Common Mistakes in Null Checking
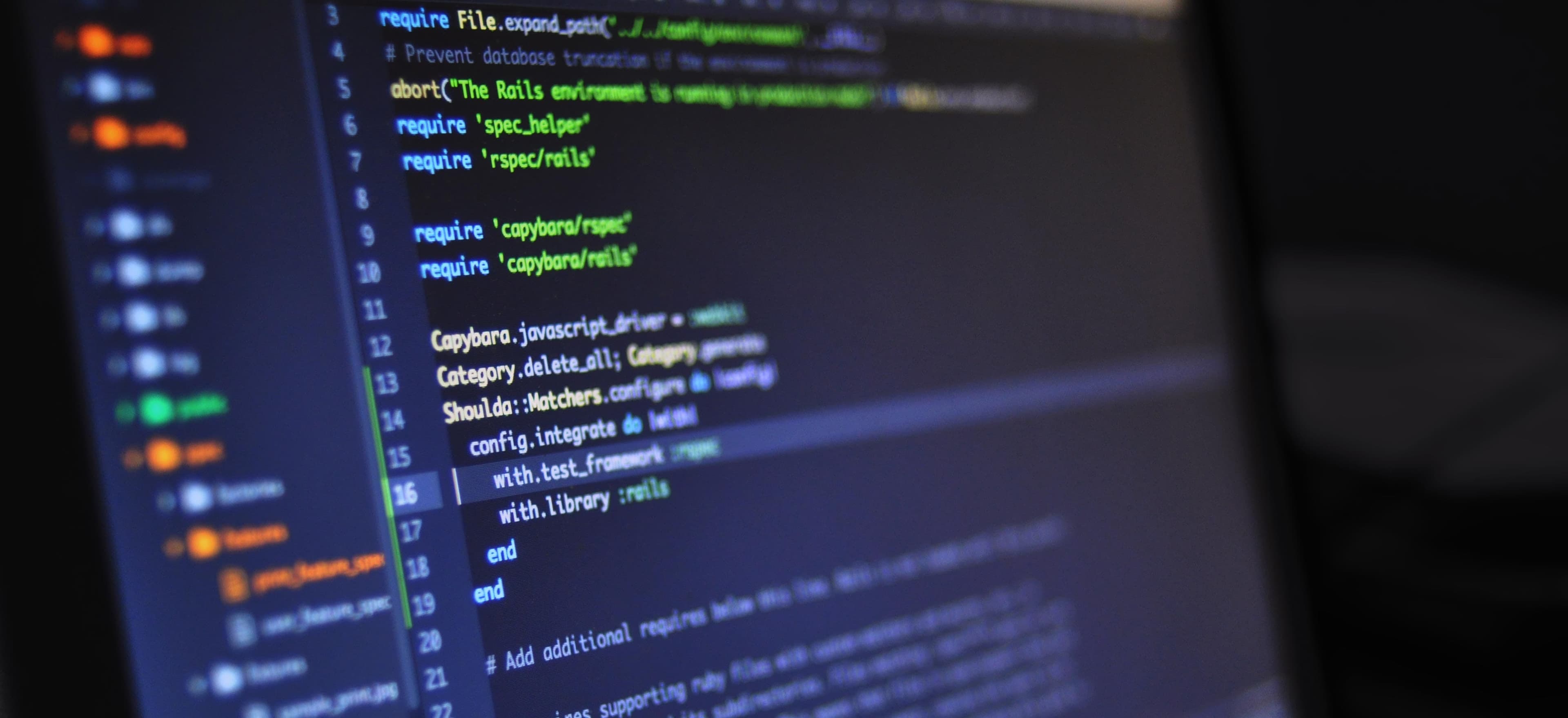
- Published on
Common Mistakes in Null Checking in Java
When writing Java code, handling null values is a common task. Failing to do so can lead to unexpected NullPointerExceptions, which can be difficult to trace and debug. Although Java provides various ways to handle null checks, there are several common mistakes that developers make. Let's dive into some of these mistakes and explore best practices for null checking in Java.
1. Using == for Object Comparison
One of the most common mistakes is using the ==
operator for comparing objects for nullity. In Java, the ==
operator checks for reference equality, not for object equality. When comparing objects for null, the correct approach is to use the equals()
method or the Objects.isNull()
method introduced in Java 7.
String str = null;
// Incorrect way using ==
if (str == null) {
// Handle null case
}
// Correct way using Objects.isNull()
if (Objects.isNull(str)) {
// Handle null case
}
The Objects.isNull()
method clearly expresses the intention of null checking and avoids the pitfall of using ==
for object comparison.
2. Not Handling Potential Null Returns
Many Java APIs return null to indicate the absence of a value. Forgetting to check for null when invoking methods that may return null can lead to NullPointerExceptions. It’s essential to ensure that proper null checks are in place when working with such methods.
List<String> list = someMethodThatMayReturnNull();
// Potential NPE if list is null
int size = list.size();
It is important to always validate the return values from such methods and handle null cases appropriately to avoid runtime exceptions.
3. Overusing Null Checks
While null checks are essential for robust code, overusing them can clutter the code and make it less readable. In some cases, excessive null checks may indicate design flaws or the need for refactoring.
if (object != null) {
if (object.getProperty() != null) {
if (object.getProperty().getValue() != null) {
// Perform operations on the value
}
}
}
In such scenarios, using the Optional class introduced in Java 8 can provide a cleaner and more expressive way to handle potentially null values.
4. Not Using Optional Correctly
Java 8 introduced the Optional class as a container that may or may not contain a non-null value. However, misusing Optional or using it excessively can lead to verbose and convoluted code.
Optional<String> optionalString = Optional.ofNullable(getString());
// Unnecessarily verbose usage of Optional
if (optionalString.isPresent()) {
String value = optionalString.get();
// Process the value
}
// Preferred way using Optional's map
optionalString.map(value -> {
// Process the value
});
It's crucial to understand the functional aspects of Optional and leverage its methods such as map
, filter
, and orElse
to write concise and expressive code.
5. Ignoring Preconditions
Ignoring preconditions can result in null pointer exceptions. For instance, passing a null argument to a method that explicitly disallows null can lead to unexpected behavior.
public void performAction(String input) {
Objects.requireNonNull(input, "Input cannot be null");
// Perform action
}
performAction(null); // Will result in NullPointerException
By ignoring preconditions, developers make their code susceptible to errors that could be easily avoided with proper null checks and validation.
Bringing It All Together
Null checking is an indispensable part of writing robust and error-free Java code. By avoiding common pitfalls such as using ==
for object comparison, neglecting potential null returns, overusing null checks, misusing Optional, and ignoring preconditions, developers can ensure their code is resilient to null pointer exceptions.
Incorporating best practices for null checking not only improves the reliability of the code but also enhances its readability and maintainability. By being mindful of these common mistakes and adhering to established conventions, developers can elevate the quality of their Java codebase and deliver more robust software solutions.
For further insights on effective Java programming practices, explore the Java section of our blog.