Real-Time User Tracking with Twitter4j and Esper
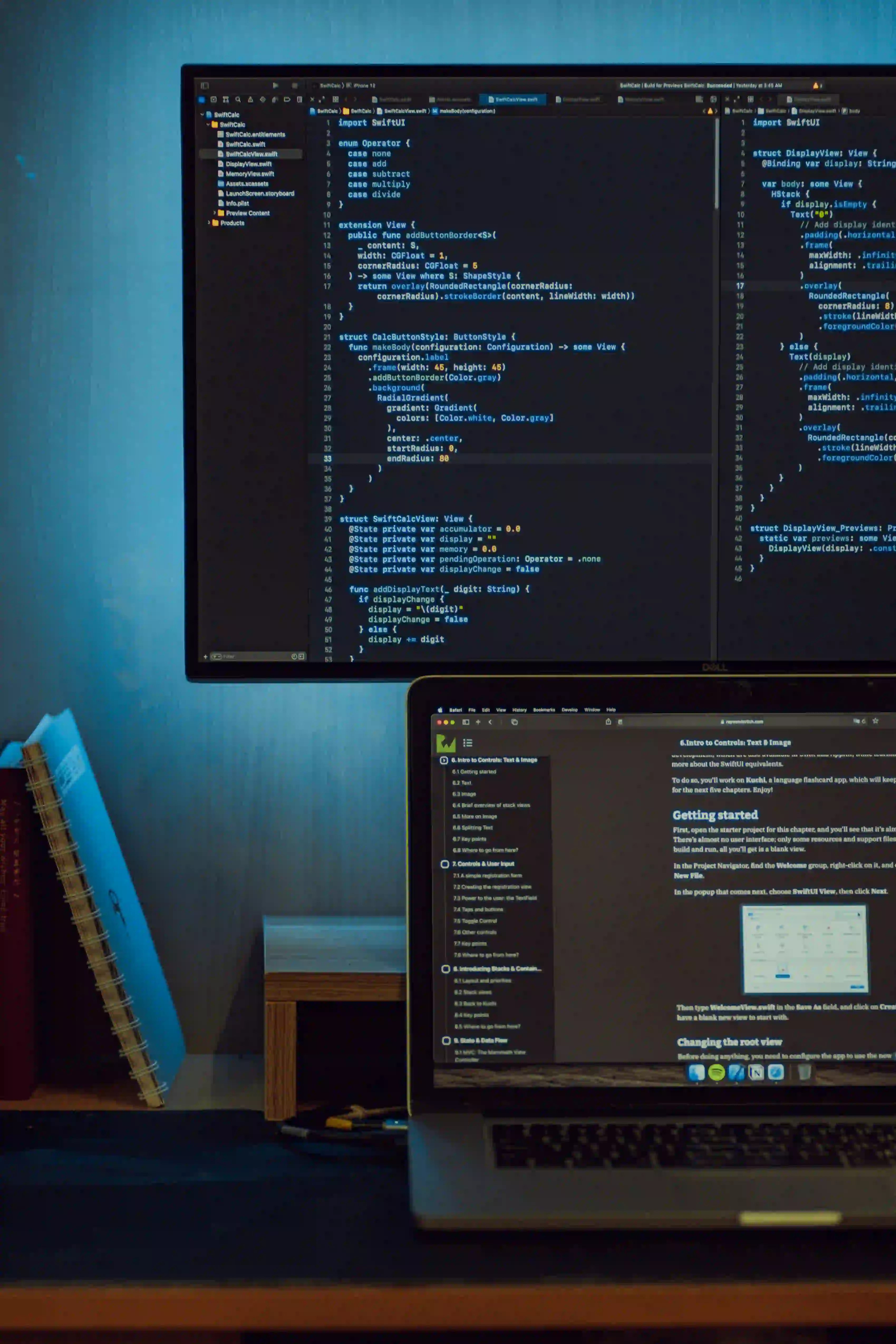
Real-Time User Tracking with Twitter4j and Esper
In today's digital age, real-time user tracking has become a crucial aspect of many applications. Whether it's for monitoring user engagement, analyzing customer behavior, or tracking social media activity, the ability to capture and process data in real-time has substantial implications. In this blog post, we will explore how to implement real-time user tracking using Twitter4j, a Java library for the Twitter API, in conjunction with Esper, a powerful event processing and streaming SQL engine.
What is Twitter4j?
Twitter4j is a Java library for the Twitter API. It provides a convenient way to interact with Twitter's RESTful API and seamlessly integrates Twitter functionality into Java applications. With Twitter4j, developers can access Twitter's features, such as retrieving tweets, posting tweets, streaming real-time tweets, and accessing user information.
What is Esper?
