Handling Overlapping Alerts in WebDriverIO
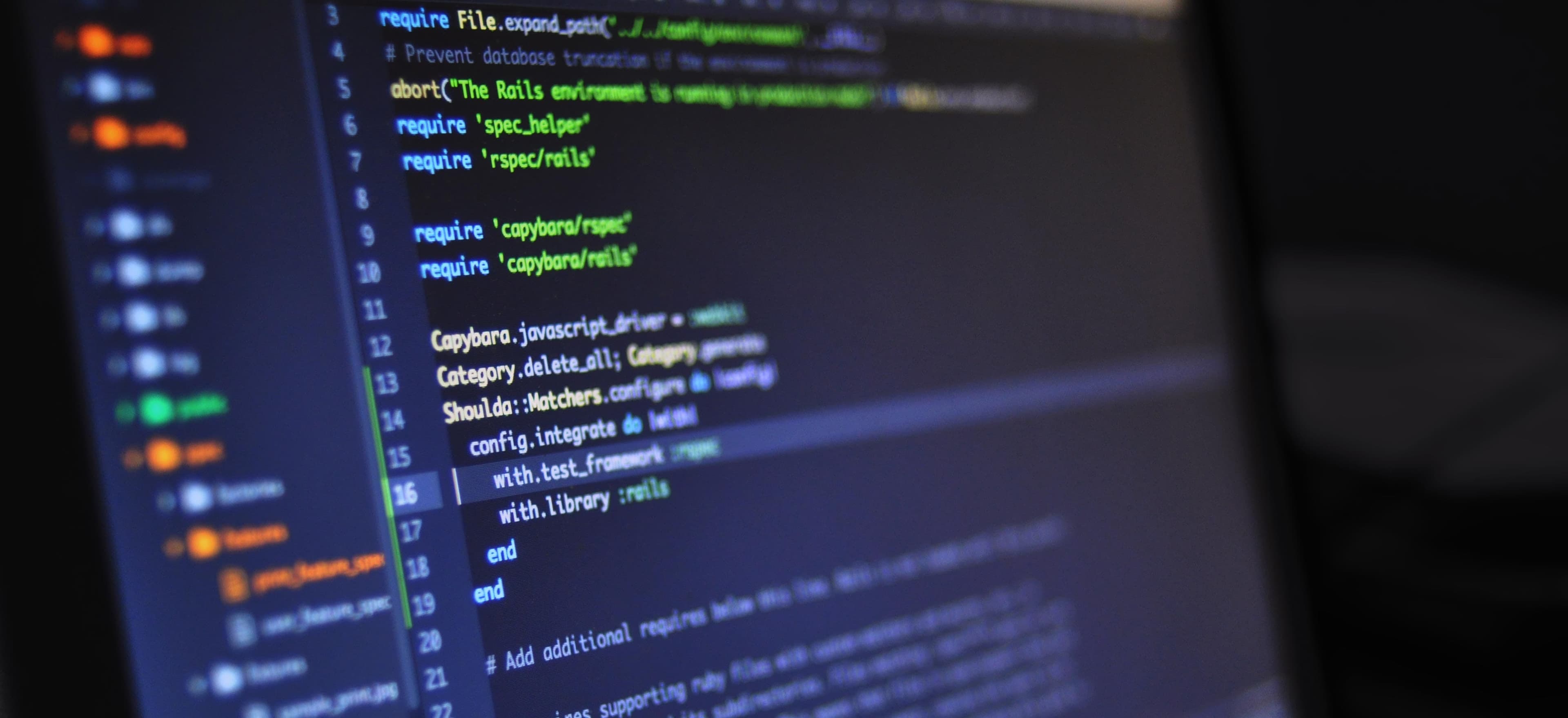
- Published on
Dealing with Overlapping Alerts in WebDriverIO
When testing web applications with WebDriverIO, you may encounter scenarios where alerts or pop-up windows appear on the page, sometimes overlapping with each other. This can pose a challenge for automated tests, as WebDriverIO needs to handle these alerts appropriately in order to interact with the underlying page elements.
In this post, we will explore how to handle overlapping alerts in WebDriverIO, discussing different strategies and providing code examples to effectively manage these situations.
