Managing Java Dependencies with OSGi
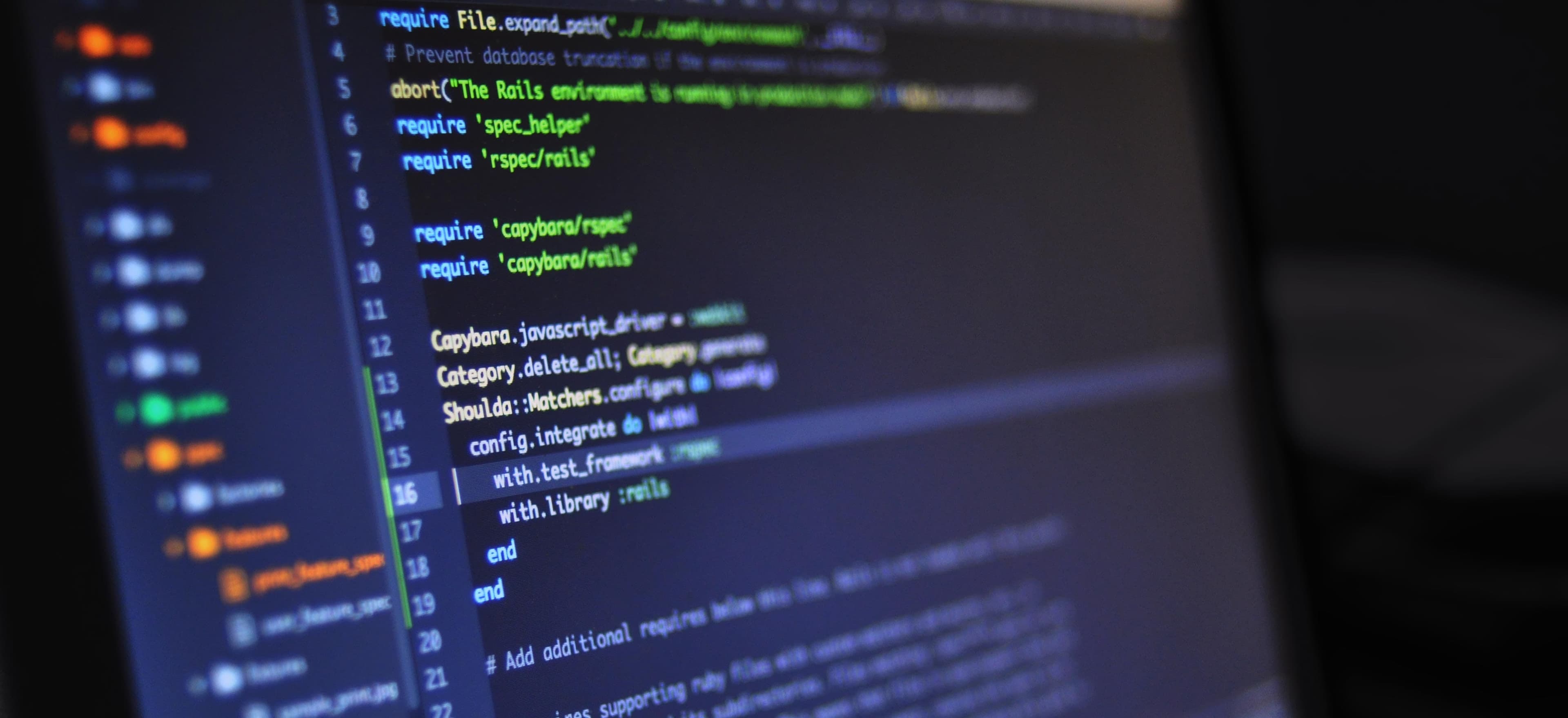
- Published on
Managing Java Dependencies with OSGi
In the world of Java development, managing dependencies is a crucial task that can make or break a project. OSGi (Open Service Gateway initiative) is a powerful modular system for Java that can help simplify the process of managing dependencies and building modular applications.
What is OSGi?
OSGi is a dynamic module system for Java that enables developers to create highly modular applications. It allows the creation of loosely coupled modules or components, each of which can be independently developed, tested, and managed. OSGi provides a runtime environment that supports the dynamic installation, update, and removal of modules.
Benefits of Using OSGi for Dependency Management
1. Modularity
OSGi promotes modularity by allowing applications to be built from small, reusable and collaborative modules. This approach makes it easier to understand, maintain, and extend complex systems.
