Understanding the Ambiguity: Java 8 Default Method Conflict
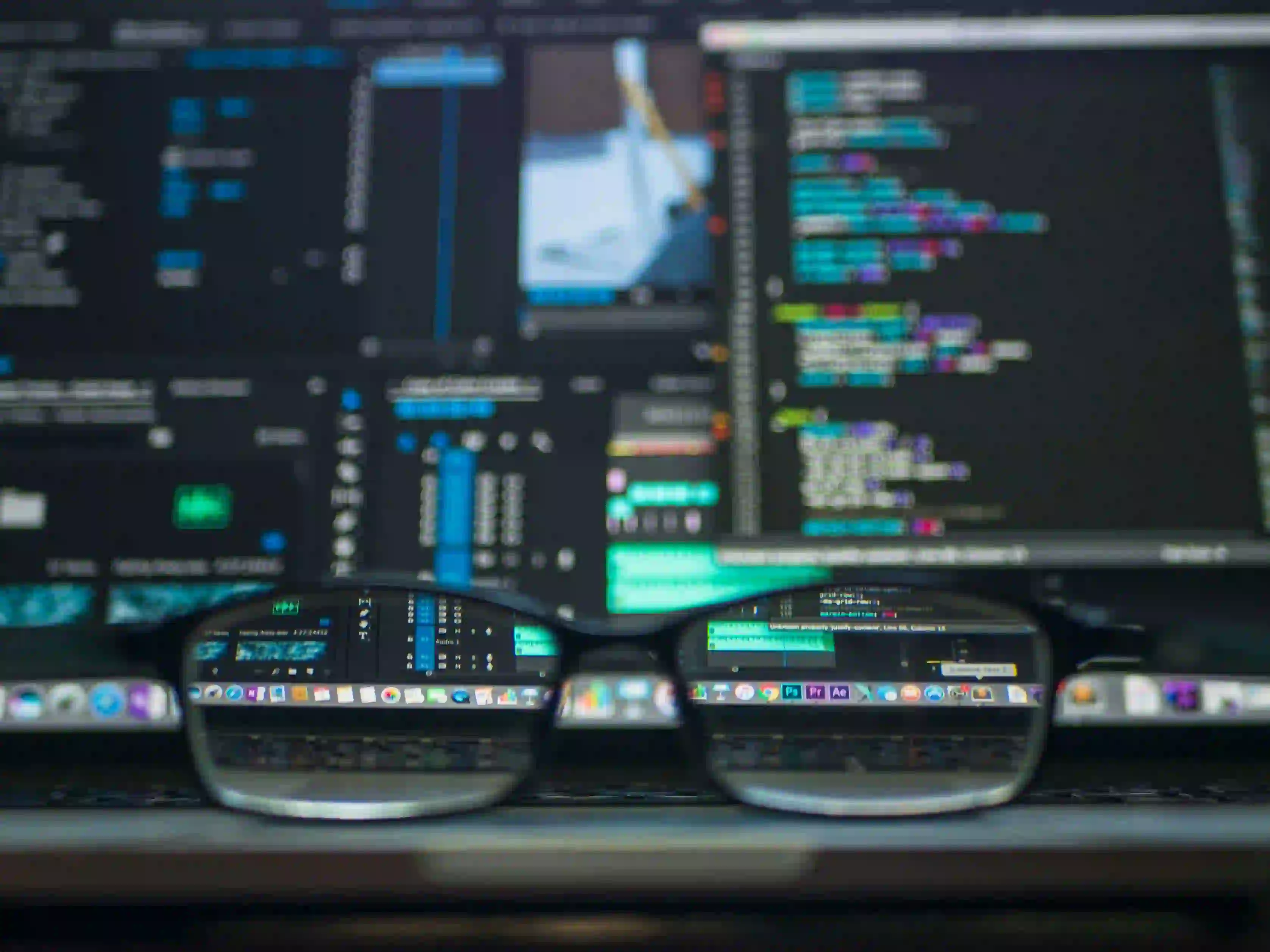
Demystifying Java 8 Default Method Conflict
In the world of Java development, one of the most remarkable features introduced in Java 8 was the addition of default methods in interfaces. This was a significant shift, allowing interfaces to provide method implementations. However, with this power came the potential for ambiguity - the scenario where a class implements multiple interfaces, and those interfaces have default methods with the same signature.
The Scenario
Consider a scenario where a class Car
implements two interfaces, Convertible
and Luxury
, and both of these interfaces provide a default method openRoof()
.
interface Convertible {
default void openRoof() {
System.out.println("Opening the convertible roof");
}
}
interface Luxury {
default void openRoof() {
System.out.println("Opening the luxury roof");
}
}
class Car implements Convertible, Luxury {
// code for Car class
}
The Conflict
In this scenario, when calling openRoof()
on an instance of Car
, which method should be invoked - openRoof()
defined in Convertible
or Luxury
? This dilemma represents a conflict known as the "diamond problem," a form of multiple inheritance ambiguity.
Resolving the Conflict
Java 8 provides a straightforward resolution by requiring the class implementing these interfaces to provide its own implementation for the conflicting default methods. This forces us to explicitly resolve the ambiguity.
class Car implements Convertible, Luxury {
@Override
public void openRoof() {
Convertible.super.openRoof(); // Resolving the conflict by explicitly calling the interface methods
}
}
By doing this, the ambiguity is resolved, and the compiler can determine which method implementation to use based on the class's explicit choice.
The 'Super' Keyword in Resolving Default Method Conflicts
Notice the usage of Convertible.super.openRoof()
in the overridden openRoof()
method. This is the "super" construct for referring to specific interface default methods.
By using InterfaceName.super
, we explicitly specify which interface's default method implementation should be used. This provides clarity and maintains the benefits of default methods while avoiding ambiguity.
The Significance
Understanding and resolving default method conflicts is crucial for maintaining code clarity and ensuring seamless integration of new features. The ability to leverage default methods in interfaces is a powerful tool for evolving codebases without breaking existing implementations.
By comprehending the resolution strategies, developers can harness the full potential of default methods while mitigating any potential conflicts that may arise in complex class hierarchies and interface implementations.
Closing Remarks
In essence, default method conflicts in Java 8 interfaces, though potentially puzzling, can be effectively managed through explicit resolution in implementing classes. The InterfaceName.super
construct provides a precise mechanism for disambiguation, thereby maintaining the simplicity and clarity of Java code.
To delve deeper into this topic, explore the official Java documentation on default methods, and consider practical examples to reinforce understanding and proficiency in handling default method conflicts.