Maximizing Performance with Java 11's New HTTP Client
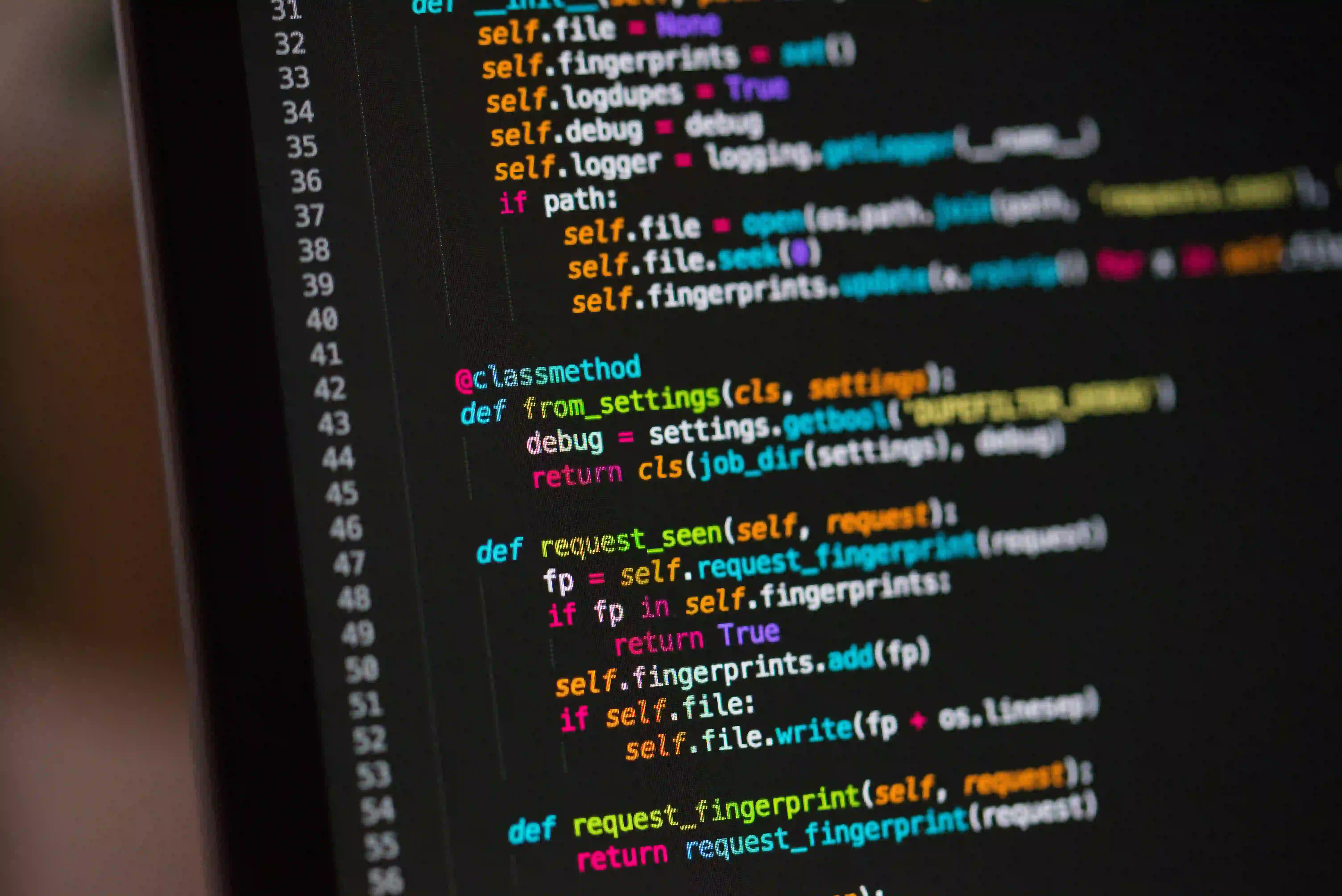
Maximizing Performance with Java 11's New HTTP Client
In the world of web development, optimizing performance is crucial. With the release of Java 11, developers were introduced to a new HTTP client that promises improved performance and increased flexibility. In this blog post, we'll explore the capabilities of Java 11's new HTTP client and how it can be utilized to maximize performance in your Java applications.
Understanding the Need for a New HTTP Client
The legacy HttpURLConnection
API, while functional, had its limitations. It was verbose, lacked flexibility, and did not fully utilize modern best practices in HTTP client design. In contrast, Java 11's HttpClient
provides a more modern and efficient approach to handling HTTP requests and responses.
Key Features of Java 11's HttpClient
The HttpClient
in Java 11 offers several key features that make it an attractive option for optimizing performance:
-
Asynchronous API: The new HTTP client provides an asynchronous API, allowing for non-blocking HTTP requests. This is essential for applications requiring high concurrency.
