Troubleshooting Java Heap Dump Generation Issues
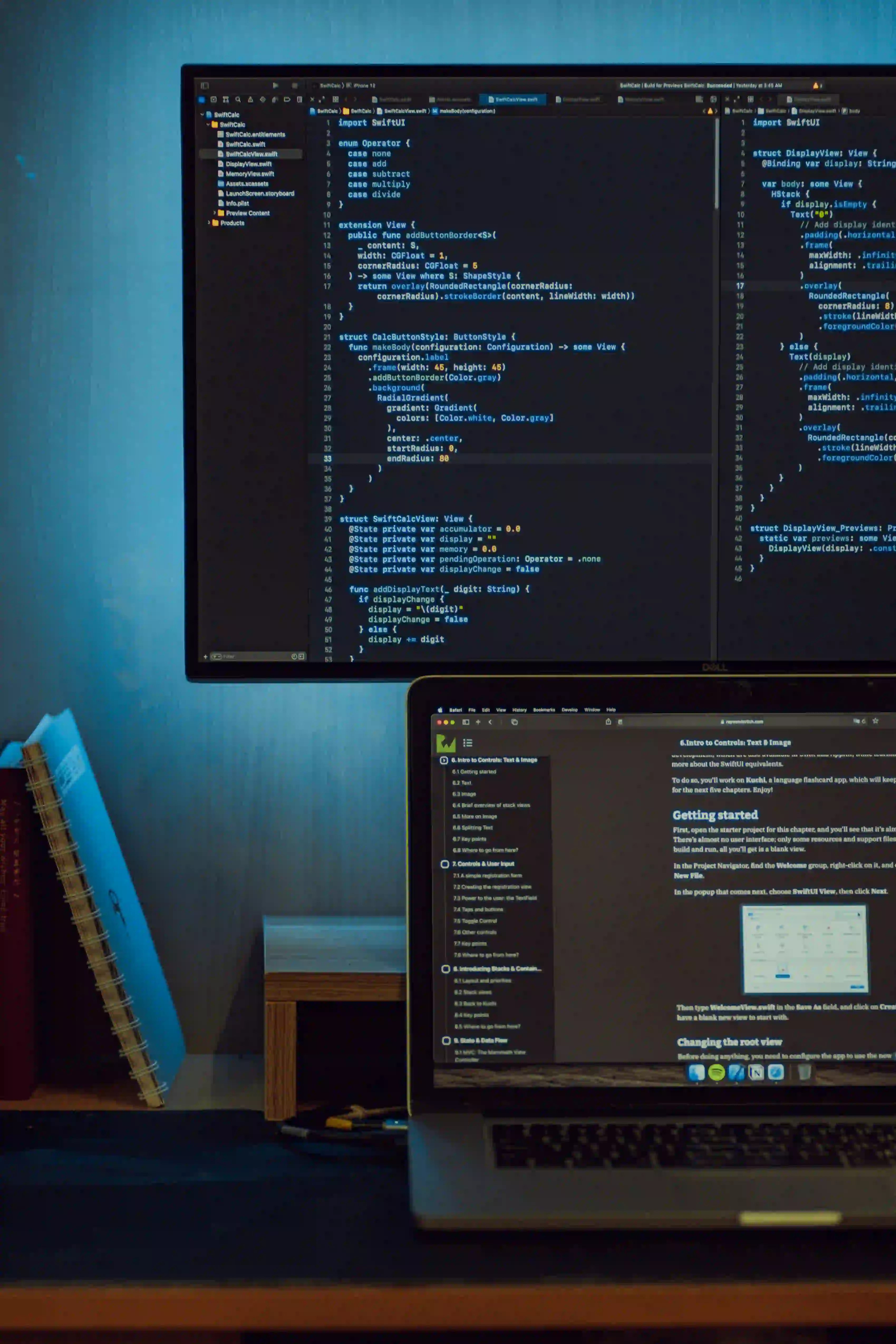
Troubleshooting Java Heap Dump Generation Issues
Java applications often encounter memory-related issues, and when they do, generating a heap dump becomes crucial for diagnosing and resolving these problems. A heap dump is a snapshot of the Java Virtual Machine's memory and provides valuable insights into memory usage, object retention, and potential memory leaks.
In this article, we'll explore the common issues encountered when generating heap dumps in Java applications and provide troubleshooting steps to overcome these challenges.
Understanding Heap Dump Generation
Before diving into troubleshooting, it's essential to understand how heap dumps are generated in Java applications. A heap dump can be triggered programmatically, or through tools like JConsole, JVisualVM, or by sending specific signals to the JVM using tools like jcmd
or jmap
.