2. Versioning
With OSGi, different versions of the same library can coexist within an application, resolving potential conflicts and enabling better management of dependencies.
3. Dynamism
OSGi supports dynamic module lifecycle, allowing modules to be installed, started, stopped, updated, and uninstalled without requiring a full application restart.
4. Service-Oriented Architecture
OSGi facilitates the development of service-oriented architectures by providing a registry for services, allowing modules to declare and consume services provided by other modules.
How to Use OSGi for Dependency Management
1. Define Modules
In OSGi, modules are defined using a manifest file, which specifies metadata about the module such as its name, version, dependencies, and exported packages. Here is an example of a simple OSGi manifest file:
Manifest-Version: 1.0
Bundle-ManifestVersion: 2
Bundle-SymbolicName: com.example.mybundle
Bundle-Version: 1.0.0
Import-Package: com.example.dependency;version="1.0.0"
Export-Package: com.example.mybundle.api
In this example, Import-Package
specifies the dependencies required by the module, while Export-Package
specifies the packages that the module makes available to other modules.
2. Use a Bundle Repository
A bundle repository is a central location where OSGi modules are stored and managed. It provides a way to discover, download, and install modules as needed. Apache Felix and Eclipse Equinox are popular implementations of OSGi bundle repositories.
3. Service Registry
OSGi provides a service registry that allows modules to publish services and other modules to discover and consume those services. This promotes a loosely-coupled, service-oriented architecture within OSGi applications.
OSGi in Action
Let's take a look at a simple example to demonstrate how OSGi can manage dependencies in a Java application.
Suppose we have two OSGi modules: com.example.consumer
and com.example.provider
. The com.example.consumer
module depends on the services provided by the com.example.provider
module.
Provider Module
// com.example.provider.ProviderService.java
package com.example.provider;
public interface ProviderService {
void doSomething();
}
// com.example.provider.internal.ProviderServiceImpl.java
package com.example.provider.internal;
import com.example.provider.ProviderService;
public class ProviderServiceImpl implements ProviderService {
public void doSomething() {
// Implementation
}
}
// MANIFEST.MF
Manifest-Version: 1.0
Bundle-ManifestVersion: 2
Bundle-SymbolicName: com.example.provider
Bundle-Version: 1.0.0
Export-Package: com.example.provider
Consumer Module
// com.example.consumer.ConsumerService.java
package com.example.consumer;
public interface ConsumerService {
void useProviderService();
}
// com.example.consumer.internal.ConsumerServiceImpl.java
package com.example.consumer.internal;
import com.example.provider.ProviderService;
import com.example.consumer.ConsumerService;
public class ConsumerServiceImpl implements ConsumerService {
private ProviderService providerService;
// OSGi will inject the ProviderService implementation
public void setProviderService(ProviderService providerService) {
this.providerService = providerService;
}
public void useProviderService() {
providerService.doSomething();
}
}
// MANIFEST.MF
Manifest-Version: 1.0
Bundle-ManifestVersion: 2
Bundle-SymbolicName: com.example.consumer
Bundle-Version: 1.0.0
Import-Package: com.example.provider;version="[1.0.0,2.0.0)"
In this example, the com.example.consumer
module imports the com.example.provider
package and consumes the ProviderService
provided by the com.example.provider
module. This allows for loose coupling between the two modules and enables dynamic service discovery at runtime.
Closing the Chapter
OSGi provides a robust solution for managing Java dependencies through its modular architecture, versioning support, dynamic lifecycle, and service-oriented capabilities. By embracing OSGi, developers can build flexible and scalable applications that are easier to maintain and extend.
In summary, OSGi offers a powerful way to manage Java dependencies, promoting modularity, versioning, dynamism, and service-oriented architecture within applications. Embracing OSGi can lead to more manageable and robust software systems.
Incorporating OSGi into your Java projects can streamline dependency management and foster a modular, scalable, and maintainable codebase.
For further exploration, Apache Felix and Eclipse Equinox are two popular OSGi implementations that provide valuable insights into OSGi-based development and dependency management.
By leveraging OSGi, developers can gain a competitive edge in building robust, scalable, and maintainable Java applications. Start exploring OSGi today and experience the benefits of streamlined dependency management in action.
Checkout our other articles