Addressing Code Smells for Better Software Quality
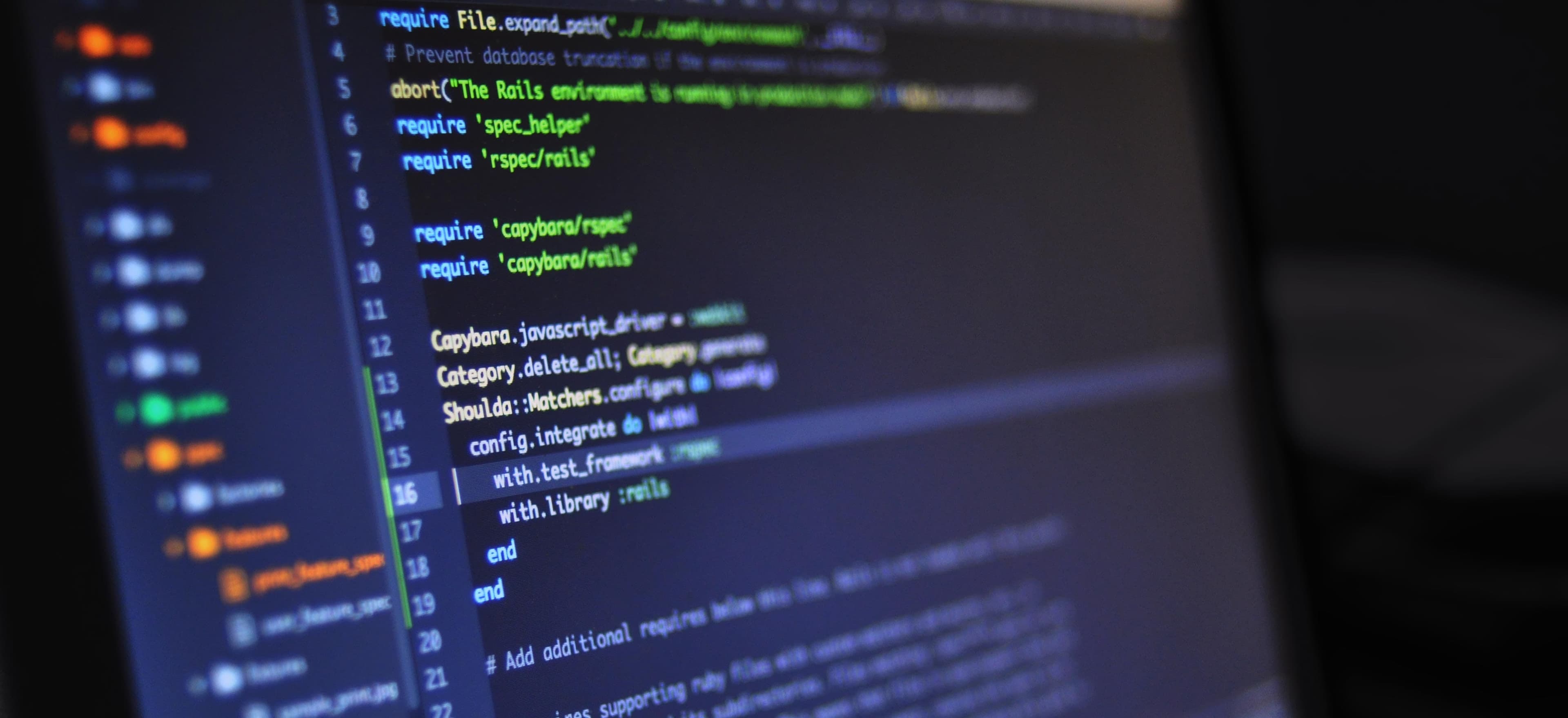
- Published on
Addressing Code Smells for Better Software Quality
Good code is the foundation of any successful software project. It not only ensures the smooth functioning of the application but also makes it easier to maintain, scale, and extend. However, as software projects grow, it's common to encounter "code smells" - symptoms of poor design or implementation that can lead to bugs, inefficiencies, and difficulties in understanding and modifying the code. In this blog, we'll explore what code smells are, why they matter, and how to address them in Java to ensure better software quality.
What are Code Smells?
Code smells are certain structures in the code that may indicate a deeper problem. They are not bugs or errors, but rather indicators of design issues that can lead to problems in the future. For example, long methods, deeply nested conditional statements, and excessive commented-out code are all considered code smells.
Why Do Code Smells Matter?
Addressing code smells is crucial for several reasons. First, code smells can make the codebase harder to understand, leading to more bugs and maintenance issues. They can also slow down development and make it harder to add new features or modify existing ones. Additionally, code smells can be a sign of technical debt, which, if left unaddressed, can accumulate and become increasingly difficult and costly to fix.
Identifying and Addressing Code Smells
Long Methods
Let's start by examining long methods, one of the most common code smells. Long methods are harder to understand, test, and maintain. They often indicate that a method is doing too much and violating the Single Responsibility Principle. Here's an example of a long method:
public void processOrderAndSendEmail(Order order) {
// 50 lines of code...
}
To address this code smell, we can refactor the method into smaller, more focused methods, each responsible for a specific task. This not only improves readability but also makes the code easier to test and maintain.
Nested Conditional Statements
Deeply nested conditional statements make code harder to follow and increase the risk of introducing bugs. Consider the following example:
if (condition1) {
// 20 lines of code...
if (condition2) {
// 30 lines of code...
if (condition3) {
// 40 lines of code...
}
}
}
To address this code smell, we can use techniques like early returns, switch statements, or refactoring the logic into separate methods based on the conditions. This improves readability and reduces the complexity of the code.
Commented-Out Code
Commented-out code is a clear indicator of unused or obsolete code that adds clutter and confusion to the codebase. Consider the following example:
// My old implementation
// public void oldMethod() {
// // ...
// }
To address this code smell, we should simply remove the commented-out code. If the code is no longer needed, keeping it in the codebase only serves to confuse and clutter the code.
Code Duplication
Code duplication is another common code smell that can lead to maintenance nightmares and inconsistencies. It often indicates a lack of proper abstractions and can result in the need to make the same change in multiple places. For example:
public void calculateArea(int length, int width) {
// ...
}
public void calculatePerimeter(int length, int width) {
// ...
}
To address this code smell, we can create a separate method to calculate the common part and then call it from the original methods. This promotes reusability and reduces the chance of inconsistencies.
Tools for Identifying Code Smells
There are several tools available for identifying code smells in Java. SonarQube and Checkstyle are popular choices that can analyze code and report on various code smells. Additionally, modern Integrated Development Environments (IDEs) like IntelliJ IDEA and Eclipse often come with built-in code inspection tools that can help detect and address code smells during development.
Lessons Learned
In conclusion, addressing code smells is essential for maintaining a healthy and maintainable codebase. By identifying and refactoring code smells, we can improve the readability, maintainability, and extensibility of our code, leading to better software quality and overall developer productivity. Remember, good code is not just about functionality; it's also about clarity, maintainability, and scalability.
By following best practices and using the right tools, we can ensure that our Java codebase remains clean and free of code smells, making it easier to work with and less prone to bugs and future technical debt.
So keep an eye out for code smells in your Java projects, and don't hesitate to refactor and improve the code when necessary. Your future self and your fellow developers will thank you for it.
Addressing code smells isn't just about writing cleaner code – it's about ensuring the long-term success of your software projects.
For further reading, if you're interested in diving deeper into code quality and refactoring, check out Martin Fowler's book Refactoring: Improving the Design of Existing Code and Joshua Kerievsky's Refactoring to Patterns. It's always helpful to learn from the best in the industry!