When a heap dump is generated, the JVM captures the state of the heap, including live and unreachable objects, and writes this information to a file in a binary format.
Common Issues and Troubleshooting
1. Insufficient Permissions
Issue: When attempting to generate a heap dump, the JVM may encounter permission-related errors, especially in environments with restricted access.
Troubleshooting:
- Ensure that the user running the Java process has the necessary permissions to write files to the specified directory.
- If the process is running as a service, verify the permissions of the service account.
- Consider using a location with broad write permissions for testing purposes, such as the user's home directory.
2. Out of Memory Errors
Issue: In scenarios where the application is experiencing memory exhaustion, attempting to generate a heap dump may exacerbate the problem and lead to an out of memory error.
Troubleshooting:
- Use the
-XX:OnOutOfMemoryError
JVM option to specify a script or command to run when an out of memory error occurs. This can be used to automatically trigger a heap dump when memory exhaustion is detected. - Increase the available memory for the application if possible to prevent out of memory errors during heap dump generation.
3. Unresponsive Application During Dump Generation
Issue: Generating a heap dump can be a resource-intensive operation, potentially causing the application to become temporarily unresponsive or slow to process requests.
Trouleshooting:
- Consider using tools that allow for non-disruptive heap dump generation, such as JVisualVM, which can connect to a running application and trigger a heap dump without causing significant performance impact.
- Schedule heap dump generation during off-peak hours or low-traffic periods to minimize disruption to end users.
4. Large Heap Sizes and Prolonged Dump Generation
Issue: Applications with large heap sizes may experience prolonged heap dump generation times, leading to extended periods of increased resource usage.
Troubleshooting:
- Utilize the
-F
option withjmap
to force the heap dump generation, which may help speed up the process at the cost of potentially increased disruption to the application. - Consider using specialized profiling tools like YourKit or Java Mission Control for capturing heap dumps in high-traffic or large-scale applications, as they may offer more efficient heap analysis capabilities.
Best Practices for Efficient Heap Dump Generation
In addition to troubleshooting specific issues, following best practices can streamline the heap dump generation process and minimize potential disruptions to the application.
1. Selective Heap Dump Generation
Instead of capturing the entire heap, focus on generating targeted heap dumps for specific memory areas or suspected memory leak locations. Tools like jmap -histo:live
, which provides a histogram of live objects, or YourKit's selective memory dump feature can aid in this approach.
2. Monitor and Automate Heap Dumps
Implement automated monitoring for memory usage and set up alerts for predefined thresholds. This enables proactive heap dump generation based on actual memory consumption patterns, allowing for early detection and analysis of potential memory-related issues.
3. Heap Dump Analysis and Profiling
Consider using advanced heap analysis and profiling tools to gain deeper insights into heap usage patterns, object retention, and memory leaks. Tools like Eclipse Memory Analyzer (MAT) or VisualVM's heap walker can assist in analyzing heap dumps and identifying problematic memory consumption patterns.
Example: Triggering Heap Dump Programmatically
Let's explore a simple example of programmatically triggering a heap dump in a Java application using the HotSpotDiagnosticMXBean
:
import java.lang.management.ManagementFactory;
import com.sun.management.HotSpotDiagnosticMXBean;
public class HeapDumpExample {
public static void main(String[] args) {
String filePath = "/path/to/heapdump.hprof";
HotSpotDiagnosticMXBean diagnosticMXBean =
ManagementFactory.getPlatformMXBean(HotSpotDiagnosticMXBean.class);
try {
diagnosticMXBean.dumpHeap(filePath, true);
System.out.println("Heap dump generated successfully at: " + filePath);
} catch (Exception e) {
e.printStackTrace();
}
}
}
In this example, we obtain an instance of the HotSpotDiagnosticMXBean
and use its dumpHeap
method to generate a heap dump at the specified file path.
The Closing Argument
Troubleshooting heap dump generation issues in Java applications requires a combination of understanding the underlying mechanisms of heap dumps, addressing common challenges, and adopting best practices for efficient heap dump generation and analysis.
By identifying and overcoming obstacles related to heap dump generation, developers and operators can leverage the valuable insights provided by heap dumps to diagnose and resolve memory-related issues, ultimately improving the stability and performance of Java applications.
Remember, effective troubleshooting and proactive heap dump generation are essential components of robust memory management in Java applications.
For further reading, Oracle's documentation on Java Troubleshooting provides in-depth insights into diagnosing and resolving various issues in Java applications.
Have you encountered challenges with heap dump generation in Java? What troubleshooting techniques have you found helpful? Let's continue the conversation!