Building a Scalable AI for Connect Four in JavaFX
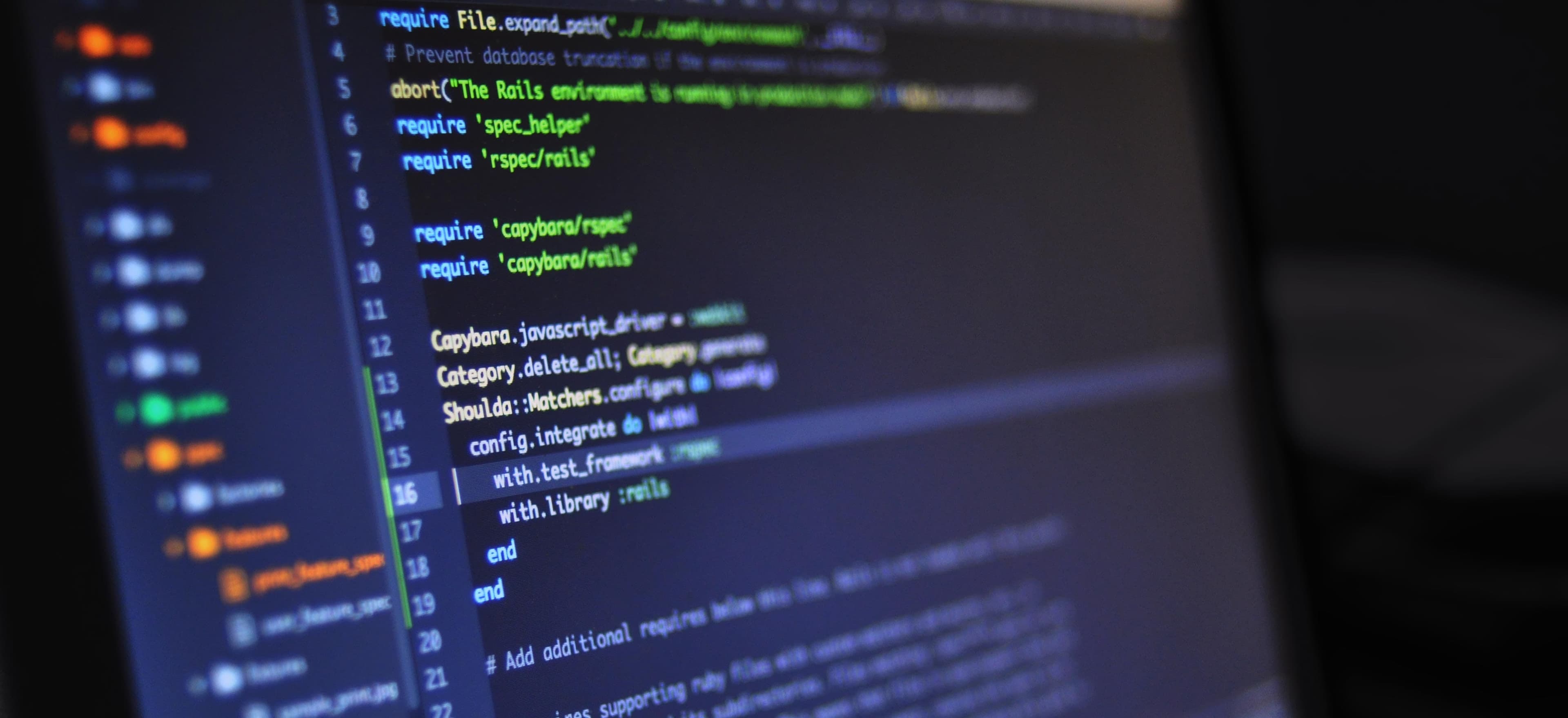
- Published on
Building a Scalable AI for Connect Four in JavaFX
In this blog post, we will explore the process of building a scalable AI for the game of Connect Four using JavaFX. Connect Four is a classic two-player game in which the players take turns dropping colored discs into a grid. The first player to connect four of their discs in a row, either horizontally, vertically, or diagonally, wins the game.
