Improving Code Readability with Functional Style
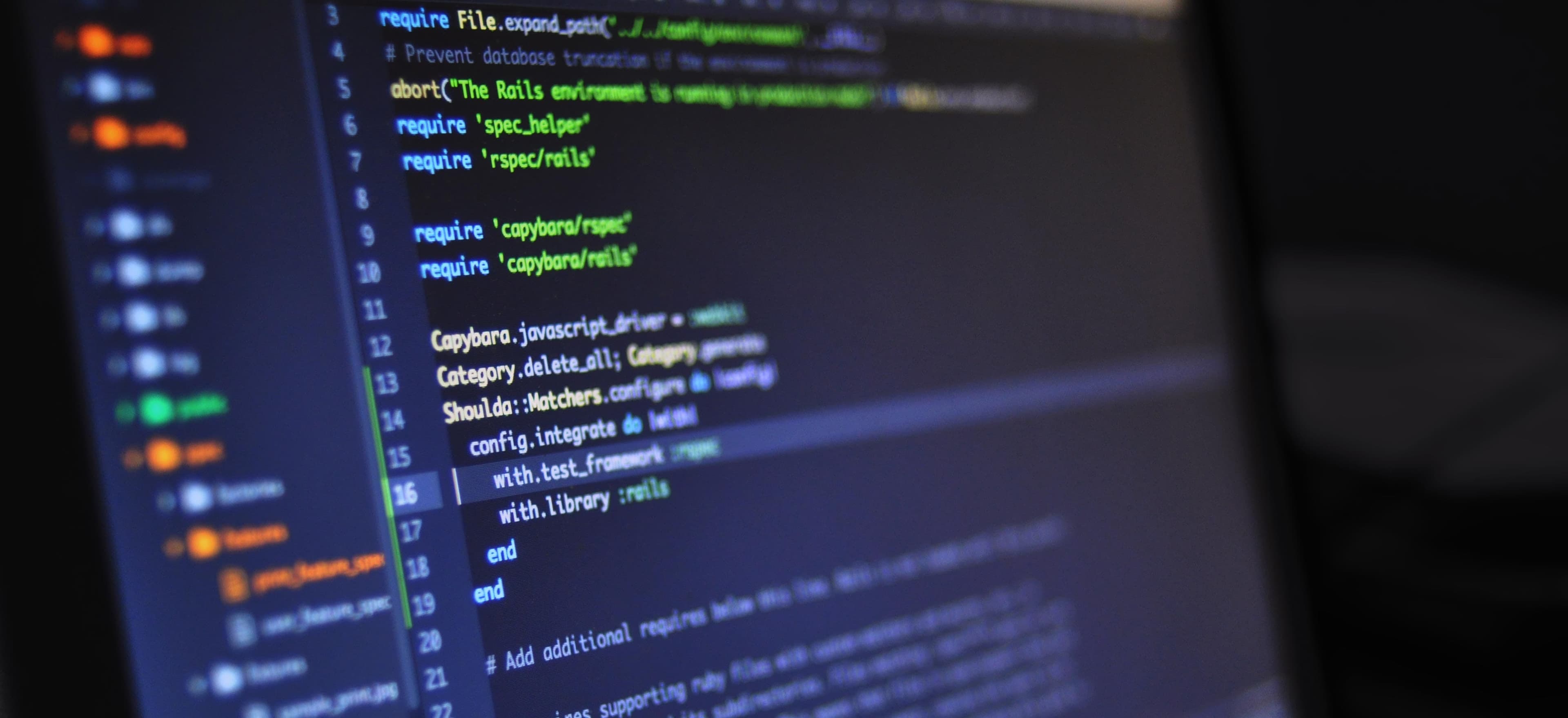
- Published on
Improving Code Readability with Functional Style
When it comes to writing clean, maintainable Java code, readability is crucial. Code that is easy to read not only helps developers understand the logic more quickly but also makes it easier to spot and fix bugs. One way to achieve better readability in Java code is by utilizing a functional programming style. In this article, we'll explore how to improve the readability of your Java code using functional techniques.
