Maximizing Performance with Oracle GlassFish
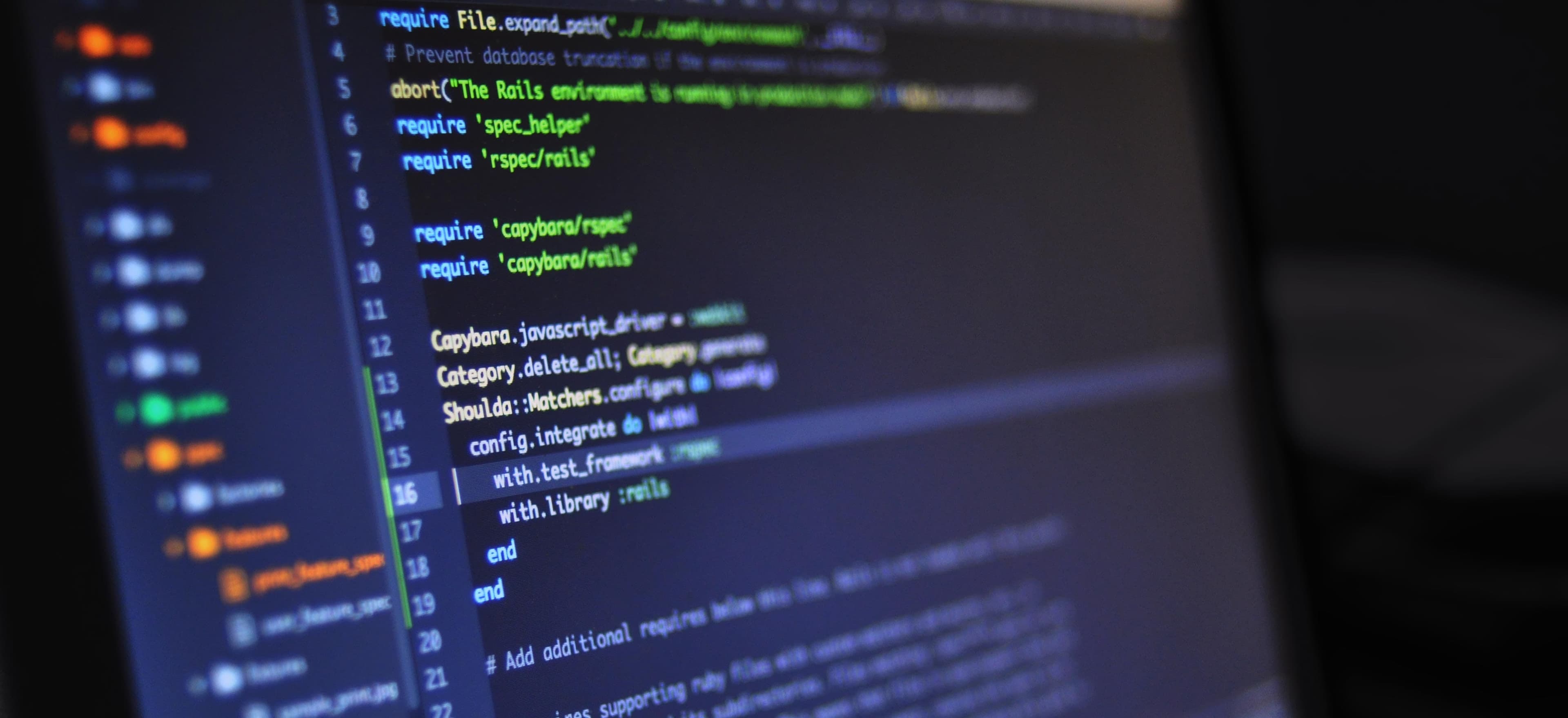
- Published on
Maximizing Performance with Oracle GlassFish
Oracle GlassFish is a robust, open-source application server that provides a powerful platform for developing and deploying Java EE applications. Maximize your application's performance by leveraging the advanced features and optimization techniques offered by Oracle GlassFish. In this article, we will explore best practices and strategies to enhance the performance of Java applications running on GlassFish.
Understanding Performance Optimization
Performance optimization is crucial for ensuring that your Java applications run efficiently and deliver responsive user experiences. By fine-tuning various aspects of your application and the underlying server infrastructure, you can achieve significant improvements in throughput, scalability, and response times. Oracle GlassFish offers a range of features and configuration options that can be leveraged to optimize the performance of your applications.
