Heap Memory vs ByteBuffer vs Direct Memory: Performance Comparison
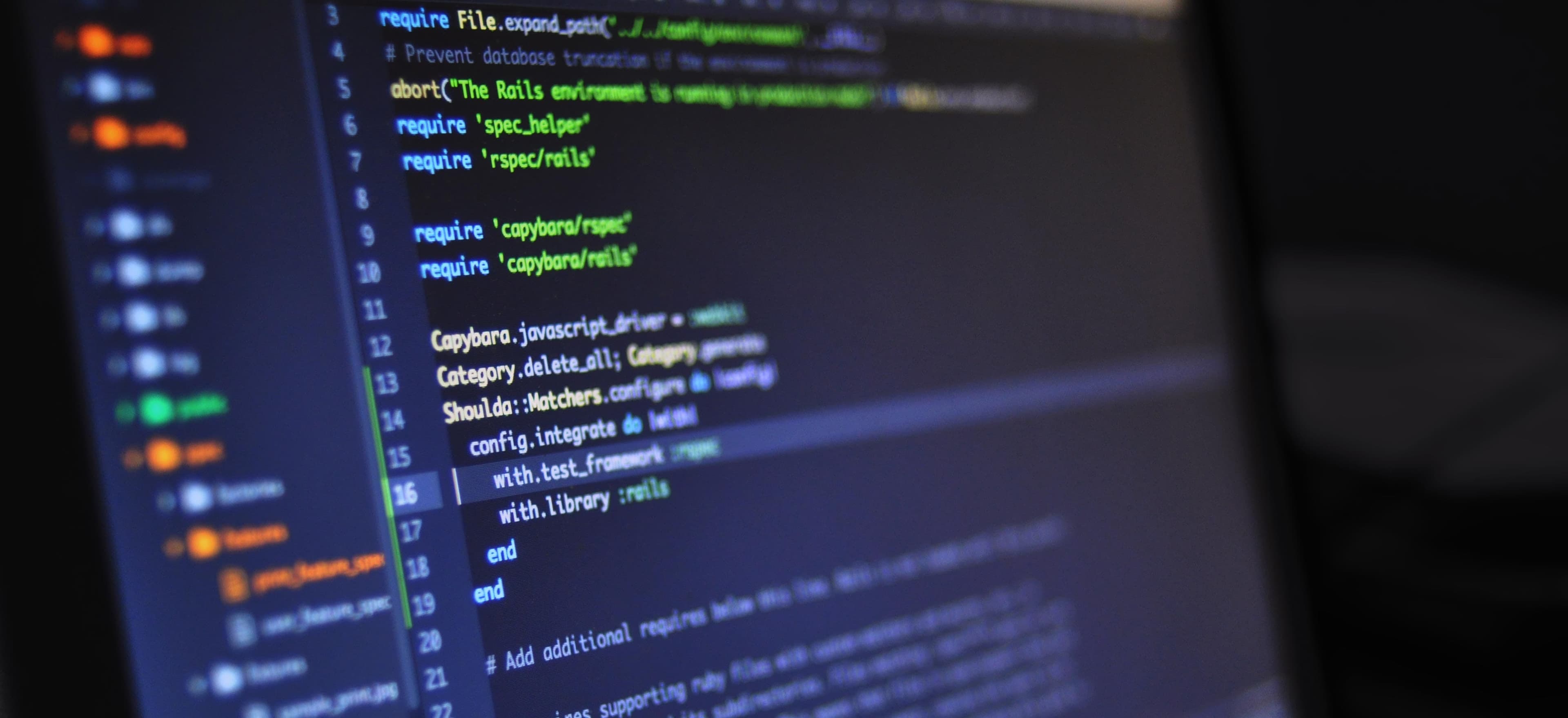
- Published on
Heap Memory vs ByteBuffer vs Direct Memory: Performance Comparison
When working with Java, memory management is a crucial aspect of ensuring the performance of an application. Java provides various options for managing memory, including heap memory, ByteBuffer, and direct memory. In this article, we will compare the performance of these memory management options to understand their strengths and weaknesses.