Why Functional Style?
Functional programming focuses on writing code in a way that minimizes mutable state and emphasizes the use of functions as first-class citizens. This style of programming can lead to code that is easier to reason about, as it tends to be more declarative and less imperative. By embracing functional style, you can write code that is concise, predictable, and easier to test.
Leveraging Lambda Expressions
Java 8 introduced lambda expressions, which allow you to treat functionality as a method argument or code as data. This feature enables a more functional style of programming by supporting the use of higher-order functions. Here's an example of how lambda expressions can improve the readability of your code:
List<String> names = Arrays.asList("Alice", "Bob", "Charlie", "Dave");
// Imperative style
for (String name : names) {
if (name.length() > 4) {
System.out.println(name);
}
}
// Functional style with lambda expression
names.stream()
.filter(name -> name.length() > 4)
.forEach(System.out::println);
In the imperative style, the code explicitly outlines the iteration and condition logic. On the other hand, the functional style leverages lambda expressions and method chaining to clearly express the filtering and action to be taken on the filtered elements. This makes the code more concise and readable, with the logic being expressed more directly.
Utilizing Method References
Method references, introduced in Java 8, provide a concise way to refer to methods as lambda expressions. They can be particularly useful when working with functional interfaces. Let's look at an example of how method references can enhance the readability of your code:
// Using lambda expression
List<Integer> numbers = Arrays.asList(1, 2, 3, 4, 5);
numbers.forEach(number -> System.out.println(number));
// Using method reference
numbers.forEach(System.out::println);
In this example, the method reference System.out::println
succinctly refers to the println
method of System.out
, making the code more compact and clearer. Method references are especially beneficial when working with existing methods, as they enable you to use them as lambdas without having to define the logic explicitly.
Employing Stream Operations
The Stream API, introduced in Java 8, provides a functional approach to processing collections of objects. It allows for declarative operations on data, leading to more concise and readable code. Consider the following example:
List<String> fruits = Arrays.asList("Apple", "Banana", "Cherry", "Date");
// Imperative style
List<String> upperCaseFruits = new ArrayList<>();
for (String fruit : fruits) {
upperCaseFruits.add(fruit.toUpperCase());
}
// Functional style with stream operations
List<String> upperCaseFruits = fruits.stream()
.map(String::toUpperCase)
.collect(Collectors.toList());
By using stream operations such as map
, the functional style code clearly communicates the transformation to be applied to each element of the list. This results in more readable code that better conveys the intention of the transformation.
Embracing Immutability
In functional programming, immutability is a key concept that promotes code that is easier to reason about and less error-prone. By emphasizing immutability, you can reduce unexpected side effects and make the flow of data more predictable. In Java, you can achieve immutability by using the final
keyword for variables and creating immutable data structures. Here's an example:
final List<String> colors = Arrays.asList("Red", "Green", "Blue");
// colors.add("Yellow"); // This would result in a compilation error
By declaring the colors
list as final
, you ensure that it cannot be reassigned or modified after its initial assignment, contributing to code that is more predictable and easier to understand.
Closing the Chapter
Incorporating functional programming techniques into your Java code can greatly enhance its readability. By leveraging lambda expressions, method references, stream operations, and immutability, you can write code that is clearer, more concise, and easier to maintain. Embracing a functional style can lead to more expressive, declarative code that better conveys the intent of the logic. As you continue to enhance your Java coding skills, consider exploring functional programming further and applying these techniques to improve the readability of your codebase.
By developing a deeper understanding of these concepts, you'll be well-equipped to write Java code that not only functions efficiently but is also easily comprehensible to other developers. When it comes to creating a durable and smooth codebase, readability is just as crucial as functionality. And mastering a functional-style approach in Java is a vital step on that path. So, the next time you find yourself coding in Java, remember the power of functional programming in enhancing your code's readability and maintainability.
Take your time to go through these learning resources - Functional Programming in Java and Java 8 Lambda Basics. These would further bolster your understanding of functional style in Java.