Effective Resource Management
Efficient resource management is essential for optimizing application performance. Oracle GlassFish provides a comprehensive set of tools for managing resources such as connection pools, thread pools, and memory allocation. By properly configuring these resources, you can ensure optimal utilization and prevent bottlenecks that could hinder performance.
Connection Pool Optimization
@Resource(name = "jdbc/MyDataSource")
DataSource ds;
When working with databases, connection pooling is a critical aspect of performance optimization. By configuring connection pools in GlassFish, you can efficiently manage database connections, minimize overhead, and improve the responsiveness of your application. Properly configured connection pooling can help prevent connection leaks and enhance the overall throughput of database operations.
Thread Pool Management
@ManagedBean
public class MyBean {
@Resource(name = "concurrent/__defaultManagedThreadFactory")
ManagedThreadFactory threadFactory;
}
GlassFish allows you to configure thread pools to manage concurrent requests effectively. By adjusting the size and configuration of thread pools, you can prevent thread contention and ensure that your application can handle a high volume of concurrent requests without compromising performance.
Memory Allocation Tuning
<jvm-options>-Xms1024m</jvm-options>
<jvm-options>-Xmx2048m</jvm-options>
Optimizing memory allocation is crucial for Java application performance. Properly configuring heap size and garbage collection settings in GlassFish can prevent memory-related performance issues and support the efficient management of Java objects.
Leveraging Caching Mechanisms
Caching is a powerful technique for improving application performance by reducing the need to regenerate data or perform expensive computations. Oracle GlassFish provides built-in support for caching, allowing you to cache objects, database queries, and web resources to enhance the responsiveness of your application.
Application-Level Caching
@Cacheable
public String getCustomerDetails(int customerId) {
// Fetch and return customer details
}
By leveraging application-level caching annotations in GlassFish, you can cache the results of expensive method calls, reducing the computational overhead and improving response times for frequently accessed data.
Web Resource Caching
<cacheControl>
<cache-directive>max-age=3600</cache-directive>
</cacheControl>
GlassFish enables you to configure caching directives for web resources, such as static files and REST API responses. By specifying cache-control directives, you can instruct clients to cache resources locally, reducing the need for frequent server requests and enhancing overall performance.
Performance Monitoring and Profiling
Effective performance optimization requires continuous monitoring and profiling of your applications to identify bottlenecks and areas for improvement. GlassFish provides robust monitoring and profiling capabilities that enable you to gather insights into the runtime behavior of your applications and the underlying server infrastructure.
Monitoring with JMX
GlassFish exposes a wide range of performance metrics through Java Management Extensions (JMX), allowing you to monitor aspects such as thread utilization, memory consumption, and database connection pool statistics. By leveraging JMX, you can gain visibility into the runtime behavior of your applications and take proactive steps to address performance issues.
Profiling with VisualVM
VisualVM is a powerful Java profiling tool that seamlessly integrates with GlassFish, providing in-depth insights into CPU usage, memory allocation, and thread activity. By profiling your applications with VisualVM, you can identify performance hotspots and optimize critical areas of your code to enhance overall application performance.
Utilizing Asynchronous Processing
@Asynchronous
public Future<Void> processOrder(Order order) {
// Asynchronous processing logic
}
Asynchronous processing is a key strategy for improving the responsiveness and scalability of Java applications. GlassFish provides support for asynchronous EJBs and methods, allowing you to offload time-consuming tasks to background processes and free up valuable server resources to handle additional requests, ultimately enhancing overall application performance.
Closing Remarks
Optimizing the performance of Java applications running on Oracle GlassFish is essential for delivering a seamless user experience and maximizing the scalability of your applications. By effectively managing resources, leveraging caching mechanisms, monitoring application performance, and utilizing asynchronous processing, you can unlock the full potential of GlassFish and ensure that your applications perform at their best.
Maximize the performance of your Java applications with Oracle GlassFish and unleash the full power of your enterprise solutions.
Join the vibrant community of Java developers and explore the endless possibilities with Oracle GlassFish today!
Learn more about Oracle GlassFish and discover how it can propel your Java applications to new heights.