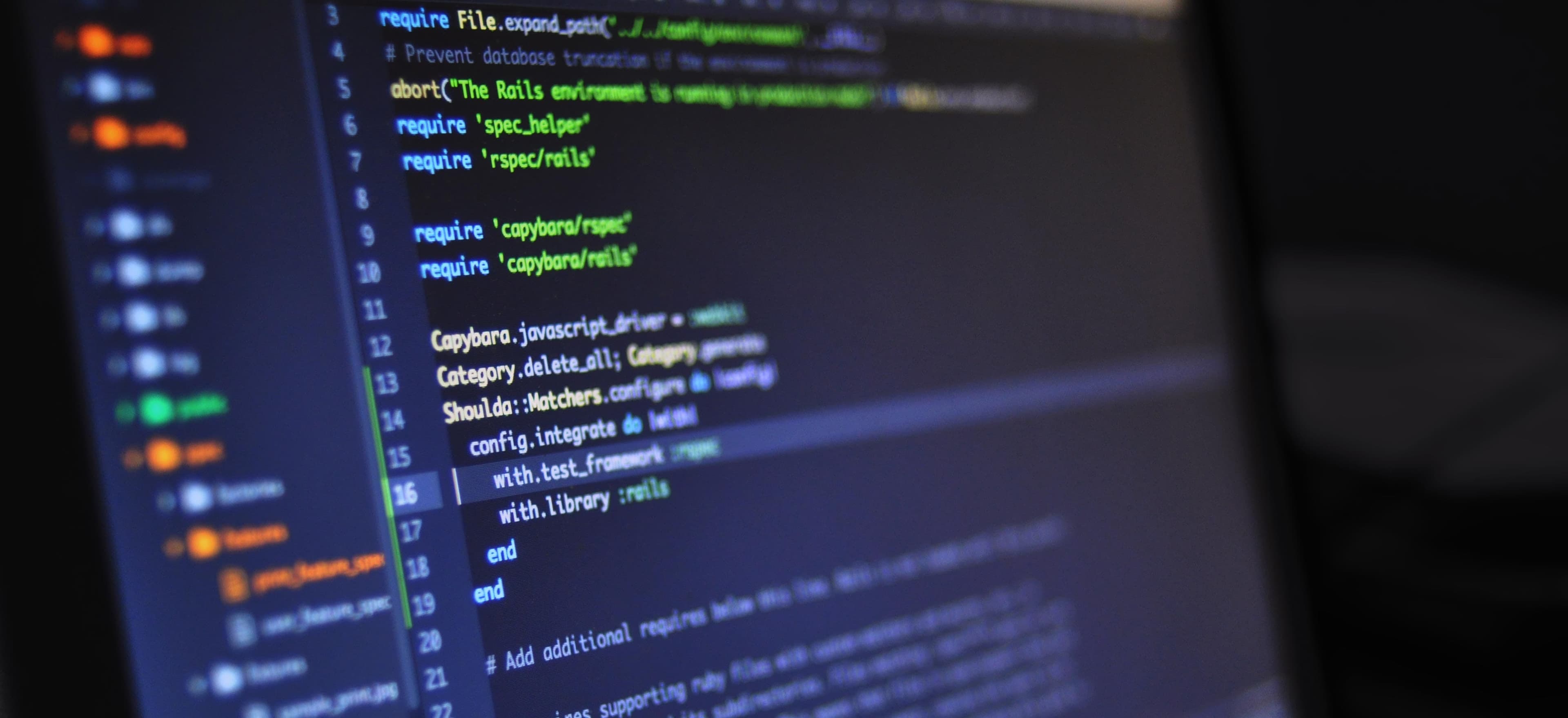
- Published on
Optimizing Task Scheduling in Android: A Comprehensive Guide
When developing Android applications, efficient task scheduling is crucial for achieving optimal performance and responsiveness. In this guide, we'll explore various techniques and best practices for optimizing task scheduling in Android applications using Java.
Understanding Task Scheduling
Task scheduling involves managing the execution of asynchronous tasks within an Android application. This includes tasks such as network requests, database operations, and UI updates. Efficient task scheduling ensures that the application remains responsive and performs well across a wide range of devices.
Using AsyncTask for Asynchronous Operations
In Android, the AsyncTask
class is a popular choice for performing background operations and updating the UI. However, when used incorrectly, AsyncTask
can lead to memory leaks and inconsistent behavior. It's essential to use AsyncTask
judiciously and consider alternative approaches for more complex use cases.
private class MyTask extends AsyncTask<Void, Void, String> {
@Override
protected String doInBackground(Void... params) {
// Perform background task
return result;
}
@Override
protected void onPostExecute(String result) {
// Update UI with the result
}
}
Leveraging Executors and ThreadPool for Concurrency
When dealing with multiple concurrent tasks, using the Executor
framework and thread pools can significantly improve performance and resource management. By leveraging executors, developers can control the number of concurrent tasks, reuse threads, and manage task dependencies.
ExecutorService executor = Executors.newFixedThreadPool(5);
executor.submit(() -> {
// Perform task
});
Implementing Task Prioritization with PriorityQueues
In certain scenarios, it's essential to prioritize tasks based on their urgency or importance. By using a PriorityQueue
along with a custom Comparable
implementation, tasks can be dynamically prioritized and executed accordingly.
Queue<Runnable> taskQueue = new PriorityQueue<>(10, (task1, task2) -> {
// Compare task priorities
});
Utilizing Android's Handler and Looper for Message Passing
Android's Handler
and Looper
classes play a pivotal role in facilitating message passing and delayed execution within the application's main thread. This mechanism is instrumental in updating the UI from background threads and executing tasks at specific intervals.
Handler handler = new Handler(Looper.getMainLooper());
handler.postDelayed(() -> {
// Execute delayed task
}, 1000);
Managing Task Lifecycle with AsyncTaskLoader
For managing long-running tasks tied to the Android lifecycle (such as activity or fragment), the AsyncTaskLoader
provides a convenient solution. It enables seamless execution and management of tasks across configuration changes and ensures proper resource cleanup.
public class MyLoader extends AsyncTaskLoader<String> {
@Override
public String loadInBackground() {
// Perform background task
return result;
}
}
A Final Look
Efficient task scheduling is fundamental to the overall performance and user experience of Android applications. By utilizing the right tools and techniques such as AsyncTask
, executors, message passing, and task prioritization, developers can optimize task scheduling and create responsive, high-performing Android applications.
In conclusion, mastering task scheduling in Android involves a combination of understanding the available options and choosing the most appropriate approach for the specific requirements of the application.
For further insights into Android task scheduling and concurrency, refer to the official Android Developer Documentation and the Android Performance Patterns resources.
Remember, efficient task scheduling leads to responsive and performant applications, ultimately enhancing the user experience.