Esper is an open-source, complex event processing (CEP) engine for Java. It enables developers to process and analyze incoming data streams in real-time and derive actionable insights from the data. Esper employs an SQL-like syntax for querying and analyzing event streams, making it a powerful tool for real-time data processing and analysis.
Setting Up the Project
To begin with real-time user tracking using Twitter4j and Esper, we need to set up a new Java project. We can use Maven or Gradle for managing dependencies, but for this example, let's use Maven.
Add the following dependency for Twitter4j in your Maven pom.xml
:
<dependency>
<groupId>org.twitter4j</groupId>
<artifactId>twitter4j-core</artifactId>
<version>4.0.7</version>
</dependency>
Next, add the Esper dependency:
<dependency>
<groupId>com.espertech</groupId>
<artifactId>esper</artifactId>
<version>8.8.0</version>
</dependency>
After adding the dependencies, your project is ready to start integrating real-time user tracking with Twitter4j and Esper.
Implementing Real-Time User Tracking
Initializing Twitter4j
The first step is to initialize Twitter4j and set up a stream to track user activities. Twitter's Streaming API allows us to receive real-time data, which is ideal for user tracking. Here's a basic example of setting up the Twitter stream using Twitter4j:
import twitter4j.*;
import twitter4j.conf.*;
public class TwitterStreamExample {
public static void main(String[] args) {
ConfigurationBuilder cb = new ConfigurationBuilder();
cb.setDebugEnabled(true)
.setOAuthConsumerKey("yourConsumerKey")
.setOAuthConsumerSecret("yourConsumerSecret")
.setOAuthAccessToken("yourAccessToken")
.setOAuthAccessTokenSecret("yourAccessTokenSecret");
TwitterStream twitterStream = new TwitterStreamFactory(cb.build()).getInstance();
// Set up a listener to receive user activities
twitterStream.addListener(new UserActivityListener());
twitterStream.user();
}
}
In this example, we initialize the Twitter stream with the requisite authentication keys. We then set up a listener (UserActivityListener
) to handle user activities, which we'll define shortly.
Creating the UserActivityListener
The UserActivityListener
class implements UserStreamListener
to handle various user activities such as tweets, retweets, mentions, and follows. Here's a simplified version of the listener:
import twitter4j.*;
public class UserActivityListener implements UserStreamListener {
@Override
public void onStatus(Status status) {
// Handle incoming tweets
String user = status.getUser().getScreenName();
String tweet = status.getText();
// Process and analyze the tweet
// ...
}
@Override
public void onDeletionNotice(StatusDeletionNotice statusDeletionNotice) {
// Handle deleted tweets
}
// Implement other listener methods for retweets, mentions, and follows
// ...
}
In the onStatus
method, we extract the user's screen name and the tweet text, providing an opportunity for further processing and analysis.
Integrating With Esper
Once we've obtained user activities from Twitter4j, we can integrate Esper to process and analyze these activities in real-time. Esper allows us to define event patterns and apply continuous queries to the incoming data stream. Here's how we can define an Esper statement to track the frequency of tweets by users in a specific time window:
import com.espertech.esper.client.*;
public class EsperUserActivityTracker {
public static void main(String[] args) {
Configuration config = new Configuration();
EPServiceProvider epService = EPServiceProviderManager.getDefaultProvider(config);
EPAdministrator admin = epService.getEPAdministrator();
String eplStatement = "select user, count(*) as tweetCount " +
"from UserActivityEvent.win:time(1 hour) " +
"where activityType = 'TWEET' " +
"group by user";
EPStatement statement = admin.createEPL(eplStatement);
statement.addListener((newData, oldData) -> {
// Handle the user activity data, e.g., update a dashboard or database
});
}
}
In this example, we define an EPL (Esper Processing Language) statement to calculate the tweet count for each user in a one-hour window. The UserActivityEvent
represents the event type received from Twitter4j, and we extract relevant information using the select
and group by
clauses. The addListener
method allows us to define the action to be taken when new data matching the statement is received.
Bringing It All Together
Real-time user tracking is a powerful feature that can provide valuable insights into user behavior, social media impact, and audience engagement. By combining Twitter4j for accessing Twitter's real-time user activities and Esper for real-time event processing, Java developers can build sophisticated applications for tracking and analyzing user interactions.
In this blog post, we explored the integration of Twitter4j and Esper to achieve real-time user tracking in Java. We covered setting up the Twitter stream, defining a custom listener for user activities, and integrating Esper to process and analyze the incoming user activity events. The possibilities of real-time user tracking extend beyond tweet counts, enabling developers to explore trends, user interactions, and campaign impact.
By utilizing the capabilities of Twitter4j and Esper, developers can create compelling applications that harness the power of real-time user tracking to deliver actionable insights and enhance user engagement.
Start implementing real-time user tracking today and unlock the potential of real-time user data in your Java applications!