-
WebSocket Support: It includes built-in support for WebSocket, allowing seamless integration with WebSocket-based communication.
-
HTTP/2 Support: Java 11's
HttpClient
natively supports HTTP/2, providing improved performance and efficiency over its predecessor, HTTP/1.1. -
Simplified API: The API is more concise and intuitive, making it easier to use and reducing boilerplate code.
Now, let's dive into some code examples to demonstrate how these features can be leveraged to maximize performance.
Making Asynchronous Requests
One of the most significant advantages of the new HttpClient
is its support for making asynchronous requests. This feature enables the application to continue performing other tasks while waiting for the HTTP response, leading to improved overall performance and responsiveness.
import java.net.URI;
import java.net.http.HttpClient;
import java.net.http.HttpRequest;
import java.net.http.HttpResponse;
import java.util.concurrent.CompletableFuture;
public class AsyncHttpRequest {
public static void main(String[] args) {
HttpClient httpClient = HttpClient.newHttpClient();
HttpRequest request = HttpRequest.newBuilder()
.uri(URI.create("https://api.example.com/data"))
.build();
CompletableFuture<HttpResponse<String>> responseFuture = httpClient
.sendAsync(request, HttpResponse.BodyHandlers.ofString());
responseFuture.thenAccept(response -> {
System.out.println("Response status code: " + response.statusCode());
System.out.println("Response body: " + response.body());
});
// Other tasks can be performed while waiting for the response
}
}
In this example, the sendAsync
method is used to initiate the asynchronous HTTP request, and the thenAccept
method is used to handle the response when it becomes available.
Leveraging HTTP/2 Support
HTTP/2 is a major revision of the HTTP protocol that offers significant performance improvements over HTTP/1.1, particularly in the context of web applications with high load and throughput requirements. Java 11's HttpClient
provides native support for HTTP/2, allowing developers to take advantage of these performance benefits.
import java.net.URI;
import java.net.http.HttpClient;
import java.net.http.HttpRequest;
import java.net.http.HttpResponse;
public class Http2Request {
public static void main(String[] args) throws Exception {
HttpClient httpClient = HttpClient.newBuilder()
.version(HttpClient.Version.HTTP_2)
.build();
HttpRequest request = HttpRequest.newBuilder()
.uri(URI.create("https://api.example.com/data"))
.build();
HttpResponse<String> response = httpClient.send(request, HttpResponse.BodyHandlers.ofString());
System.out.println("Response status code: " + response.statusCode());
System.out.println("Response body: " + response.body());
}
}
In this example, we explicitly set the HTTP version to HTTP_2
when building the HttpClient
, ensuring that the client utilizes the HTTP/2 protocol for improved performance.
Supporting WebSocket Communication
WebSocket is a communication protocol that provides full-duplex communication channels over a single TCP connection, making it suitable for applications requiring real-time data exchange. With Java 11's HttpClient
, integrating WebSocket communication is straightforward.
import java.net.URI;
import java.net.http.HttpClient;
import java.net.http.WebSocket;
import java.util.concurrent.CompletionStage;
import java.util.concurrent.CountDownLatch;
import java.util.concurrent.TimeUnit;
public class WebSocketClient {
public static void main(String[] args) throws Exception {
CountDownLatch latch = new CountDownLatch(1);
HttpClient httpClient = HttpClient.newHttpClient();
WebSocket webSocket = httpClient.newWebSocketBuilder()
.buildAsync(URI.create("wss://example.com/socket"), new WebSocket.Listener() {
@Override
public CompletionStage<?> onText(WebSocket webSocket, CharSequence data, boolean last) {
System.out.println("Received message: " + data);
latch.countDown();
return null;
}
}).join();
// Application logic and other tasks can be performed while the WebSocket connection is open
latch.await(10, TimeUnit.SECONDS);
webSocket.sendText("Hello, WebSocket!", true);
}
}
In this example, we create a WebSocket client using the newWebSocketBuilder
method and specify the WebSocket URI. We also provide a WebSocket.Listener
to handle incoming messages. This allows for efficient real-time communication, thereby maximizing performance in WebSocket-based applications.
Key Takeaways
Java 11's new HTTP client introduces a modern and efficient approach to handling HTTP requests and responses. By leveraging its asynchronous capabilities, HTTP/2 support, and WebSocket integration, developers can maximize the performance of their Java applications. It's clear that the new HttpClient
is a valuable addition to the Java ecosystem, offering a range of features that enable developers to build high-performance, modern web applications.
To learn more about Java 11's HttpClient
and its capabilities, refer to the official Java 11 Documentation.
Optimize your Java applications' performance with Java 11's new HTTP client today!