Mastering Selenium 2.0: Solving Compatibility Issues
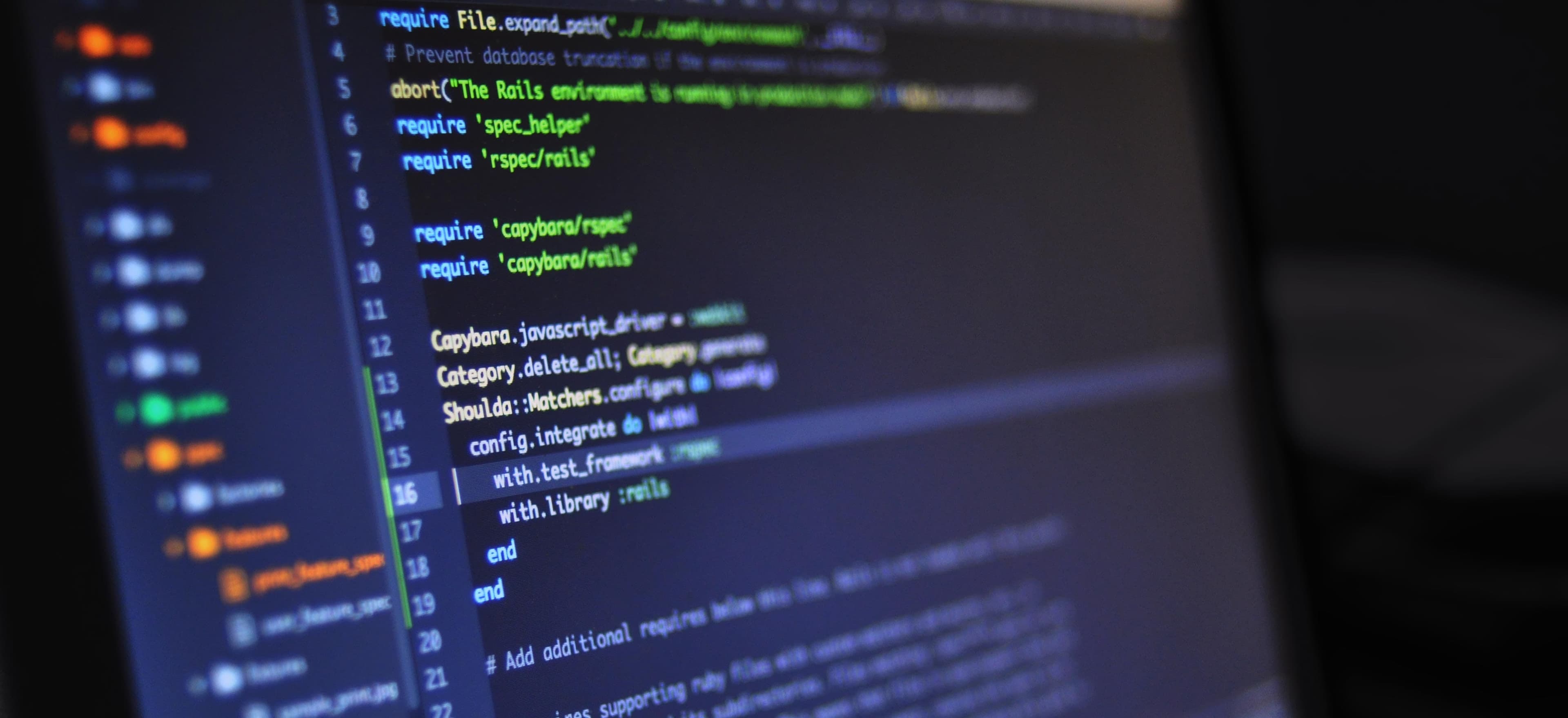
- Published on
Mastering Selenium 2.0: Solving Compatibility Issues
Selenium is a powerful tool for automating web browsers. With its wide range of functionalities, it has become a staple in the arsenal of any Java developer involved in web application testing. However, as technology moves forward, one of the challenges that Selenium developers face is ensuring compatibility with the latest web browsers and their versions. In this post, we will delve into compatibility issues with Selenium 2.0 and discuss how to solve them using Java.