Heap Memory
Heap memory is the default memory space allocated to a Java program for storing objects, arrays, and other data structures. It is managed by the Java Virtual Machine (JVM) and is subject to garbage collection, which can lead to occasional pauses in the application as the JVM reclaims unused memory.
Heap memory is suitable for most general-purpose use cases, as it provides automatic memory allocation and deallocation. However, it may not be the most efficient option for certain performance-critical applications.
ByteBuffer
ByteBuffer is a part of the NIO (New I/O) package introduced in Java to provide an alternative to standard I/O operations. It allows for direct manipulation of memory and is particularly useful for handling I/O operations for networking, file I/O, and other data manipulation tasks.
ByteBuffer allows for efficient read and writes operations on memory, and it's often used in networking and high-performance data processing scenarios. It provides better control over memory management compared to heap memory, and it can be more efficient in certain use cases.
Direct Memory
Direct memory is a special type of memory provided by Java through the java.nio package. It allows for memory allocation outside of the JVM's heap, providing direct access to native memory. This can be beneficial for scenarios where direct interaction with native code or libraries is required.
Direct memory avoids the overhead of garbage collection and can offer improved performance for certain types of applications, such as those involving extensive data processing or interacting with native code.
Performance Comparison
To compare the performance of heap memory, ByteBuffer, and direct memory, let's consider a scenario involving intensive read and write operations on a large dataset. We will examine the impact of each memory management option on the application's throughput and resource utilization.
Example: Reading and Writing Data
Let's start by looking at how each memory management option handles reading and writing data. In this example, we will use a large dataset and measure the time it takes to perform read and write operations using heap memory, ByteBuffer, and direct memory.
// Sample code for reading and writing data using heap memory
byte[] data = new byte[1000000];
// Perform read and write operations using heap memory
// Sample code for reading and writing data using ByteBuffer
ByteBuffer buffer = ByteBuffer.allocate(1000000);
// Perform read and write operations using ByteBuffer
// Sample code for reading and writing data using direct memory
ByteBuffer directBuffer = ByteBuffer.allocateDirect(1000000);
// Perform read and write operations using direct memory
In the above examples, we can see how each memory management option is utilized for reading and writing data. The key difference lies in how the memory is allocated and accessed, which can have a significant impact on performance.
Throughput and Resource Utilization
To evaluate the performance of heap memory, ByteBuffer, and direct memory, we need to consider factors such as throughput and resource utilization. Throughput refers to the rate at which the application can process data, while resource utilization accounts for the memory and CPU usage.
By measuring the throughput and resource utilization of the application when using each memory management option, we can identify their respective performance characteristics and determine which option is best suited for a given use case.
Key Takeaways
In conclusion, choosing the right memory management option in Java is crucial for maximizing the performance of an application. While heap memory provides automatic memory management and is suitable for general use cases, ByteBuffer and direct memory offer more control and can be more efficient for specific use cases, such as networking, data processing, and native code interaction.
Understanding the performance implications of each memory management option is essential for making informed decisions when designing and optimizing Java applications.
In summary, heap memory, ByteBuffer, and direct memory offer different trade-offs in terms of performance and resource utilization. By carefully considering the requirements of a given application, developers can choose the most suitable memory management option to achieve the desired performance outcomes.
By understanding the strengths and weaknesses of each memory management option, developers can make informed decisions to optimize the performance of their Java applications.
In this article, we have explored the performance comparison of heap memory, ByteBuffer, and direct memory in Java, highlighting their respective strengths and use cases. By considering the specific requirements of an application, developers can make informed decisions about the most suitable memory management option for optimal performance.
To delve deeper into memory management in Java, you can explore Oracle's official documentation on ByteBuffer and Understanding Garbage Collection in Java for further insights.
In conclusion, understanding the performance characteristics of heap memory, ByteBuffer, and direct memory is essential for making informed decisions about memory management in Java and optimizing the performance of applications. Each option has its strengths and weaknesses, and choosing the right one depends on the specific requirements of the application.