Choosing Between Static Factory Methods and Traditional Constructors
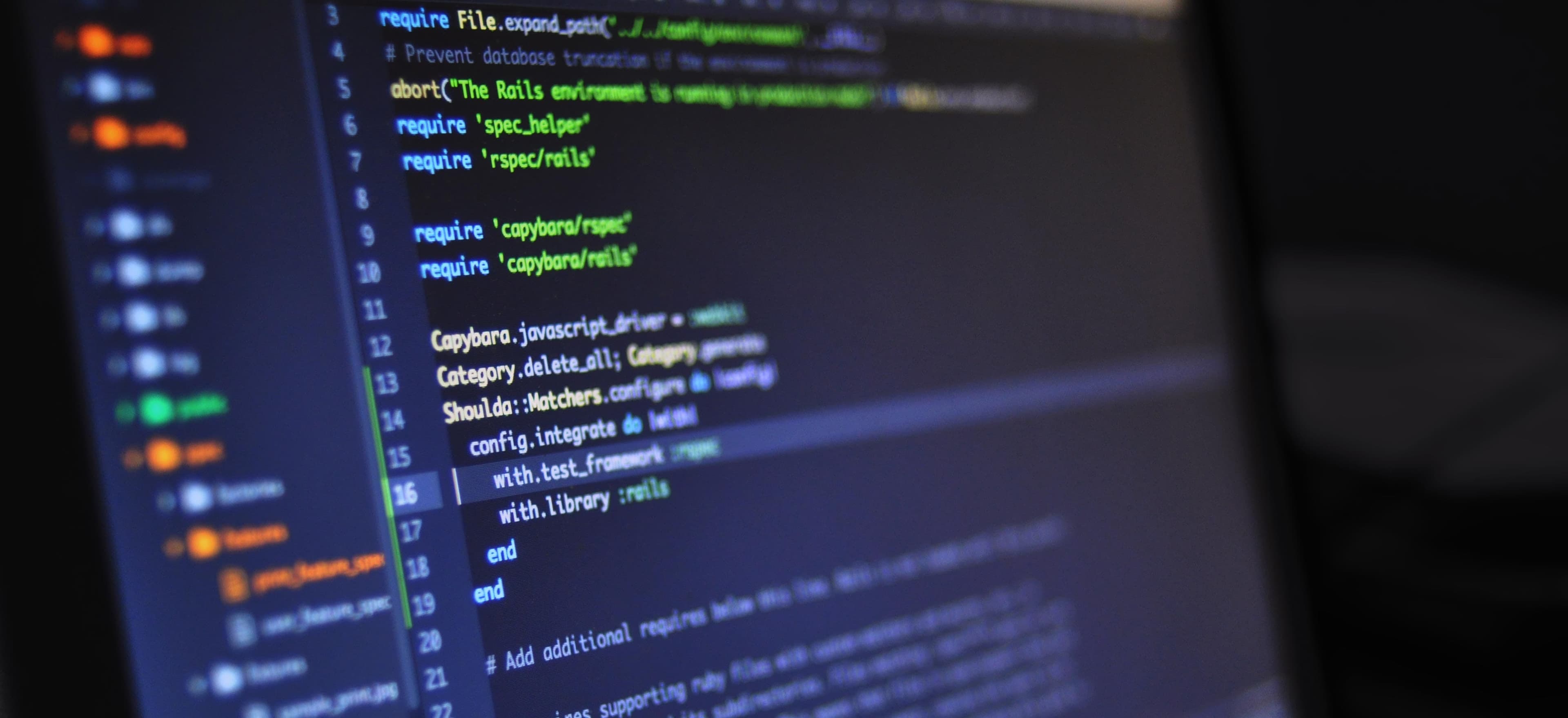
- Published on
A Guide to Choosing Between Static Factory Methods and Traditional Constructors in Java
When it comes to creating instances of a class in Java, developers often have two options: using traditional constructors or static factory methods. Both approaches have their own set of advantages and disadvantages, and choosing between the two can have a significant impact on the design and usability of your codebase. In this guide, we'll explore the characteristics of each approach and discuss scenarios where one might be more suitable than the other.
Traditional Constructors
Traditionally, constructors are used to create new instances of a class. They are defined within the class and have the same name as the class itself. When a constructor is invoked using the new
keyword, a new object is created and initialized based on the parameters passed to the constructor.
public class Car {
private String make;
private String model;
public Car(String make, String model) {
this.make = make;
this.model = model;
}
}
// Creating a new instance using a constructor
Car myCar = new Car("Toyota", "Camry");
Advantages of Constructors:
- Clarity: Constructors make it explicit that a new instance of a class is being created.
- Inheritance: Constructors are inherited by subclasses, allowing for consistent instantiation behavior across class hierarchies.
Disadvantages of Constructors:
- Limited Flexibility: Constructors have limited flexibility in terms of instance creation logic and are unable to return an existing instance under certain circumstances.
Static Factory Methods
Static factory methods, as the name suggests, are static methods within a class that return an instance of the class. Unlike constructors, they have the freedom to return an existing instance or a subclass/subtype of the class. Additionally, static factory methods can have descriptive names, making the code more readable.
public class Car {
private String make;
private String model;
private Car(String make, String model) {
this.make = make;
this.model = model;
}
public static Car createCar(String make, String model) {
return new Car(make, model);
}
}
// Creating a new instance using a static factory method
Car myCar = Car.createCar("Toyota", "Camry");
Advantages of Static Factory Methods:
- Descriptive Naming: Static factory methods can have names that describe the intention of the instance creation, adding to the readability of the code.
- Instance Reuse: They can return an existing instance, implementing a form of object pooling or caching for improved memory efficiency.
- Flexibility: Static factory methods allow for more varied instance creation logic than traditional constructors.
Disadvantages of Static Factory Methods:
- Visibility: They cannot be subclassed or overridden without using complicated workarounds.
- Increased Complexity: Introducing multiple static factory methods can lead to an explosion of method names and potential confusion.
Choosing Between the Two
Use Constructors When:
- Simple Object Creation: For straightforward object instantiation without the need for additional logic or flexibility, traditional constructors are often the simplest approach.
- Inheritance: When working with class hierarchies, constructors facilitate consistent instantiation behavior across subclasses.
Use Static Factory Methods When:
- Caching or Reusing Instances: If there is a need to reuse instances across the application, static factory methods can manage instance reuse more efficiently than constructors.
- Flexible Object Creation: When the logic for instantiating instances is more complex or requires the ability to return a cached instance, static factory methods provide greater flexibility.
Final Thoughts
In conclusion, the choice between traditional constructors and static factory methods in Java depends on the specific requirements of the class being instantiated. While constructors are straightforward and offer inheritance support, static factory methods provide more flexibility and control over instance creation and reuse.
Understanding the trade-offs between the two approaches is crucial for writing maintainable and extensible code. By carefully considering the requirements of your classes and their intended use cases, you can make an informed decision on which instantiation approach best suits your needs.
For further reading on this topic, you may find the following resources helpful:
- Effective Java: Item 1 - Consider static factory methods instead of constructors
- Java static factory methods vs constructors
We hope you found this guide useful for making informed decisions when choosing between traditional constructors and static factory methods in Java. Happy coding!