Understanding Compatibility Issues
Web browsers are constantly evolving, and with each new version, they introduce changes to their internal structure, web standards support, and security protocols. This evolution often leads to compatibility issues with the older versions of Selenium. As a result, tests written using an older version of Selenium may fail to execute correctly on the latest web browsers.
Selenium WebDriver
Selenium 2.0, also known as Selenium WebDriver, is a powerful tool for automating web browsers. It provides a programming interface to drive the browser in the same way a user would. Selenium WebDriver overcomes the limitations of Selenium RC and is now the de-facto standard for web browser automation. However, this does not exempt it from compatibility issues with the latest web browsers.
Let's take a look at an example of a compatibility issue and explore the ways to resolve it.
Example: Compatibility Issue with Chrome Browser
Suppose you have a Selenium test script that was working perfectly with an older version of the Chrome browser. However, after updating the Chrome browser to the latest version, the test script starts failing. This is a classic compatibility issue between Selenium 2.0 and the latest Chrome browser version.
Resolving Compatibility Issues
Update Selenium WebDriver Dependencies
First and foremost, it's crucial to ensure that you have the latest version of Selenium WebDriver dependencies in your Java project. By updating the Selenium WebDriver dependencies, you can take advantage of the latest bug fixes, improvements, and compatibility enhancements that have been made to address issues with the latest web browsers.
<dependency>
<groupId>org.seleniumhq.selenium</groupId>
<artifactId>selenium-java</artifactId>
<version>3.141.59</version>
</dependency>
In this snippet, we see the Maven dependency for Selenium WebDriver. By specifying the latest version, we ensure that our project utilizes the most up-to-date Selenium WebDriver library.
Chrome Driver Compatibility
Once the Selenium WebDriver dependencies are updated, we need to address the compatibility of ChromeDriver, which is essential for interacting with the Chrome browser using Selenium. The ChromeDriver version should align with the Chrome browser version installed on the testing machine.
System.setProperty("webdriver.chrome.driver", "path_to_chromedriver_exe");
WebDriver driver = new ChromeDriver();
Here, we set the system property to specify the path to the ChromeDriver executable. It's crucial to use the compatible version of ChromeDriver that corresponds to the installed Chrome browser version. Failing to do so may lead to compatibility issues.
Firefox GeckoDriver Compatibility
Similar to ChromeDriver, if you are using Firefox with Selenium WebDriver, you need to ensure compatibility between GeckoDriver and the Firefox browser version installed on the testing machine.
System.setProperty("webdriver.gecko.driver", "path_to_geckodriver_exe");
WebDriver driver = new FirefoxDriver();
The snippet above demonstrates setting the system property for GeckoDriver. Just like ChromeDriver, it's imperative to use the compatible version of GeckoDriver for seamless compatibility with Firefox.
Internet Explorer Driver Compatibility
When working with Internet Explorer and Selenium WebDriver, it's essential to ensure compatibility between the Internet Explorer Driver and the installed Internet Explorer version on the testing machine.
System.setProperty("webdriver.ie.driver", "path_to_IEDriverServer_exe");
WebDriver driver = new InternetExplorerDriver();
In this code, we specify the system property for Internet Explorer Driver. Again, it's crucial to match the version of the driver with the installed Internet Explorer version to avoid compatibility issues.
In Conclusion, Here is What Matters
In this post, we've explored the significance of addressing compatibility issues with Selenium 2.0 and various web browsers. By updating Selenium WebDriver dependencies and ensuring the compatibility of browser-specific drivers such as ChromeDriver, GeckoDriver, and Internet Explorer Driver, developers can overcome compatibility issues and ensure smooth execution of Selenium tests across different web browsers and their versions. It's imperative to stay vigilant about compatibility and maintain an up-to-date Selenium environment to reap the full benefits of automated web browser testing with Selenium 2.0.
Happy testing!