The Game Logic
Before diving into the AI implementation, let's first establish the game logic for Connect Four. The game is played on a 6x7 grid, and the players take turns dropping their colored discs into the columns. The discs fall to the lowest available position within the chosen column. The key to implementing a scalable AI lies in efficiently evaluating potential moves and selecting the best one.
In Java, we can represent the game board using a 2D array, where each cell corresponds to a position on the grid. We can use integer constants to represent the players' discs, for example, PLAYER_ONE = 1
and PLAYER_TWO = 2
.
public class ConnectFourGame {
private static final int ROWS = 6;
private static final int COLUMNS = 7;
private int[][] board;
public ConnectFourGame() {
board = new int[ROWS][COLUMNS];
// Initialize the board with empty cells
}
// Other game logic methods such as dropDisc() and checkForWin()
}
Minimax Algorithm
The AI for Connect Four can be implemented using the Minimax algorithm, which is a decision-making algorithm used in two-player games. It evaluates all possible game states resulting from different moves and selects the best move for the AI player. However, the naïve implementation of Minimax can be computationally expensive, especially for a game like Connect Four with a large branching factor.
Alpha-Beta Pruning
To address the computational complexity of Minimax, we can incorporate the Alpha-Beta Pruning technique. Alpha-Beta Pruning is a significant optimization over the Minimax algorithm, as it eliminates the need to evaluate all the possible moves at every game state, resulting in a dramatic reduction in the number of nodes that need to be evaluated.
Let's implement the Minimax algorithm with Alpha-Beta Pruning for our Connect Four AI. We'll start by creating a method to calculate the best move for the AI player.
public class ConnectFourAI {
public int getBestMove(ConnectFourGame game, int depth, int player) {
// Add Minimax with Alpha-Beta Pruning implementation here
}
// Other AI-related methods
}
Evaluating Game States
In the context of Connect Four, evaluating the game states involves assessing the desirability of a given game position for the AI player. We can assign scores to game positions based on certain criteria. For example, a position that allows the AI player to create a potential winning sequence should be assigned a high score, while a position that sets up the opponent for a win should be assigned a low score.
private int evaluatePosition(ConnectFourGame game, int player) {
// Evaluate the position and return a score
}
Scalability
The scalability of the AI can be achieved by controlling the depth to which the Minimax algorithm explores the game tree. A shallow search depth will result in quick but less optimal moves, while a deeper search depth will lead to more optimal but time-consuming moves. Balancing between the search depth and computational resources is crucial for building a scalable AI.
public int getBestMove(ConnectFourGame game, int depth, int player) {
if (depth == 0 || game.isGameOver()) {
return evaluatePosition(game, player);
}
// Implement the rest of the Minimax algorithm here
}
User Interface with JavaFX
To complete the Connect Four game with AI, we can create a user interface using JavaFX. This will allow users to interact with the game and play against the AI. The user interface should include a grid for the game board, interactive disc dropping, and game state display.
public class ConnectFourUI extends Application {
@Override
public void start(Stage primaryStage) {
// Implement the JavaFX user interface for Connect Four game
}
// Other JavaFX-related methods
}
Lessons Learned
In conclusion, we have explored the process of building a scalable AI for Connect Four using JavaFX. By implementing the Minimax algorithm with Alpha-Beta Pruning and evaluating game states based on certain criteria, we can create an AI player capable of making intelligent moves in the game. Integrating the AI with a JavaFX user interface enables an engaging gaming experience for the users.
Building a scalable AI for Connect Four not only showcases the power of algorithms and data structures but also illustrates the seamless integration of AI with user interfaces in Java applications.
For further exploration, you can delve into JavaFX documentation for advanced user interface techniques and algorithm optimization for improving the performance of the AI.
Happy coding!