Understanding Overlapping Alerts
Overlapping alerts can occur when multiple asynchronous events trigger alerts or pop-ups on the page simultaneously. This can happen during form submissions, AJAX requests, or when interacting with dynamic elements on the web page.
When dealing with WebDriverIO tests, encountering overlapping alerts can lead to unexpected behavior, causing tests to fail if not handled correctly. Therefore, it is crucial to have robust mechanisms in place to address such scenarios.
Let's dive into some strategies to handle overlapping alerts effectively in WebDriverIO.
Using alertAccept
and alertDismiss
WebDriverIO provides built-in methods to handle alerts, such as alertAccept
and alertDismiss
. These methods allow you to accept or dismiss an alert, respectively.
it('should handle overlapping alerts', () => {
// Clicking a button that triggers an alert
const triggerAlertButton = $('#trigger-alert');
triggerAlertButton.click();
// Dismissing the first alert
browser.alertDismiss();
// Clicking another button that triggers a second alert
const triggerAnotherAlertButton = $('#trigger-another-alert');
triggerAnotherAlertButton.click();
// Accepting the second alert
browser.alertAccept();
});
In the above example, we simulate a scenario where two alerts overlap. We dismiss the first alert using alertDismiss
and accept the second alert using alertAccept
, hence handling both alerts sequentially.
Working with Promises and Async/Await
When dealing with asynchronous tasks in WebDriverIO, it's essential to handle promises effectively. Using async/await
syntax can simplify the code and make it more readable.
it('should handle overlapping alerts with async/await', async () => {
const triggerAlertButton = $('#trigger-alert');
const triggerAnotherAlertButton = $('#trigger-another-alert');
// Using async/await to handle overlapping alerts
triggerAlertButton.click();
await browser.alertDismiss();
triggerAnotherAlertButton.click();
await browser.alertAccept();
});
By leveraging async/await
, the code becomes more straightforward and easier to follow, especially when dealing with multiple asynchronous alert events.
Waiting for Alerts to Appear
In certain scenarios, alerts may not appear immediately after triggering the action that prompts them. For example, when dealing with AJAX requests, there might be a delay before the alert is rendered on the page.
In such cases, it's crucial to wait for the alert to appear before attempting to interact with it. WebDriverIO provides the waitUntil
method, which can be used to wait for a specific condition to be met before proceeding with the test.
it('should wait for alerts to appear', () => {
const triggerDelayedAlertButton = $('#trigger-delayed-alert');
triggerDelayedAlertButton.click();
// Waiting for the alert to appear
browser.waitUntil(() => {
return browser.isAlertOpen();
}, 5000, 'expected alert to be present after 5s');
browser.alertAccept();
});
In this example, we wait until the alert is open by using the waitUntil
method, ensuring that the test proceeds only after the alert is visible.
Handling Overlapping Alerts with Custom Wait Strategies
When dealing with complex web applications, standard wait strategies may not always suffice. In such cases, it can be beneficial to implement custom wait strategies to handle overlapping alerts effectively.
WebDriverIO allows you to define custom wait conditions using the waitUntil
method, allowing for greater flexibility in handling alert scenarios.
it('should implement custom wait strategy for overlapping alerts', () => {
const triggerCustomAlertButton = $('#trigger-custom-alert');
triggerCustomAlertButton.click();
// Define a custom wait strategy for the alert
browser.waitUntil(() => {
const alertText = browser.getAlertText();
return alertText.includes('expected alert message');
}, 5000, 'custom alert did not appear within 5s');
browser.alertAccept();
});
In this example, we define a custom wait strategy to wait for the alert with a specific message to appear before proceeding with the test.
Closing the Chapter
Handling overlapping alerts in WebDriverIO tests is a common challenge, especially when dealing with dynamic and asynchronous web applications. By leveraging the built-in alert handling methods, working with promises and async/await, waiting for alerts to appear, and implementing custom wait strategies, you can effectively manage overlapping alerts and ensure the stability of your automated tests.
As you continue to work with WebDriverIO, being adept at handling overlapping alerts will prove invaluable in creating robust and reliable test suites for your web applications.
References:
- WebDriverIO Docs: Alert Handling
- Asynchronous JavaScript: Understanding async/await